LINKED LIST IMPLEMENTATION Linked list Write a C++ program to implement insertion, deletion, and display operations in a Linked List Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Node has the following member variable Datatype Variable Usage int data to store data Node* next to store the next node Define the following public member functions in the class LinkedList. Member function Function description void insertNode(int value) This function inserts the data into the linked list at the end void deleteNode(int value) This function deletes the node from the linked list void display() This function is used to display the nodes in the linked list In the main() function, read inputs and call the functions of the LinkedList class based on the inputs. Note: If the Linked list is empty while displaying, then print "List is empty" in the display() function. Input and Output Format: Refer to sample input and output for formatting specifications. [All text in bold corresponds to input and the rest corresponds to output.] Sample Input and Output 1: 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 1 Enter the element to be inserted : 10 Element 10 is inserted into the Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 1 Enter the element to be inserted : 3 Element 3 is inserted into the Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 1 Enter the element to be inserted : 2 Element 2 is inserted into the Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 3 10 3 2 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 2 Enter the element to be deleted : 3 Element 3 is deleted from Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 3 10 2 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 4 Sample Input and Output 2: 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 3 List is empty 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 4
LINKED LIST IMPLEMENTATION Linked list Write a C++ program to implement insertion, deletion, and display operations in a Linked List Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. The class Node has the following member variable Datatype Variable Usage int data to store data Node* next to store the next node Define the following public member functions in the class LinkedList. Member function Function description void insertNode(int value) This function inserts the data into the linked list at the end void deleteNode(int value) This function deletes the node from the linked list void display() This function is used to display the nodes in the linked list In the main() function, read inputs and call the functions of the LinkedList class based on the inputs. Note: If the Linked list is empty while displaying, then print "List is empty" in the display() function. Input and Output Format: Refer to sample input and output for formatting specifications. [All text in bold corresponds to input and the rest corresponds to output.] Sample Input and Output 1: 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 1 Enter the element to be inserted : 10 Element 10 is inserted into the Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 1 Enter the element to be inserted : 3 Element 3 is inserted into the Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 1 Enter the element to be inserted : 2 Element 2 is inserted into the Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 3 10 3 2 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 2 Enter the element to be deleted : 3 Element 3 is deleted from Linked list 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 3 10 2 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 4 Sample Input and Output 2: 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 3 List is empty 1.Insertion 2.Deletion 3.Display 4.Exit Enter your choice : 4
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 13PE
Related questions
Question
LINKED LIST IMPLEMENTATION
Linked list
Write a C++ program to implement insertion, deletion, and display operations in a Linked List
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
The class Node has the following member variable
Datatype | Variable | Usage |
int | data | to store data |
Node* | next | to store the next node |
Define the following public member functions in the class LinkedList.
Member function | Function description |
void insertNode(int value) | This function inserts the data into the linked list at the end |
void deleteNode(int value) | This function deletes the node from the linked list |
void display() | This function is used to display the nodes in the linked list |
In the main() function, read inputs and call the functions of the LinkedList class based on the inputs.
Note:
- If the Linked list is empty while displaying, then print "List is empty" in the display() function.
Input and Output Format:
Refer to sample input and output for formatting specifications.
[All text in bold corresponds to input and the rest corresponds to output.]
Sample Input and Output 1:
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
1
Enter the element to be inserted :
10
Element 10 is inserted into the Linked list
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
1
Enter the element to be inserted :
3
Element 3 is inserted into the Linked list
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
1
Enter the element to be inserted :
2
Element 2 is inserted into the Linked list
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
3
10 3 2
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
2
Enter the element to be deleted :
3
Element 3 is deleted from Linked list
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
3
10 2
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
4
Sample Input and Output 2:
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
3
List is empty
1.Insertion
2.Deletion
3.Display
4.Exit
Enter your choice :
4
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
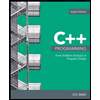
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
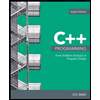
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning