Java: Design the class Circle that can store the radius and the center of the circle based on the class Point we talked in the class. You should be able to perform the usual operation on a circle, such as setting radius, printing the radius, calculating and printing the area and circumference, and carrying out the usual operations on the center.
Java: Design the class Circle that can store the radius and the center of the circle based on the class Point we talked in the class. You should be able to perform the usual operation on a circle, such as setting radius, printing the radius, calculating and printing the area and circumference, and carrying out the usual operations on the center.
Here is the code:
public class Point {
public static void main(String[] args) {
}
protected double x, y; // coordinates of the Point
//Default constructor
public Point()
{
setPoint( 0, 0 );
}
//Constructor with parameters
public Point(double xValue, double yValue )
{
setPoint(xValue, yValue );
}
// set x and y coordinates of Point
public void setPoint(double xValue, double yValue )
{
x = xValue;
y = yValue;
}
// get x coordinate
public double getX()
{
return x;
}
// get y coordinate
public double getY()
{
return y;
}
// convert point into String representation
public String toString()
{
return "[" + String.format("%.2f", x)
+ ", " + String.format("%.2f", y) + "]";
}
//Method to compare two points
public boolean equals(Point otherPoint)
{
return(x == otherPoint.x &&
y == otherPoint.y);
}
//Method to compare two points
public void makeCopy(Point otherPoint)
{
x = otherPoint.x;
y = otherPoint.y;
}
public Point getCopy()
{
Point temp = new Point();
temp.x = x;
temp.y = y;
return temp;
}
// print method
public void printPoint()
{
System.out.print("[" + String.format("%.2f", x)
+ ", " + String.format("%.2f", y) + "]");
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

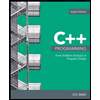
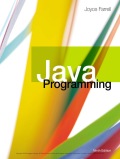
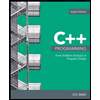
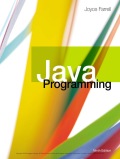