Please help with this modification: 2. Use the logic in that program to design your own Recursive Descent parser in C for the following grammar: S →aAB A →Abc | b B →d 3. Make sure that you choose at least two strings that pass the grammar and one string that does not pass the grammar just like I did in the model program 4. Submit both the code and a screen shot of your results for the choice of strings that you provided. Below is the C code that can be used to implement a Recursive Descent parser for the above grammar /* Recursive Descent Parser for the Expression Grammar: S → (L) |a L' →,SL'|ε L → SL' Valid inputs: (a,(a,a)) and (a,((a,a),(a,a))) Invalid inputs:(aa,a) */ #include #include int S(), Ldash(), L(); char *ip; char string[50]; int main() { printf("Enter the string\n"); scanf("%s", string); ip = string; printf("\n\nInput\t\tAction\n ------------------------------\n"); if (S()) { printf("\n------------------------------------------------\n"); printf("\n String is successfully parsed\n"); } else { printf("\n ------------------------------------------------\n"); printf("Error in parsing string\n"); } } int L() { printf("%s\t\tL →SL' \n", ip); if (S()) { if (Ldash()) { return 1; } else return 0; } else return 0; } int Ldash() { if (*ip == ',') { printf("%s\t\tL' →, SL' \n", ip); ip++; if (S()) { if (Ldash()) { return 1; } else return 0; } else return 0; } else { printf("%s\t\tL' →ε \n", ip); return 1; } } int S() { if (*ip == '(') { printf("%s\t\tS →(L) \n", ip); ip++; if (L()) { if (*ip == ')') { ip++; return 1; } else return 0; } else return 0; } else if (*ip == 'a') { ip++; printf("%s\t\tS →a \n", ip); return 1; } else return 0; }
Please help with this modification: 2. Use the logic in that program to design your own Recursive Descent parser in C for the following grammar: S →aAB A →Abc | b B →d 3. Make sure that you choose at least two strings that pass the grammar and one string that does not pass the grammar just like I did in the model program 4. Submit both the code and a screen shot of your results for the choice of strings that you provided. Below is the C code that can be used to implement a Recursive Descent parser for the above grammar /* Recursive Descent Parser for the Expression Grammar: S → (L) |a L' →,SL'|ε L → SL' Valid inputs: (a,(a,a)) and (a,((a,a),(a,a))) Invalid inputs:(aa,a) */ #include #include int S(), Ldash(), L(); char *ip; char string[50]; int main() { printf("Enter the string\n"); scanf("%s", string); ip = string; printf("\n\nInput\t\tAction\n ------------------------------\n"); if (S()) { printf("\n------------------------------------------------\n"); printf("\n String is successfully parsed\n"); } else { printf("\n ------------------------------------------------\n"); printf("Error in parsing string\n"); } } int L() { printf("%s\t\tL →SL' \n", ip); if (S()) { if (Ldash()) { return 1; } else return 0; } else return 0; } int Ldash() { if (*ip == ',') { printf("%s\t\tL' →, SL' \n", ip); ip++; if (S()) { if (Ldash()) { return 1; } else return 0; } else return 0; } else { printf("%s\t\tL' →ε \n", ip); return 1; } } int S() { if (*ip == '(') { printf("%s\t\tS →(L) \n", ip); ip++; if (L()) { if (*ip == ')') { ip++; return 1; } else return 0; } else return 0; } else if (*ip == 'a') { ip++; printf("%s\t\tS →a \n", ip); return 1; } else return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help with this modification:
2. Use the logic in that program to design your own Recursive Descent parser in C
for the following grammar:
S →aAB
A →Abc | b
B →d
3. Make sure that you choose at least two strings that pass the grammar and one
string that does not pass the grammar just like I did in the model program
4. Submit both the code and a screen shot of your results for the choice of strings
that you provided.
Below is the C code that can be used to implement a Recursive Descent parser for the above
grammar
/* Recursive Descent Parser for the Expression Grammar:
S → (L) |a
L' →,SL'|ε
L → SL'
Valid inputs: (a,(a,a)) and (a,((a,a),(a,a)))
Invalid inputs:(aa,a)
*/
#include <stdio.h>
#include <string.h>
int S(), Ldash(), L();
char *ip;
char string[50];
int main()
grammar
/* Recursive Descent Parser for the Expression Grammar:
S → (L) |a
L' →,SL'|ε
L → SL'
Valid inputs: (a,(a,a)) and (a,((a,a),(a,a)))
Invalid inputs:(aa,a)
*/
#include <stdio.h>
#include <string.h>
int S(), Ldash(), L();
char *ip;
char string[50];
int main()
{
printf("Enter the string\n");
scanf("%s", string);
ip = string;
printf("\n\nInput\t\tAction\n ------------------------------\n");
if (S())
{
printf("\n------------------------------------------------\n");
printf("\n String is successfully parsed\n");
}
else
{
printf("\n ------------------------------------------------\n");
printf("Error in parsing string\n");
}
}
int L()
{
printf("%s\t\tL →SL' \n", ip);
if (S())
{
if (Ldash())
{
return 1;
}
else
return 0;
}
else
return 0;
}
int Ldash()
{
if (*ip == ',')
{
printf("%s\t\tL' →, SL' \n", ip);
ip++;
if (S())
{
if (Ldash())
{
return 1;
}
else
return 0;
}
else
printf("Enter the string\n");
scanf("%s", string);
ip = string;
printf("\n\nInput\t\tAction\n ------------------------------\n");
if (S())
{
printf("\n------------------------------------------------\n");
printf("\n String is successfully parsed\n");
}
else
{
printf("\n ------------------------------------------------\n");
printf("Error in parsing string\n");
}
}
int L()
{
printf("%s\t\tL →SL' \n", ip);
if (S())
{
if (Ldash())
{
return 1;
}
else
return 0;
}
else
return 0;
}
int Ldash()
{
if (*ip == ',')
{
printf("%s\t\tL' →, SL' \n", ip);
ip++;
if (S())
{
if (Ldash())
{
return 1;
}
else
return 0;
}
else
return 0;
}
else
{
printf("%s\t\tL' →ε \n", ip);
return 1;
}
}
int S()
{
if (*ip == '(')
{
printf("%s\t\tS →(L) \n", ip);
ip++;
if (L())
{
if (*ip == ')')
{
ip++;
return 1;
}
else
return 0;
}
else
return 0;
}
else if (*ip == 'a')
{
ip++;
printf("%s\t\tS →a \n", ip);
return 1;
}
else
return 0;
}
}
else
{
printf("%s\t\tL' →ε \n", ip);
return 1;
}
}
int S()
{
if (*ip == '(')
{
printf("%s\t\tS →(L) \n", ip);
ip++;
if (L())
{
if (*ip == ')')
{
ip++;
return 1;
}
else
return 0;
}
else
return 0;
}
else if (*ip == 'a')
{
ip++;
printf("%s\t\tS →a \n", ip);
return 1;
}
else
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
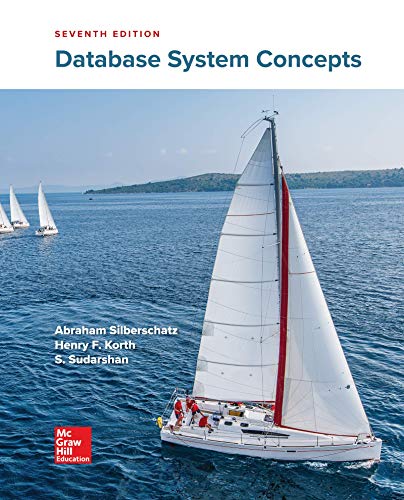
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
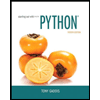
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
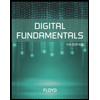
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
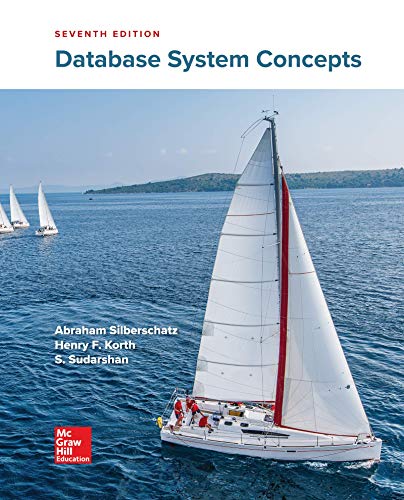
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
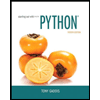
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
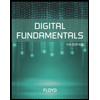
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
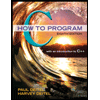
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
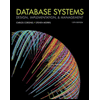
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
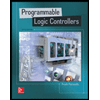
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education