lled myGraph and a node class (if necessary) using composition. #include #incl
I need to convert this code using C++, that currently works with structs into classes, with the main class being called myGraph and a node class (if necessary) using composition.
#include <stdio.h>
#include <stdlib.h>
#include <iostream>
using namespace std;
struct AdjListNode //node class
{
int dest;
struct AdjListNode* next;
};
struct AdjList //node class
{
struct AdjListNode *head;
};
struct Graph //myGraph class
{
int V;
struct AdjList* array;
};
struct AdjListNode * newAdjListNode(int dest)
{
struct AdjListNode * newNode = new AdjListNode;
newNode->dest = dest;
newNode->next = NULL;
return newNode;
}
struct Graph* createGraph(int V) //constructor
{
struct Graph* graph = new Graph;
graph->V = V;
graph->array = new AdjList;
int i;
for (i = 0; i < V; ++i)
graph->array[i].head = NULL;
return graph;
}
void addEdge(struct Graph* graph, int src, int dest)
{
struct AdjListNode* newNode = newAdjListNode(dest);
newNode->next = graph->array[src].head;
graph->array[src].head = newNode;
newNode = newAdjListNode(src);
newNode->next = graph->array[dest].head;
graph->array[dest].head = newNode;
}
void printGraph(struct Graph* graph)
{
int v;
for (v = 0; v < graph->V; ++v)
{
struct AdjListNode* pCrawl = graph->array[v].head;
cout << endl << "Adjacency list of vertex " << v << endl << " head";
while (pCrawl)
{
cout << "-> " << pCrawl->dest;
pCrawl = pCrawl->next;
}
cout << endl;
}
}
int main()
{
int V = 5;
struct Graph* graph = createGraph(V);
addEdge(graph, 0, 1);
addEdge(graph, 0, 4);
addEdge(graph, 1, 2);
addEdge(graph, 1, 3);
addEdge(graph, 1, 4);
addEdge(graph, 2, 3);
addEdge(graph, 3, 4);
printGraph(graph);
system("pause");
return 0;
}

Step by step
Solved in 4 steps with 2 images

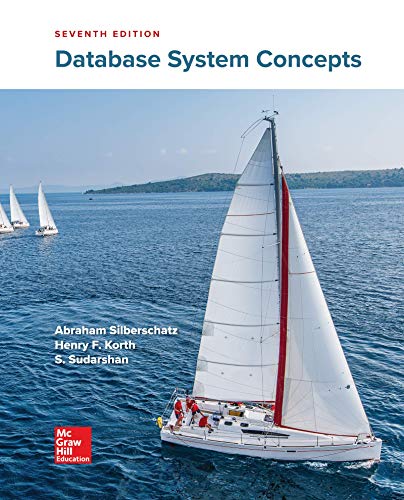
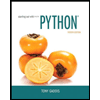
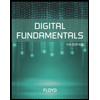
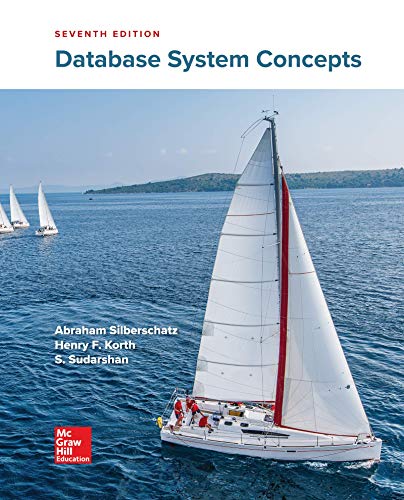
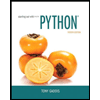
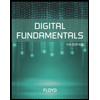
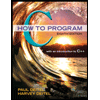
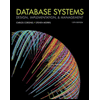
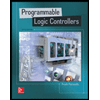