Music App 1. practise file I/O and exception handling; 2. practise String processing. Aim: Task: Create a Java application that transcripts a simple song score sheet. The program asks user for filename of a text file that contains a simple song score sheet in this format: Sample song score sheet 1, song1.txt: #simple song with assumed time signature = 4 d1mls2o2s 1mld2o2 dlrimlfls3o1s1flm1rld4 Lines beginning with a hash #' is a comment line. A song contains one or more lines of musical notes, d, r, m, f, s, 1, t and also o meaning "Off' or rest. Each single character note is followed by a single digit integer in [1 – 4], denoting the duration of the note in number of beats. After transcription, the program outputs the melody on the screen as well as to another text file: Melody output 1, on screen AND in melody_song1.txt: #simple song with assumed time signature Do Me So- | Off- So Me Do Re Me Fa So-- Off = 4 Do- Off- | So Fa Me Re| Do--- The default time signature is 4 beats per section. Sections are delimited by bars '. Notes are converted into full-names with 2 characters, Do, Re, Me, Fa, So, La, Ti, and Off. Notes lasting for one beat are displayed directly. A note lasting two or more beats are elongated with a display of dashes -', e.g., So-- for s3. Notes and bars are delimited by a space. Time signature can be changed with a newline starting with an asterisk *', followed by a single digit integer n in [1- 6]. Transcript and output such a line as "#Time Sign ture = n", e.g., Sample song score sheet 2, song2.txt: #song with different time signatures *3 dirimlo3m1rld1 d1rimlflslols1f1m1rld2 *2 d2r1mlfls111t1 *4 d4r4o2m2 Melody output 2, on screen AND in melody_song2.txt: #song with different time signatures #Time Signature = 3 Do Re Me Off-- Me Re Do Do Re Me Fa So Off so Fa Me l Re Do-
Music App 1. practise file I/O and exception handling; 2. practise String processing. Aim: Task: Create a Java application that transcripts a simple song score sheet. The program asks user for filename of a text file that contains a simple song score sheet in this format: Sample song score sheet 1, song1.txt: #simple song with assumed time signature = 4 d1mls2o2s 1mld2o2 dlrimlfls3o1s1flm1rld4 Lines beginning with a hash #' is a comment line. A song contains one or more lines of musical notes, d, r, m, f, s, 1, t and also o meaning "Off' or rest. Each single character note is followed by a single digit integer in [1 – 4], denoting the duration of the note in number of beats. After transcription, the program outputs the melody on the screen as well as to another text file: Melody output 1, on screen AND in melody_song1.txt: #simple song with assumed time signature Do Me So- | Off- So Me Do Re Me Fa So-- Off = 4 Do- Off- | So Fa Me Re| Do--- The default time signature is 4 beats per section. Sections are delimited by bars '. Notes are converted into full-names with 2 characters, Do, Re, Me, Fa, So, La, Ti, and Off. Notes lasting for one beat are displayed directly. A note lasting two or more beats are elongated with a display of dashes -', e.g., So-- for s3. Notes and bars are delimited by a space. Time signature can be changed with a newline starting with an asterisk *', followed by a single digit integer n in [1- 6]. Transcript and output such a line as "#Time Sign ture = n", e.g., Sample song score sheet 2, song2.txt: #song with different time signatures *3 dirimlo3m1rld1 d1rimlflslols1f1m1rld2 *2 d2r1mlfls111t1 *4 d4r4o2m2 Melody output 2, on screen AND in melody_song2.txt: #song with different time signatures #Time Signature = 3 Do Re Me Off-- Me Re Do Do Re Me Fa So Off so Fa Me l Re Do-
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![Music App
1. practise file I/O and exception handling;
2. practise String processing.
Aim:
Task: Create a Java application that transcripts a simple song score sheet.
The program asks user for filename of a text file that contains a simple song score sheet in this format:
Sample song score sheet 1, song1.txt:
#simple song with assumed time signature = 4
d1mls202s 1mld2o2
d1rimlfls 301s1f1m1rld4
Lines beginning with a hash #' is a comment line. A song contains one or more lines of musical
notes, d, r, m, f, s, l, tand also o meaning "Off" or rest. Each single character note is
followed by a single digit integer in [1 – 4], denoting the duration of the note in number of beats.
After transcription, the program outputs the melody on the screen as well as to another text file:
Melody output 1, on screen AND in melody_song1.txt:
#simple song with assumed time signature
Do Me So- Off- So Me
Do Re Me Fa| So-- Off
= 4
Do- Off- |
So Fa Me Re Do---
|
The default time signature is 4 beats per section. Sections are delimited by bars '. Notes are
converted into full-names with 2 characters, Do, Re, Me, Fa, So, La, Ti, and Off. Notes lasting for
one beat are displayed directly. A note lasting two or more beats are elongated with a display of
dashes -', e.g., So-- for s3. Notes and bars are delimited by a space.
