Python Code: class Node: def __init__(self, initial_data): self.data = initial_data self.next = None def __str__(self): return str(self.data) class LinkedList: def __init__(self): self.head = None self.tail = None def append(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: self.tail.next = new_node self.tail = new_node def Generate(self,num_nodes): # Your code goes here def printList(self): # Your code goes here def swap(self): # Your code goes here if __name__ == '__main__': LL = LinkedList() num_nodes = int(input()) LL.Generate(num_nodes) LL.swap() LL.printList()
Python Code:
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
def __str__(self):
return str(self.data)
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def Generate(self,num_nodes):
# Your code goes here
def printList(self):
# Your code goes here
def swap(self):
# Your code goes here
if __name__ == '__main__':
LL = LinkedList()
num_nodes = int(input())
LL.Generate(num_nodes)
LL.swap()
LL.printList()
*****NOTE*******
Consider the following example to get an idea of how the output of the above program should be
Example:
Input
5 Morning Noon Afternoon Evening Night
Program's Output
Night Noon Afternoon Evening Morning


Step by step
Solved in 3 steps with 1 images

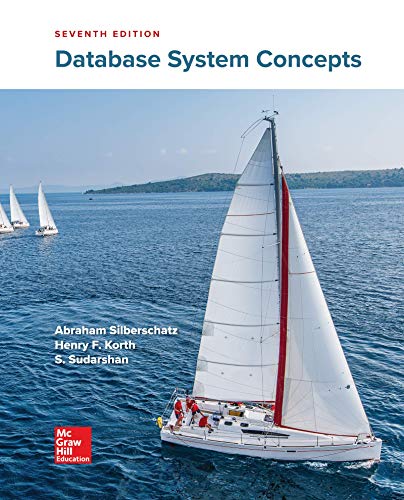
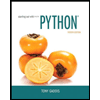
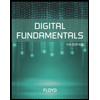
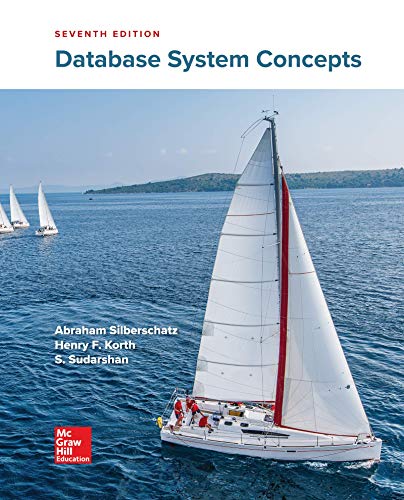
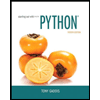
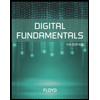
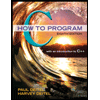
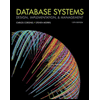
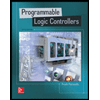