public class FibonacciComparison { // Fibonacci Sequence: 0, 1, 1, 2, 3, 5, 8 . /* input cases 1) 0 2) 3 3) -1 4) 9 output cases
public class FibonacciComparison { // Fibonacci Sequence: 0, 1, 1, 2, 3, 5, 8 . /* input cases 1) 0 2) 3 3) -1 4) 9 output cases
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![import java.lang.System;
3.
public class FibonacciComparison {
4.
// Fibonacci Sequence: 0, 1, 1, 2, 3, 5, 8 ....
/*
7
input cases
8.
1) 0
9
2) 3
10
3) -1
11
4) 9
12
output cases
13
1) 0
14
2) 2
15
3) 0
16
4) 34
17
*/
// Note that you need to return 0 if the input is negative.
// Please pay close attention to the fact that the first index in our fib sequence is 0.
18
19
20
// Recursive Fibonacci
public static int fib(int n) {
// Code this func.
21
22
23
24
return -1;
25
26
// Iterative Fibonacci
27
28
public static int fiblinear(int n) {
// Code this func.
29
30
return -1;
31
32
33
public static void main(String[] args) {
34
// list of fibonacci sequence numbers
int[] nlist
w
{ 5,10, 15, 20, 25, 30, 35, 40, 45};
35
36
37
// Two arrays (one for fibLinear, other for fibRecursive) to store time for each run.
// There are a total of nlist.length inputs that we will test
double[] timingsEF = new double[nlist.Length];
double[] timingsLF = new double[nlist.length];
38
39
40
41
42
// Every number in n_list will be given as input 5 times to both fibonacci functions
43
// and an average will be taken to make the results more accurate.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc7f33394-5a52-4a6c-8272-5682354fd51c%2Fdef39413-be39-47ad-aa6c-9f158a7ce2dc%2F40q92s5_processed.png&w=3840&q=75)
Transcribed Image Text:import java.lang.System;
3.
public class FibonacciComparison {
4.
// Fibonacci Sequence: 0, 1, 1, 2, 3, 5, 8 ....
/*
7
input cases
8.
1) 0
9
2) 3
10
3) -1
11
4) 9
12
output cases
13
1) 0
14
2) 2
15
3) 0
16
4) 34
17
*/
// Note that you need to return 0 if the input is negative.
// Please pay close attention to the fact that the first index in our fib sequence is 0.
18
19
20
// Recursive Fibonacci
public static int fib(int n) {
// Code this func.
21
22
23
24
return -1;
25
26
// Iterative Fibonacci
27
28
public static int fiblinear(int n) {
// Code this func.
29
30
return -1;
31
32
33
public static void main(String[] args) {
34
// list of fibonacci sequence numbers
int[] nlist
w
{ 5,10, 15, 20, 25, 30, 35, 40, 45};
35
36
37
// Two arrays (one for fibLinear, other for fibRecursive) to store time for each run.
// There are a total of nlist.length inputs that we will test
double[] timingsEF = new double[nlist.Length];
double[] timingsLF = new double[nlist.length];
38
39
40
41
42
// Every number in n_list will be given as input 5 times to both fibonacci functions
43
// and an average will be taken to make the results more accurate.
![44
int numTrials = 5;
45
46
//Iterating over number list
for ( int i = 0; i < nlist.length; i++ ) {
47
48
int n =
nlist[i];
49
50
//
FibRecursive
51
// Start recording time
52
<Code here>
53
// Run fibRecursive function 5 times
for ( int k = 0; k < numTrials; k++ )
54
55
fib(n);
// Stop recording time
56
57
<Code here>
wwww
58
// Taking average of the run time and store it in the array
59
timingsEF[i] = le-9*(stop-start) / numTrials;
60
61
//
Fiblinear
62
// Start recording time
63
<Code here>
ww
// Run Fiblinear 5 times
64
65
for ( int k = 0; k < numTrials; k++ )
fiblinear (n);
66
67
68
// Stop recording time
69
<Code here>
70
//Taking average and store it in the array
71
timingsLF[i] = le-9*(stop-start) / numTrials;
72
}
73
74
// Print out the runtimes for different fib functions.
75
System.out.println("Timings for Exponential Fibonacci");
for ( double time : timingsEF )
76
77
System.out.print(" "+time);
78
System.out.println();
79
System.out.println();
80
System.out.println();
81
System.out.println("Timings for Linear Fibonacci");
for ( double time : timingsLF )
82
83
System.out.print(" "+time);
84
System.out.println();
85
86](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc7f33394-5a52-4a6c-8272-5682354fd51c%2Fdef39413-be39-47ad-aa6c-9f158a7ce2dc%2Fwh45us_processed.png&w=3840&q=75)
Transcribed Image Text:44
int numTrials = 5;
45
46
//Iterating over number list
for ( int i = 0; i < nlist.length; i++ ) {
47
48
int n =
nlist[i];
49
50
//
FibRecursive
51
// Start recording time
52
<Code here>
53
// Run fibRecursive function 5 times
for ( int k = 0; k < numTrials; k++ )
54
55
fib(n);
// Stop recording time
56
57
<Code here>
wwww
58
// Taking average of the run time and store it in the array
59
timingsEF[i] = le-9*(stop-start) / numTrials;
60
61
//
Fiblinear
62
// Start recording time
63
<Code here>
ww
// Run Fiblinear 5 times
64
65
for ( int k = 0; k < numTrials; k++ )
fiblinear (n);
66
67
68
// Stop recording time
69
<Code here>
70
//Taking average and store it in the array
71
timingsLF[i] = le-9*(stop-start) / numTrials;
72
}
73
74
// Print out the runtimes for different fib functions.
75
System.out.println("Timings for Exponential Fibonacci");
for ( double time : timingsEF )
76
77
System.out.print(" "+time);
78
System.out.println();
79
System.out.println();
80
System.out.println();
81
System.out.println("Timings for Linear Fibonacci");
for ( double time : timingsLF )
82
83
System.out.print(" "+time);
84
System.out.println();
85
86
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Recommended textbooks for you
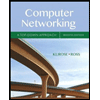
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
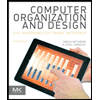
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
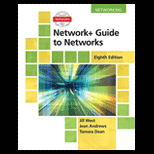
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
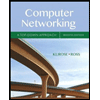
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
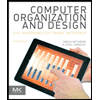
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
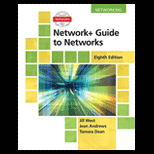
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
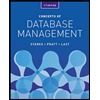
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
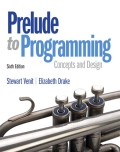
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
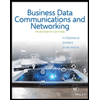
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY