++ PROGRAM: Please complete my program Implement the 4 functions: bool search(int num), bool insert(int num) , bool remove(int num), bool isEmpty bstree.h #include "tree.h" #include using namespace std; class BSTree { BTree* tree; public: BSTree() { tree = new BTree(); } //////////////////////////////////////////////// bool search(int num) { } //////////////////////////////////////////////// bool insert(int num) { // TODO insert } //////////////////////////////////////////////// bool remove(int num) { // TODO remove } /////////////////////////////////////////////// // WARNING. Do not modify this method. void print() { if (isEmpty()) { cout << "EMPTY"; return; } cout << "PRE-ORDER: "; print_preorder(tree->getRoot()); cout << endl << "IN-ORDER: "; print_inorder(tree->getRoot()); cout << endl << "POST-ORDER: "; print_postorder(tree->getRoot()); cout << endl << "STATUS: " << check_health(tree->getRoot(), NULL); } ///////////////////////////////////////////////////// bool isEmpty() { // TODO isEmpty } ///////////////////////////////////////////////////// void print_preorder(node* curr) { // TODO preorder traversal cout << curr->element << " "; if(curr->left != NULL){ print_preorder(curr->left); } if(curr->right != NULL){ print_preorder(curr->right); } } // } ///////////////////////////////////////////////////// // WARNING. Do not modify this method. void print_inorder(node* curr) { if (curr->left != NULL) { print_inorder(curr->left); } cout << curr->element << " "; if (curr->right != NULL) { print_inorder(curr->right); } } //////////////////////////////////////////////////// void print_postorder(node* curr) { // TODO postorder traversal if(curr->left != NULL){ print_postorder(curr->left); } if(curr->right != NULL){ print_postorder(curr->right); } cout << curr->element << " "; } // } //////////////////////////////////////////////////// // WARNING. Do not modify this method. bool check_health(node* curr, node* parent) { return tree->check_health(curr, parent); } };
C++ PROGRAM: Please complete my program
Implement the 4 functions:
bool search(int num), bool insert(int num) , bool remove(int num), bool isEmpty
bstree.h
#include "tree.h"
#include <iostream>
using namespace std;
class BSTree {
BTree* tree;
public:
BSTree() {
tree = new BTree();
}
////////////////////////////////////////////////
bool search(int num) {
}
////////////////////////////////////////////////
bool insert(int num) {
// TODO insert
}
////////////////////////////////////////////////
bool remove(int num) {
// TODO remove
}
///////////////////////////////////////////////
// WARNING. Do not modify this method.
void print() {
if (isEmpty()) {
cout << "EMPTY";
return;
}
cout << "PRE-ORDER: ";
print_preorder(tree->getRoot());
cout << endl << "IN-ORDER: ";
print_inorder(tree->getRoot());
cout << endl << "POST-ORDER: ";
print_postorder(tree->getRoot());
cout << endl << "STATUS: " << check_health(tree->getRoot(), NULL);
}
/////////////////////////////////////////////////////
bool isEmpty() {
// TODO isEmpty
}
/////////////////////////////////////////////////////
void print_preorder(node* curr) {
// TODO preorder traversal
cout << curr->element << " ";
if(curr->left != NULL){
print_preorder(curr->left);
}
if(curr->right != NULL){
print_preorder(curr->right);
}
}
// }
/////////////////////////////////////////////////////
// WARNING. Do not modify this method.
void print_inorder(node* curr) {
if (curr->left != NULL) {
print_inorder(curr->left);
}
cout << curr->element << " ";
if (curr->right != NULL) {
print_inorder(curr->right);
}
}
////////////////////////////////////////////////////
void print_postorder(node* curr) {
// TODO postorder traversal
if(curr->left != NULL){
print_postorder(curr->left);
}
if(curr->right != NULL){
print_postorder(curr->right);
}
cout << curr->element << " ";
}
// }
////////////////////////////////////////////////////
// WARNING. Do not modify this method.
bool check_health(node* curr, node* parent) {
return tree->check_health(curr, parent);
}
};

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

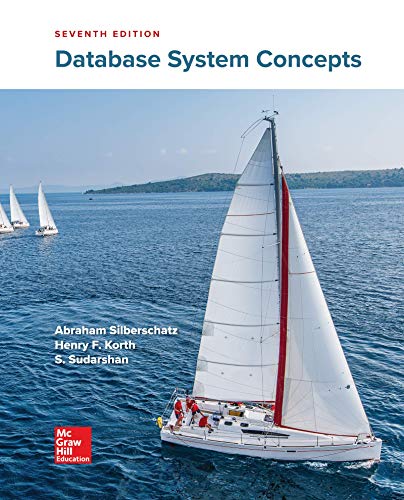
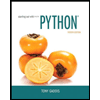
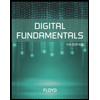
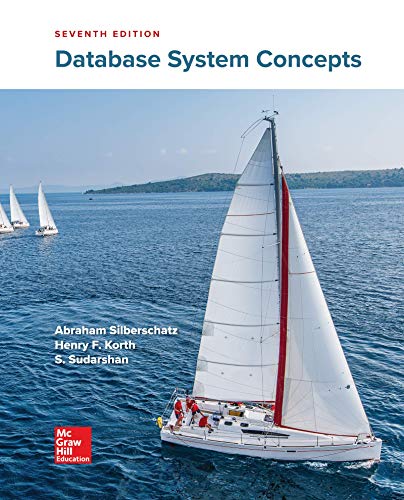
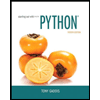
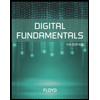
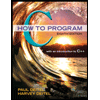
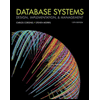
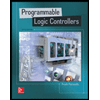