Output for this project: • Whether or not the grid is magic square Programmer's full name Project number Project due date
Output for this project: • Whether or not the grid is magic square Programmer's full name Project number Project due date
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.5: Case Studies
Problem 3E
Related questions
Question
![See the sample outputs for more clarification.
Project Specifications
Input for this project:
Values of the grid (row by row)
Output for this project:
Whether or not the grid is magic square
Programmer's full name
Project number
Project due date
Processing Requirements
Use the following template to start your project:
#include<iostream>
using namespace std;
// Global constants
// The number of rows in the array
// The number of columns in the array
// The value of the smallest number
// The value of the largest number
const int ROWS = 3;
const int COLS = 3;
const int MIN = 1;
const int MAX = 9;
// Function prototypes
bool isMagicsquare(int arrayRow1[], int arrayRow2 [], int arrayRow3[], int size);
bool checkRange (int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int
max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkColiSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
void fillArray(int arrayRowl[], int arrayRow2[], int arrayRow3[], int size);
void showArray (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
int main ()
/* Define a Lo Shu Magic Square using 3 parallel arrays corresponding
to each row of the grid */
int magicArrayRowl [COLS], magicArrayRow2 [COLS], magicArrayRow3 [COLS];
// Your code goes here
return 0;
// Function definitions go here
2](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faa2476d0-9f22-483b-a8fb-d8c41f347393%2Ff9308b7f-54b2-4296-b6cc-b3f0fd962132%2F417c5jh_processed.jpeg&w=3840&q=75)
Transcribed Image Text:See the sample outputs for more clarification.
Project Specifications
Input for this project:
Values of the grid (row by row)
Output for this project:
Whether or not the grid is magic square
Programmer's full name
Project number
Project due date
Processing Requirements
Use the following template to start your project:
#include<iostream>
using namespace std;
// Global constants
// The number of rows in the array
// The number of columns in the array
// The value of the smallest number
// The value of the largest number
const int ROWS = 3;
const int COLS = 3;
const int MIN = 1;
const int MAX = 9;
// Function prototypes
bool isMagicsquare(int arrayRow1[], int arrayRow2 [], int arrayRow3[], int size);
bool checkRange (int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int
max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkColiSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
bool checkDiagSum(int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
void fillArray(int arrayRowl[], int arrayRow2[], int arrayRow3[], int size);
void showArray (int arrayrow1[], int arrayrow2[], int arrayrow3[], int size);
int main ()
/* Define a Lo Shu Magic Square using 3 parallel arrays corresponding
to each row of the grid */
int magicArrayRowl [COLS], magicArrayRow2 [COLS], magicArrayRow3 [COLS];
// Your code goes here
return 0;
// Function definitions go here
2

Transcribed Image Text:The Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below.
4
9
2
3
7
8
1
6
The Lo Shu Magic Square has the following properties:
• The grid contains the numbers 1 - 9 exactly
• The sum of each row, each column and each diagonal all add up to the same
number.
This is shown below:
15
9
2
15
3
5
7
+ 15
8
1
6
+15
15
15 15 15
Write a program that simulates a magic square using 3 one dimensional parallel
arrays of integer type.
Each one the arrays corresponds to a row of the magic square.
The program asks the user to enter the values of the magic square row by row and
informs the user if the grid is a magic square or not.
4.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
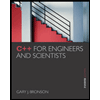
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
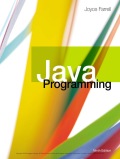
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
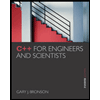
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
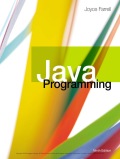
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
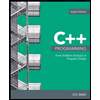
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning