Please complete these files. There are two text files attached. Running the code should result in the creation of an output file ("PE_output.txt"). Please give full reasoning to the answer. Please also paste the full java code and please attach a screenshot of the successful program run. package solution; import java.io.*; import java.util.Scanner; public class PE_1_problem { @SuppressWarnings("resource") public static String readFirstLetters(String filename) { String result = ""; File f = new File(filename); // Surround the next command inside a try/catch block, // as it is possible the file doesn't exist. try { Scanner fileContents = new Scanner(f); while (fileContents.hasNextLine()) { // 1. Get the next line and store in a variable // 2. Check the length of the line. If the line is not an // empty string, add the character at the start of the line // to the result string. // 3. Otherwise, add an empty space to the result string. } fileContents.close(); } catch (FileNotFoundException e) { e.printStackTrace(); } return result; } public static String readNthChars (String filename) { String result = ""; // This is a very similar method, except that instead of // reading the first letter, you will read the nth character, where // 'n' is the same as the line number (using a numbering system // that starts at 0, so the first line has n = 0). Blank lines in // the text file should not be counted when determining line number, // but should still contribute spaces as in the method above. Spaces // within line should also NOT be counted, so you might want to use a // command such as the following in your code: // currentLine = currentLine.replaceAll("\\s", ""); // This command removes all the spaces in the String 'currentLine' // You should also use the command: // currentLine = currentLine.toUpperCase() at some point, so that the // result is formatted all in upper case letters. return result; } public static void main(String[] args) { System.out.println(readFirstLetters("PE_file_1.txt")); System.out.println(readNthChars("PE_file_2.txt")); // Your code here to write the two lines above to an output file ("PE_output.txt"). // Hint: You might want to start with: FileWriter myWriter = new FileWriter("PE_output.txt"); // You should then be able to use myWriter.write("some string here"); // To move to a new line, use: myWriter.write("\n"); // You should check your output file to see that you got the output you wanted. // To find your output file, look in your eclipse workspace. } } PE_file_1.txt Can of Soup - 1 Sweet potatoes - 5 Ice cream - 1 gallon Swiss cheese - 1/2 pound Fish - 1.5 pounds Unsweetened soy milk Nuts, assorted - 1 can PE_file_2.txt Can I write a functional Java program? Is it possible to get a CS internship? Am I a good programmer? Can someone explain what good coding style is? How is data science related to computer science? What attributes should a good programmer have? How can I become a better programmer? What will I do after this class? How do you learn how to debug a program? How much effort does it take to prep for a technical interview? How come I usually can't understand everything in my CS class? What do you consider the best advice for someone studying CS?
Please complete these files. There are two text files attached. Running the code should result in the creation of an output file ("PE_output.txt"). Please give full reasoning to the answer. Please also paste the full java code and please attach a screenshot of the successful program run.
package solution;
import java.io.*;
import java.util.Scanner;
public class PE_1_problem {
@SuppressWarnings("resource")
public static String readFirstLetters(String filename) {
String result = "";
File f = new File(filename);
// Surround the next command inside a try/catch block,
// as it is possible the file doesn't exist.
try {
Scanner fileContents = new Scanner(f);
while (fileContents.hasNextLine()) {
// 1. Get the next line and store in a variable
// 2. Check the length of the line. If the line is not an
// empty string, add the character at the start of the line
// to the result string.
// 3. Otherwise, add an empty space to the result string.
}
fileContents.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
return result;
}
public static String readNthChars (String filename) {
String result = "";
// This is a very similar method, except that instead of
// reading the first letter, you will read the nth character, where
// 'n' is the same as the line number (using a numbering system
// that starts at 0, so the first line has n = 0). Blank lines in
// the text file should not be counted when determining line number,
// but should still contribute spaces as in the method above. Spaces
// within line should also NOT be counted, so you might want to use a
// command such as the following in your code:
// currentLine = currentLine.replaceAll("\\s", "");
// This command removes all the spaces in the String 'currentLine'
// You should also use the command:
// currentLine = currentLine.toUpperCase() at some point, so that the
// result is formatted all in upper case letters.
return result;
}
public static void main(String[] args) {
System.out.println(readFirstLetters("PE_file_1.txt"));
System.out.println(readNthChars("PE_file_2.txt"));
// Your code here to write the two lines above to an output file ("PE_output.txt").
// Hint: You might want to start with: FileWriter myWriter = new FileWriter("PE_output.txt");
// You should then be able to use myWriter.write("some string here");
// To move to a new line, use: myWriter.write("\n");
// You should check your output file to see that you got the output you wanted.
// To find your output file, look in your eclipse workspace.
}
}
PE_file_1.txt
Can of Soup - 1
Sweet potatoes - 5
Ice cream - 1 gallon
Swiss cheese - 1/2 pound
Fish - 1.5 pounds
Unsweetened soy milk
Nuts, assorted - 1 can
PE_file_2.txt
Can I write a functional Java program?
Is it possible to get a CS internship?
Am I a good programmer?
Can someone explain what good coding style is?
How is data science related to computer science?
What attributes should a good programmer have?
How can I become a better programmer?
What will I do after this class?
How do you learn how to debug a program?
How much effort does it take to prep for a technical interview?
How come I usually can't understand everything in my CS class?
What do you consider the best advice for someone studying CS?

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 6 images

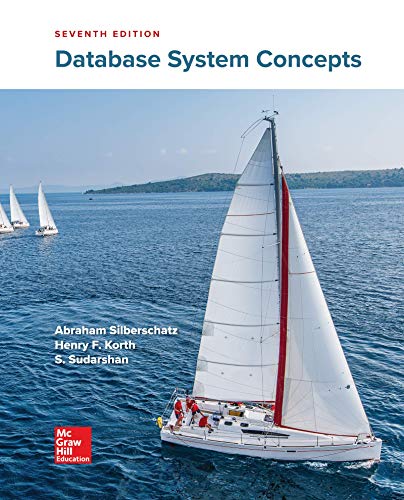
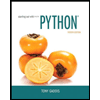
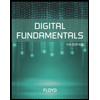
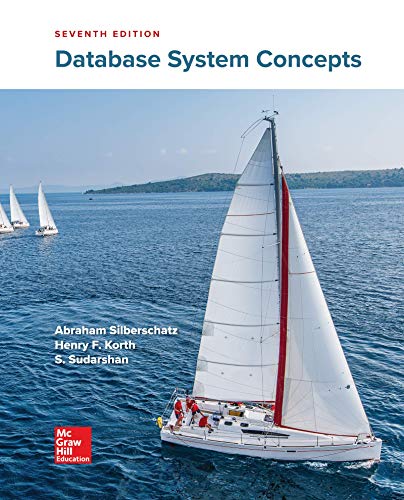
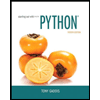
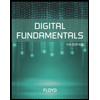
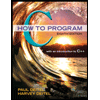
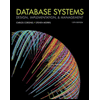
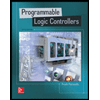