Make sure the program run smooth and perfectly, make sure no error encounter. compatible for python software. Complete the sample code template to implement a student list as a binary search tree (BST). Specification 1. Each Studentrecord should have the following fields: =studentNumber =lastname =firstName =course =gpa 2. Run the program from an appropriate menu allowing for the following operations: * Adding a Student record * Deleting a Student record * Listing students by: • All students • A given course • GPA above a certain value • GPA below a certain value class Record: def __init__(self, studentNumber = None, lastname = None, firstName = None, course = None, gpa = None,): self.studentNumber = studentNumber self.lastname = lastname self.firstName = firstName self.course = course self.gpa = gpa def __str__(self): record = "\n\nStudent Number : {}\n".format(self.studentNumber) record += "Lastname : {}\n".format(self.lastname) record += "FirstName : {}\n".format(self.firstName) record += "Course : {}\n".format(self.course) record += "GPA : {}\n".format(self.gpa) return record class Node: def __init__(self, record = None): self.leftchild = None self.record = record self.parentchild = None self.rightchild = None def __str__(self): return self.record class StudentListBST: def __init__(self): self.root = None def add_student(self, record): x = self.root y = None z = Node(record) while x is not None: y = x if z.record.studentNumber < x.record.studentNumber: x = x.leftchild else: x = x.rightchild z.parent = y if y is None: self.root = z else: if z.record.studentNumber < y.record.studentNumber: y.leftchild = z else: y.rightchild = z def inorderTraversal(self, root): if root is not None: self.inorderTraversal(root.leftchild) print(root.record, end=" ") self.inorderTraversal(root.rightchild) """ def delete_student(self, delptr): # Note: The delptr parameter of delete_student() should be # pointed to the node to be deleted before calling the function. def list_all(self): def list_by_a_given_course(self, course): def list_above_given_gpa_value(self, gpa): def list_below_given_gpa_value(self, gpa): """ student_list_bst = StudentListBST() while True: try: no_students = int(input('How many students? : ')) if no_students >= 1 and no_students <= 10: break else: raise Exception() except Exception: print("Error! Size should be between 1 and 10") for i in range(no_students): studentNumber = input("Enter student number : ") lastname = input("Enter lastname : ") firstname = input("Enter firstname : ") course = input("Enter course : ") gpa = input("Enter GPA : ") record = Record(studentNumber, lastname, firstname, course, gpa) student_list_bst.add_student(record) student_list_bst.inorderTraversal(student_list_bst.root)
Make sure the program run smooth and perfectly, make sure no error encounter. compatible for python software.
Complete the sample code template to implement a student list as a binary search tree (BST).
Specification
1. Each Studentrecord should have the following fields:
=studentNumber
=lastname
=firstName
=course
=gpa
2. Run the program from an appropriate menu allowing for the following operations:
* Adding a Student record
* Deleting a Student record
* Listing students by:
• All students
• A given course
• GPA above a certain value
• GPA below a certain value
class Record:
def __init__(self, studentNumber = None, lastname = None, firstName = None, course = None, gpa =
None,):
self.studentNumber = studentNumber
self.lastname = lastname
self.firstName = firstName
self.course = course
self.gpa = gpa
def __str__(self):
record = "\n\nStudent Number : {}\n".format(self.studentNumber)
record += "Lastname : {}\n".format(self.lastname)
record += "FirstName : {}\n".format(self.firstName)
record += "Course : {}\n".format(self.course)
record += "GPA : {}\n".format(self.gpa)
return record
class Node:
def __init__(self, record = None):
self.leftchild = None
self.record = record
self.parentchild = None
self.rightchild = None
def __str__(self):
return self.record
class StudentListBST:
def __init__(self):
self.root = None
def add_student(self, record):
x = self.root
y = None
z = Node(record)
while x is not None:
y = x
if z.record.studentNumber < x.record.studentNumber:
x = x.leftchild
else:
x = x.rightchild
z.parent = y
if y is None:
self.root = z
else:
if z.record.studentNumber < y.record.studentNumber:
y.leftchild = z
else:
y.rightchild = z
def inorderTraversal(self, root):
if root is not None:
self.inorderTraversal(root.leftchild)
print(root.record, end=" ")
self.inorderTraversal(root.rightchild)
"""
def delete_student(self, delptr): # Note: The delptr parameter of delete_student() should be
# pointed to the node to be deleted before calling the function.
def list_all(self):
def list_by_a_given_course(self, course):
def list_above_given_gpa_value(self, gpa):
def list_below_given_gpa_value(self, gpa):
"""
student_list_bst = StudentListBST()
while True:
try:
no_students = int(input('How many students? : '))
if no_students >= 1 and no_students <= 10:
break
else:
raise Exception()
except Exception:
print("Error! Size should be between 1 and 10")
for i in range(no_students):
studentNumber = input("Enter student number : ")
lastname = input("Enter lastname : ")
firstname = input("Enter firstname : ")
course = input("Enter course : ")
gpa = input("Enter GPA : ")
record = Record(studentNumber, lastname, firstname, course, gpa)
student_list_bst.add_student(record)
student_list_bst.inorderTraversal(student_list_bst.root)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

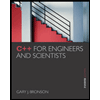
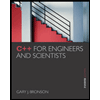