Modify your program from stage 2 so that it reads the input file (parameter 1), storing all the records into a dynamic array in memory. The program should then sort the data records and write the output file (parameter 2) in sorted order. The automarker will test your program by running it many times, each time with a different input file name and an output file name, and it will then compare your output file with the expected output file. Use the Linux library sort routine qsort to perform the sorting. Hint: you must write a comparison routine that can compare two structures according to the sort order specified for your lab. Your program will need to store all the records in memory in order to sort them. The program will allocate a dynamic array of structs (or some other data structure), and read the data file into the array. You do not know how large the file may be, so you must accommodate different file sizes. Here are two possible approaches (there are others).
Hey, I need assistance with this question;
The main reason I can't do it is I don't really understand the malloc, realloc and the whole resizing arrays and how to adapt that to my code. If you could assist me with the changes below that would be really helpful.
Modify your
1. Dynamic sized array: Allocate an initial array of some size (e.g. 100 records) and then if (while reading the file) you find that the array is not large enough then use realloc to increase (e.g. double) the size of it. Realloc allocates a new larger array in memory and copies the data from the existing array to the new larger array, before freeing the original array. Repeatedly doubling the size allows you to accommodate arbitrarily large data files without copying the data too many times.
2. Compute the number of records from the file size: This is a systems approach that will require some reading to find out how to achieve. There is a system call stat that can tell you the total number of bytes in a file. There are also other ways to find out how many bytes are in a file but you should NOT read the entire file just to find out how big it is! Your file description gives you the information about how long each record is, so you can compute the number of records in the file from the number of bytes. You can then allocate an array of struct to the exact correct size using malloc. See the Unix manual pages for stat and malloc. After sorting the records, write them out in binary form. It is suggested to use fwrite to write each field individually.
Previous Code:
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
#define MAX_SIZE 7
#define MAX_SINGLECHAR 1
struct stage2 {
char basketball;
short int brass;
short int airport;
short int popcorn;
char donkey; // decimal
char floor;
double bit;
float prose;
unsigned long match;
char holiday[MAX_SIZE];
unsigned char bells;
short loss;
char boy;
int mice;
char patch;
double dad;
unsigned int neck;
};
void usage() {
fprintf(stderr,"Program Usage: Error");
exit(2);
}
int main (int argc, char *argv[]) {
FILE *fpn;
char ch;
struct stage2 fd;
if (argc != 2) {
fprintf(stderr, "Usage: %s Filenamen", argv[0]);
exit(1);
} else {
fpn = fopen(argv[1], "r");
}
if (fpn == 0) {
fprintf(stderr, "File: %s is non existantn", argv[1]);
exit(1);
} else {
printf("basketball, brass, airport, popcorn, donkey, floor, bit, prose, match, holiday, bells, loss, boy, mice, patch, dad, neckn");
while (!feof(fpn)) {
fread(&fd.basketball, sizeof(fd.basketball), 1, fpn);
fread(&fd.brass, sizeof(fd.brass), 1, fpn);
fread(&fd.airport, sizeof(fd.airport), 1, fpn);
fread(&fd.popcorn, sizeof(fd.popcorn), 1, fpn);
fread(&fd.donkey, sizeof(fd.donkey), 1, fpn);
fread(&fd.floor, sizeof(fd.floor), 1, fpn);
fread(&fd.bit, sizeof(fd.bit), 1, fpn);
fread(&fd.prose, sizeof(fd.prose), 1, fpn);
fread(&fd.match, sizeof(fd.match), 1, fpn);
fread(&fd.holiday, sizeof(fd.holiday), 1, fpn);
fread(&fd.bells, sizeof(fd.bells), 1, fpn);
fread(&fd.loss, sizeof(fd.loss), 1, fpn);
fread(&fd.boy, sizeof(fd.boy), 1, fpn);
fread(&fd.mice, sizeof(fd.mice), 1, fpn);
fread(&fd.patch, sizeof(fd.patch), 1, fpn);
fread(&fd.dad, sizeof(fd.dad), 1, fpn);
fread(&fd.neck, sizeof(fd.neck), 1, fpn);
if (feof(fpn)) {
break;
}
printf("%d, %d, %d, %d, %hhx, %c, %f, %f, %lu, %s, %d, %d, %d, %d, %d, %f, %un", fd.basketball, fd.brass, fd.airport, fd.popcorn, fd$
}
fclose(fpn);
}
return 0;
}

Step by step
Solved in 3 steps

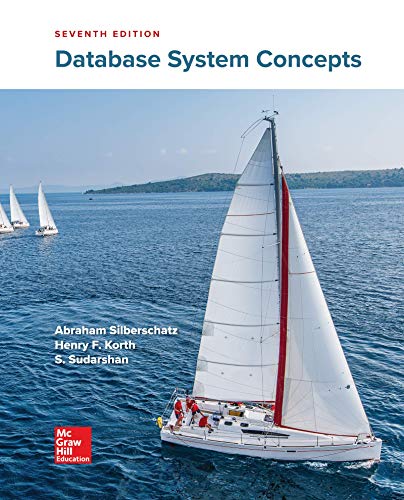
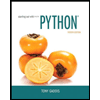
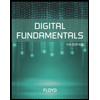
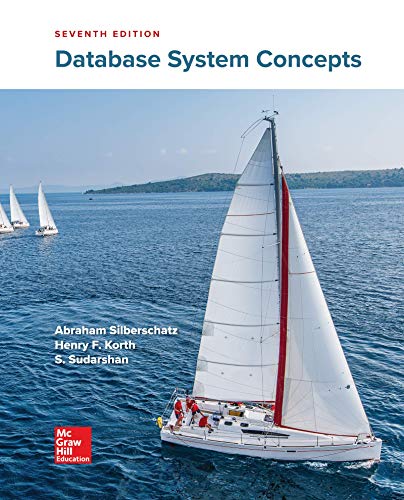
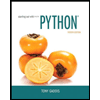
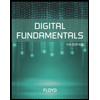
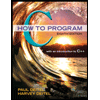
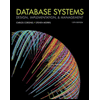
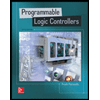