Problem Description: In this assignment, you will need to write a program that will allow the user to play a game. The program will start with showing the user a list of two games ('Guess the Number' and 'Math Challenge') and ask the user which one she/he wants to play. However, to keep the assignment manageable, you are being asked to implement only the first of these two games. If the user selects the second game, a 'technical error' message is shown, and the list of games is again shown to select an option from. If the user selects the first game, "Guess the Number", the program will randomly generate a number between 10 and 30 (inclusive) and allow the user at most 10 (ten) chances to guess it. If the user can correctly guess the number within 10 chances, she/he is awarded one point, otherwise zero point is awarded. After a round is over, the user is asked if she/he wants to play a game again or not. If the user enters a Y', she/he is again shown the list of games to choose from. If the user anything else, she/he is shown the total number of points obtained in this session of play (each round contributes at most one point) and the program exits. Implementation: You need to implement the program using 5 (five) functions: (i) (ii) (iii) (iv) (v) get_validated_input get_game_choice play_math_challenge_game play_guess_the_number_game main Each of these functions is dedicated to performing a single task. (i) gets a number from the user within a specified range, (ii) gets a number from the user indicating which game she/he wants to play, (iii) shows a message that this game is not currently playable, (iv) implements the game 'Guess the Number', and (v) is the function that drives the program by presenting the user game options, collecting the option and calling the appropriate game function, asking the choice to continue playing, showing the total points at the end, bidding goodbye, etc. Sample Outputs: Sample output #1 Available games: 1. Guess the number 2. Math challenge Please enter your choice (1/2): 1 =============== Guess the number =============== I am thinking a number between 10 and 30 You have 10 chances left to guess the number Please enter your guess (10-30): 2 Please enter your guess (10-30): 22 Sorry, you guessed wrong. Try again. You have 9 chances left to guess the number Please enter your guess (10-30): 24 Sorry, you guessed wrong. Try again. You have 8 chances left to guess the number Please enter your guess (10-30): 11 Sorry, you guessed wrong. Try again. You have 7 chances left to guess the number Please enter your guess (10-30): 12 Sorry, you guessed wrong. Try again. You have 6 chances left to guess the number Please enter your guess (10-30): 15 Sorry, you guessed wrong. Try again. You have 5 chances left to guess the number Please enter your guess (10-30): 18 Correct guess! Enter 'Y' if you want to continue. n You are leaving with 1 points ************* 318 ************
I need this done in python. I have provided the code my professor gave my class, and am required to complete steps 4 and 5, as 1, 2, 3 are done for me. Thanks.
import random
def get_validated_input(lo, hi, prompt):
user_input = int(input(prompt))
while not lo <= user_input <=hi:
user_input = int(input(prompt))
return user_input
def get_game_choice():
print()
print('Available games:')
print('1. Guess the number')
print('2. Math challenge')
print()
input_prompt = 'Please enter your choice (1/2):'
choice = get_validated_input(1,2,input_prompt)
return choice
def play_math_challenge_game():
print('We are having technical difficulties with this game. Please check back again later.')
print()
def play_guess_the_number_game():
pass # delete this pass statement (needed for an empty function in the skeleton)
def main():
pass # delete this pass statement (needed for an empty function in the skeleton)
main()



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

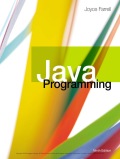
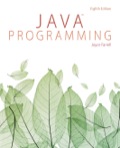
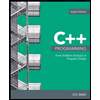
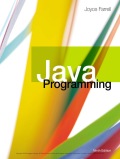
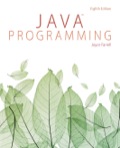
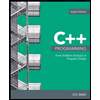
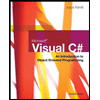