Time signature can be changed with a newline starting with an asterisk *', followed by a single digit
integer n in [1– 6]. Transcript and output such a line as "#Time Signature = n", e.g.,
Sample song score sheet 2, song2.txt:
#song with different time signatures
*3
d1rimlo3m1r1d1
d1rımlflslols1flm1r1d2
*2
d2r1mlfls111t1
*4
d4r4o2m2
Melody output 2, on screen AND in melody_song2.txt:
#song with different time signatures
#Time Signature = 3
Do Re Me Off--| Me Re Do
Do Re Me Fa So Off So Fa Me
Re Do-](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fab68ef6f-9760-4c1f-88a8-6e5ceb2168b2%2F7481e75c-923c-48c0-b1c6-509abdc0178a%2F3oog1yr_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Music App
1. practise file I/O and exception handling;
2. practise String processing.
Aim:
Task: Create a Java application that transcripts a simple song score sheet.
The program asks user for filename of a text file that contains a simple song score sheet in this format:
Sample song score sheet 1, song1.txt:
#simple song with assumed time signature = 4
d1mls202s 1mld2o2
d1rimlfls 301s1f1m1rld4
Lines beginning with a hash #' is a comment line. A song contains one or more lines of musical
notes, d, r, m, f, s, l, tand also o meaning "Off" or rest. Each single character note is
followed by a single digit integer in [1 – 4], denoting the duration of the note in number of beats.
After transcription, the program outputs the melody on the screen as well as to another text file:
Melody output 1, on screen AND in melody_song1.txt:
#simple song with assumed time signature
Do Me So- Off- So Me
Do Re Me Fa| So-- Off
= 4
Do- Off- |
So Fa Me Re Do---
|
The default time signature is 4 beats per section. Sections are delimited by bars '. Notes are
converted into full-names with 2 characters, Do, Re, Me, Fa, So, La, Ti, and Off. Notes lasting for
one beat are displayed directly. A note lasting two or more beats are elongated with a display of
dashes -', e.g., So-- for s3. Notes and bars are delimited by a space.
Time signature can be changed with a newline starting with an asterisk *', followed by a single digit
integer n in [1– 6]. Transcript and output such a line as "#Time Signature = n", e.g.,
Sample song score sheet 2, song2.txt:
#song with different time signatures
*3
d1rimlo3m1r1d1
d1rımlflslols1flm1r1d2
*2
d2r1mlfls111t1
*4
d4r4o2m2
Melody output 2, on screen AND in melody_song2.txt:
#song with different time signatures
#Time Signature = 3
Do Re Me Off--| Me Re Do
Do Re Me Fa So Off So Fa Me
Re Do-

Transcribed Image Text:#Time Signature
Do- Re Me Fa So La Ti
#Time Signature =
= 2
4
Do---
| Re---
| Off- Me-
Then, the program terminates.
To simplify this task, we assume:
Input song text files always contain valid data.
The time signature designation and the note durations are always valid.
Section bars shall NOT cut a multiple beat notes, e.g., Do--
A section shall not continue between two lines.
We accept optionally an extra space at the end of the output lines.
Re- will NOT happen.
Procedure:
1.
Work under a NetBeans Java Application project MusicApp. Name the package as musicapp.
2. Complete the program according to the above descriptions as well as the samples given.
Note that file operations may fail. We give the user THREE chances to input a proper input
filename. If all fails, the program terminates with System. exit (1).
Song filename: a.exe
Something wrong!
Song filename: C:/xyz
Something wrong!
Song filename:
Something wrong!
a
3.
Screen output of a complete sample run with text in blue indicates user keyboard input:
Song filename: a
Something wrong!
Song filename: a.exe
Something wrong!
Song filename: song2.txt
Reading song file song2.txt
#song with different time signatures
#Time Signature = 3
Do Re Me
Do Re Me
#Time Signature = 2
Do- Re Me Fa So | La Ti
#Time Signature = 4
Off-- Me Re Do ||
Fa So Off | So Fa Me | Re Do- |
Do---
| Re---
| Off- Me- |
The program also outputs those text in yellow highlight to another output text file named
melody_song2.txt,i.e., prefixed melody_<inputFilename>.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
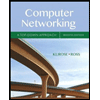
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
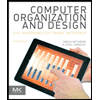
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
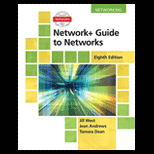
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
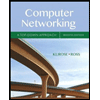
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
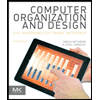
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
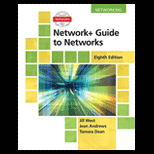
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
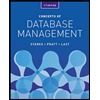
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
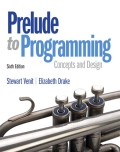
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
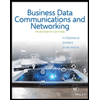
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY