Java help. The file UnsortedLinkedDictionary.java contains unimplemented methods for a dictionary basedon an unsorted linked list. Implement all these methods. The java code is below: import java.util.Iterator;import java.util.NoSuchElementException;public class UnsortedLinkedDictionary<K, V> {public static void main(String args[]){// Add you tests here}public UnsortedLinkedDictionary() {} // end default constructorpublic V add(K key, V value) {} // end addpublic V remove(K key) {} // end removepublic V getValue(K key) {} // end getValuepublic boolean contains(K key) {} // end containspublic boolean isEmpty() {} // end isEmptypublic int getSize() {} // end getSizepublic final void clear() { } // end clearprivate class Node {private K key;private V value;private Node next;private Node(K searchKey, V dataValue) {key = searchKey;value = dataValue;next = null; } // end constructorprivate Node(K searchKey, V dataValue, Node nextNode) {key = searchKey;value = dataValue;next = nextNode; } // end constructorprivate K getKey() {return key;} // end getKeyprivate V getValue() {return value;} // end getValueprivate void setValue(V newValue) {value = newValue;} // end setValueprivate Node getNextNode(){return next;} // end getNextNodeprivate void setNextNode(Node nextNode) {next = nextNode;} // end setNextNode} // end Node} // end UnsortedLinkedDictionary
Java help. The file UnsortedLinkedDictionary.java contains unimplemented methods for a dictionary basedon an unsorted linked list. Implement all these methods. The java code is below: import java.util.Iterator;import java.util.NoSuchElementException;public class UnsortedLinkedDictionary<K, V> {public static void main(String args[]){// Add you tests here}public UnsortedLinkedDictionary() {} // end default constructorpublic V add(K key, V value) {} // end addpublic V remove(K key) {} // end removepublic V getValue(K key) {} // end getValuepublic boolean contains(K key) {} // end containspublic boolean isEmpty() {} // end isEmptypublic int getSize() {} // end getSizepublic final void clear() { } // end clearprivate class Node {private K key;private V value;private Node next;private Node(K searchKey, V dataValue) {key = searchKey;value = dataValue;next = null; } // end constructorprivate Node(K searchKey, V dataValue, Node nextNode) {key = searchKey;value = dataValue;next = nextNode; } // end constructorprivate K getKey() {return key;} // end getKeyprivate V getValue() {return value;} // end getValueprivate void setValue(V newValue) {value = newValue;} // end setValueprivate Node getNextNode(){return next;} // end getNextNodeprivate void setNextNode(Node nextNode) {next = nextNode;} // end setNextNode} // end Node} // end UnsortedLinkedDictionary
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Java help.
The file UnsortedLinkedDictionary.java contains unimplemented methods for a dictionary based
on an unsorted linked list. Implement all these methods.
on an unsorted linked list. Implement all these methods.
The java code is below:
import java.util.Iterator;
import java.util.NoSuchElementException;
public class UnsortedLinkedDictionary<K, V> {
public static void main(String args[])
{
// Add you tests here
}
public UnsortedLinkedDictionary() {
} // end default constructor
public V add(K key, V value) {
} // end add
public V remove(K key) {
} // end remove
public V getValue(K key) {
} // end getValue
public boolean contains(K key) {
} // end contains
public boolean isEmpty() {
} // end isEmpty
public int getSize() {
} // end getSize
public final void clear() {
} // end clear
private class Node {
private K key;
private V value;
private Node next;
private Node(K searchKey, V dataValue) {
key = searchKey;
value = dataValue;
next = null;
} // end constructor
private Node(K searchKey, V dataValue, Node nextNode) {
key = searchKey;
value = dataValue;
next = nextNode;
} // end constructor
private K getKey() {
return key;
} // end getKey
private V getValue() {
return value;
} // end getValue
private void setValue(V newValue) {
value = newValue;
} // end setValue
private Node getNextNode()
{
return next;
} // end getNextNode
private void setNextNode(Node nextNode) {
next = nextNode;
} // end setNextNode
} // end Node
} // end UnsortedLinkedDictionary
import java.util.NoSuchElementException;
public class UnsortedLinkedDictionary<K, V> {
public static void main(String args[])
{
// Add you tests here
}
public UnsortedLinkedDictionary() {
} // end default constructor
public V add(K key, V value) {
} // end add
public V remove(K key) {
} // end remove
public V getValue(K key) {
} // end getValue
public boolean contains(K key) {
} // end contains
public boolean isEmpty() {
} // end isEmpty
public int getSize() {
} // end getSize
public final void clear() {
} // end clear
private class Node {
private K key;
private V value;
private Node next;
private Node(K searchKey, V dataValue) {
key = searchKey;
value = dataValue;
next = null;
} // end constructor
private Node(K searchKey, V dataValue, Node nextNode) {
key = searchKey;
value = dataValue;
next = nextNode;
} // end constructor
private K getKey() {
return key;
} // end getKey
private V getValue() {
return value;
} // end getValue
private void setValue(V newValue) {
value = newValue;
} // end setValue
private Node getNextNode()
{
return next;
} // end getNextNode
private void setNextNode(Node nextNode) {
next = nextNode;
} // end setNextNode
} // end Node
} // end UnsortedLinkedDictionary
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
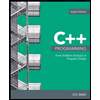
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
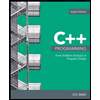
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning