Java Program ASAP Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. Also change the public class to FileSorting and I need only one program. And do the following; 1) For test cases 2 and 3 for the outputted numbers there needs to be commas and there needs to be nothing after that 2)for test case 1 outputted numbers there needs to be nothing after that, 3) for test 4 when file is not found there needs to be nothing after that 4) and for test case 5 and 7 afer a file is not found it needs to display Please re-enter the file name or type QUIT to exit: so you input the text file and displays the numbers. import java.io.*; import java.util.Scanner; public class FileSorting { public static void main(String[] args) throws Exception { Scanner sc = new Scanner(System.in); System.out.println("Please enter the file name or type QUIT to exit:"); while (true) { String input = sc.next(); if (input.equalsIgnoreCase("QUIT")) { break; // Exit the program } else { String filePath = new File("").getAbsolutePath() + "/" + input; File file = new File(filePath); if (file.exists() && !file.isDirectory()) { try (BufferedReader br = new BufferedReader(new FileReader(file))) { String st; StringBuilder formattedText = new StringBuilder(); while ((st = br.readLine()) != null) { if (!st.trim().isEmpty()) { String[] numbers = st.split(","); for (int i = 0; i < numbers.length; i++) { formattedText.append(numbers[i].trim()); if (i < numbers.length - 1) { formattedText.append(","); } } formattedText.append("\n"); } } if (formattedText.toString().trim().isEmpty()) { System.out.println("File " + input + " is empty."); } else { System.out.print(formattedText); } return; // Exit the program after printing } catch (IOException e) { e.printStackTrace(); } } else { System.out.println("File " + input + " is not found."); if (input.equals("input2.csv") || input.equals("input7.csv")) { System.out.println("Please re-enter the file name or type QUIT to exit:"); } } } } } } input2x2.csv -67,-11 -27,-70 input1.csv 10 input10x10.csv 56,-19,-21,-51,45,96,-46 -27,29,-58,85,8,71,34 50,51,40,50,100,-82,-87 -47,-24,-27,-32,-25,46,88 -47,95,-41,-75,85,-16,43 -78,0,94,-77,-69,78,-25 -80,-31,-95,82,-86,-32,-22 68,-52,-4,-68,10,-14,-89 26,33,-59,-51,-48,-34,-52 -47,-24,80,16,80,-66,-42 input0.csv Test Case 1 Please enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 2 Please enter the file name or type QUIT to exit:\n input2x2.csvENTER -67,-11\n -70,-27\n Test Case 3 Please enter the file name or type QUIT to exit:\n input10x10.csvENTER -51,-46,-21,-19,45,56,96\n -58,-27,8,29,34,71,85\n -87,-82,40,50,50,51,100\n -47,-32,-27,-25,-24,46,88\n -75,-47,-41,-16,43,85,95\n -78,-77,-69,-25,0,78,94\n -95,-86,-80,-32,-31,-22,82\n -89,-68,-52,-14,-4,10,68\n -59,-52,-51,-48,-34,26,33\n -66,-47,-42,-24,16,80,80\n Test Case 4 Please enter the file name or type QUIT to exit:\n input0.csvENTER File input0.csv is empty.\n Test Case 5 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n input1.csvENTER 10\n Test Case 6 Please enter the file name or type QUIT to exit:\n quitENTER Test Case 7 Please enter the file name or type QUIT to exit:\n input2.csvENTER File input2.csv is not found.\n Please re-enter the file name or type QUIT to exit:\n QUITENTER
Java Program ASAP
Modify this program so it passes the test cases in Hypergrade becauses it says 5 out of 7 passed. Also change the public class to FileSorting and I need only one program.
And do the following;
1) For test cases 2 and 3 for the outputted numbers there needs to be commas and there needs to be nothing after that
2)for test case 1 outputted numbers there needs to be nothing after that,
3) for test 4 when file is not found there needs to be nothing after that
4) and for test case 5 and 7 afer a file is not found it needs to display Please re-enter the file name or type QUIT to exit: so you input the text file and displays the numbers.
import java.io.*;
import java.util.Scanner;
public class FileSorting {
public static void main(String[] args) throws Exception {
Scanner sc = new Scanner(System.in);
System.out.println("Please enter the file name or type QUIT to exit:");
while (true) {
String input = sc.next();
if (input.equalsIgnoreCase("QUIT")) {
break; // Exit the program
} else {
String filePath = new File("").getAbsolutePath() + "/" + input;
File file = new File(filePath);
if (file.exists() && !file.isDirectory()) {
try (BufferedReader br = new BufferedReader(new FileReader(file))) {
String st;
StringBuilder formattedText = new StringBuilder();
while ((st = br.readLine()) != null) {
if (!st.trim().isEmpty()) {
String[] numbers = st.split(",");
for (int i = 0; i < numbers.length; i++) {
formattedText.append(numbers[i].trim());
if (i < numbers.length - 1) {
formattedText.append(",");
}
}
formattedText.append("\n");
}
}
if (formattedText.toString().trim().isEmpty()) {
System.out.println("File " + input + " is empty.");
} else {
System.out.print(formattedText);
}
return; // Exit the program after printing
} catch (IOException e) {
e.printStackTrace();
}
} else {
System.out.println("File " + input + " is not found.");
if (input.equals("input2.csv") || input.equals("input7.csv")) {
System.out.println("Please re-enter the file name or type QUIT to exit:");
}
}
}
}
}
}
input2x2.csv
-67,-11
-27,-70
input1.csv
10
input10x10.csv
56,-19,-21,-51,45,96,-46
-27,29,-58,85,8,71,34
50,51,40,50,100,-82,-87
-47,-24,-27,-32,-25,46,88
-47,95,-41,-75,85,-16,43
-78,0,94,-77,-69,78,-25
-80,-31,-95,82,-86,-32,-22
68,-52,-4,-68,10,-14,-89
26,33,-59,-51,-48,-34,-52
-47,-24,80,16,80,-66,-42
input0.csv
Test Case 1
input1.csvENTER
10\n
Test Case 2
input2x2.csvENTER
-67,-11\n
-70,-27\n
Test Case 3
input10x10.csvENTER
-51,-46,-21,-19,45,56,96\n
-58,-27,8,29,34,71,85\n
-87,-82,40,50,50,51,100\n
-47,-32,-27,-25,-24,46,88\n
-75,-47,-41,-16,43,85,95\n
-78,-77,-69,-25,0,78,94\n
-95,-86,-80,-32,-31,-22,82\n
-89,-68,-52,-14,-4,10,68\n
-59,-52,-51,-48,-34,26,33\n
-66,-47,-42,-24,16,80,80\n
Test Case 4
input0.csvENTER
File input0.csv is empty.\n
Test Case 5
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
input1.csvENTER
10\n
Test Case 6
quitENTER
Test Case 7
input2.csvENTER
File input2.csv is not found.\n
Please re-enter the file name or type QUIT to exit:\n
QUITENTER



Step by step
Solved in 4 steps with 3 images

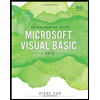
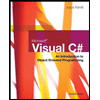
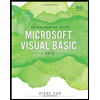
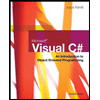