Math 130 Java Programming Does my code pass the requirements? May I have more explanation? Is my code readable? Is my code well commented? How may I better organize my code? Are there whitespaces to appropriate help separate distinct parts? import java.util.*; public class NumberArray { //method definition of printArray() which accepts //array as parameter public static void printArray(int[] array) { System.out.printf(" "); //displaying the array elements for(int j = 0; j < array.length; j++) { if(j > 0 && j % 6 ==0) { System.out.printf("\n"); System.out.printf(" "); } System.out.printf("%3d ", array[j]); } } //method definition of getTotal() //which accepts array as parameter public static double getTotal(int[]array) { //declare variable sum as double and set 0 double sum = 0; //using for loop, calculate the total of all elements in the array for(int j = 0; j < array.length; j++) { sum+= array[j]; } //returning sum to the calling method return sum; } //method definition of getAverage() //which accepts array as parameter public static double getAverage(int[]array) { //calculating the average by calling //getTotal() method and returning average to //the calling function return getTotal(array) / (double) array.length; } //method definition of getHighest() //which accepts array as parameter public static int getHighest(int[]array) { //set first element of array as max int max = array[0]; //comparing each element of the array //finding the max element in the array for(int j = 0; j < array.length; j++) { if(array[j] > max) { max = array[j]; } } //returning max to the calling function return max; } //method definition of getLowest() //which accepts array as parameter public static int getLowest(int[]array) { //set first element of array as min int min = array[0]; //comparing each element of the array //finding the min element in the array for(int j = 0; j < array.length; j++) { if(array[j] < min) { min = array[j]; } } //returning min to the calling function return min; } //method definition of countOccurrences() //which accepts array and value as parameters public static int countOccurrences(int[] array, int value) { //set count as 0 int count=0; //using for loop, count the occurrence of given value for(int i = 0; i
Math 130 Java Programming Does my code pass the requirements? May I have more explanation? Is my code readable? Is my code well commented? How may I better organize my code? Are there whitespaces to appropriate help separate distinct parts? import java.util.*; public class NumberArray { //method definition of printArray() which accepts //array as parameter public static void printArray(int[] array) { System.out.printf(" "); //displaying the array elements for(int j = 0; j < array.length; j++) { if(j > 0 && j % 6 ==0) { System.out.printf("\n"); System.out.printf(" "); } System.out.printf("%3d ", array[j]); } } //method definition of getTotal() //which accepts array as parameter public static double getTotal(int[]array) { //declare variable sum as double and set 0 double sum = 0; //using for loop, calculate the total of all elements in the array for(int j = 0; j < array.length; j++) { sum+= array[j]; } //returning sum to the calling method return sum; } //method definition of getAverage() //which accepts array as parameter public static double getAverage(int[]array) { //calculating the average by calling //getTotal() method and returning average to //the calling function return getTotal(array) / (double) array.length; } //method definition of getHighest() //which accepts array as parameter public static int getHighest(int[]array) { //set first element of array as max int max = array[0]; //comparing each element of the array //finding the max element in the array for(int j = 0; j < array.length; j++) { if(array[j] > max) { max = array[j]; } } //returning max to the calling function return max; } //method definition of getLowest() //which accepts array as parameter public static int getLowest(int[]array) { //set first element of array as min int min = array[0]; //comparing each element of the array //finding the min element in the array for(int j = 0; j < array.length; j++) { if(array[j] < min) { min = array[j]; } } //returning min to the calling function return min; } //method definition of countOccurrences() //which accepts array and value as parameters public static int countOccurrences(int[] array, int value) { //set count as 0 int count=0; //using for loop, count the occurrence of given value for(int i = 0; i
Chapter8: Arrays
Section: Chapter Questions
Problem 20RQ
Related questions
Question
100%
Math 130 Java Programming
Does my code pass the requirements? May I have more explanation? Is my code readable? Is my code well commented? How may I better organize my code? Are there whitespaces to appropriate help separate distinct parts?
import java.util.*;
public class NumberArray
{
//method definition of printArray() which accepts
//array as parameter
public static void printArray(int[] array)
{
System.out.printf(" ");
//displaying the array elements
for(int j = 0; j < array.length; j++)
{
if(j > 0 && j % 6 ==0)
{
System.out.printf("\n");
System.out.printf(" ");
}
System.out.printf("%3d ", array[j]);
}
}
//method definition of getTotal()
//which accepts array as parameter
public static double getTotal(int[]array)
{
//declare variable sum as double and set 0
double sum = 0;
//using for loop, calculate the total of all elements in the array
for(int j = 0; j < array.length; j++)
{
sum+= array[j];
}
//returning sum to the calling method
return sum;
}
//method definition of getAverage()
//which accepts array as parameter
public static double getAverage(int[]array)
{
//calculating the average by calling
//getTotal() method and returning average to
//the calling function
return getTotal(array) / (double) array.length;
}
//method definition of getHighest()
//which accepts array as parameter
public static int getHighest(int[]array)
{
//set first element of array as max
int max = array[0];
//comparing each element of the array
//finding the max element in the array
for(int j = 0; j < array.length; j++)
{
if(array[j] > max)
{
max = array[j];
}
}
//returning max to the calling function
return max;
}
//method definition of getLowest()
//which accepts array as parameter
public static int getLowest(int[]array)
{
//set first element of array as min
int min = array[0];
//comparing each element of the array
//finding the min element in the array
for(int j = 0; j < array.length; j++)
{
if(array[j] < min)
{
min = array[j];
}
}
//returning min to the calling function
return min;
}
//method definition of countOccurrences()
//which accepts array and value as parameters
public static int countOccurrences(int[] array, int value)
{
//set count as 0
int count=0;
//using for loop, count the occurrence of given value
for(int i = 0; i<array.length; i++)
{
if(array[i] == value)
{
count++;
}
}
//returning count to the calling function
return count;
}
//method definition of removeAll()
//which accepts array and value as parameters
public static int[] removeAll(int[] array, int value)
{
//invoke a method countOccurrences() by passing array and value
//as arguments
int count=countOccurrences(array, value);
//creating new array based on the count value
int[] newArray = new int[array.length - count];
//set valuesAdded as 0
int valuesAdded = 0;
//using for loop, add the elements to the new array
for(int i = 0; i < array.length; i++)
{
if(array[i] != value)
{
newArray[valuesAdded] = array[i];
valuesAdded++;
}
}
//returning newArray to the calling function
return newArray;
}
public static void main(String[] args)
{
Scanner keyboard = new Scanner(System.in);
System.out.printf("Please enter the size of array:");
//getting size of the array from the user
int array_size = keyboard.nextInt();
//creating new array
int[] array = new int[array_size];
//set sum, average, max and min as 0
int sum = 0;
int average = 0;
int max = 0;
int min = 0;
for(int i = 0; i<array_size; i++)
{
System.out.printf("Please enter an integer:");
//getting elements of the array from the user
int number = keyboard.nextInt();
array[i] = number;
}
System.out.printf("Here is a printout of the original array:" + "\n");
//invoke a method printArray() by passing array as argument
printArray(array);
//display the information on the console
//by invoking appropriate methods
System.out.printf("\nThe sum of all the elements in the array:" + getTotal(array));
System.out.printf("\nThe average of all the elements in the array:" + String.format("%.2f", getAverage(array)));
System.out.printf("\nThe max of all the elements in the array:" + getHighest(array));
System.out.printf("\nThe min of all the elements in the array:" + getLowest(array) + "\n");
System.out.printf("\nWhat value you would like to be removed:");
//get the value from the user to remove
int value = keyboard.nextInt();
//getting occurrences of value by invoking countOccurrences() method
System.out.printf("\nThe number of occurrences of " + value + " in the array is: " + countOccurrences(array, value));
System.out.printf("\nHere is a printout of the new array with number " + value + " removed:" + "\n");
//remove the element from the array using removeAll() method
array = removeAll(array, value);
//invoke a method printArray() by passing array as argument
printArray(array);
keyboard.close();
}
}
Thank you so much!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
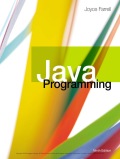
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
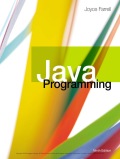
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage