JAVA CODE For this program, you are tasked to create a class called Person which will have the following private properties: name - a string which holds the name of the person. age - an integer which holds the age of the person. gender - a character which holds the gender of the person. This could either be 'M' or 'F' Then, for this class, you need to create its getters and setters for all of its properties and its toString() method which simply returns its name, age, and gender in the format, name - age - gender. An example returned value from its toString() method is "Luce - 25 - M". Also implement its constructor with the following signature: public Person(String name, int age, char gender) Additionally, you need to implement its isMinor() method which basically returns true if its age is lesser than 18 and false otherwise. After creating the class Person, create the class Father which inherits from the Person class. This class will have a constructor with the following signature: public Father(String name, int age). You don't need to pass a gender value when creating a Father object because its gender will and should always be 'M'. This Father class will have 1 method, introduceWithStyle(int n) which accepts a positive integer variable n and will print the message "I am your father" n times with each line having an increasing number of spaces. For example, if n is 4 then the printed message would be: I am your father I am your father I am your father I am your father Finally, create another class Son which inherits from the Father class. This class should be set as final and should have two constructors: public Son(String name, int age) public Son(int age) - since no name is being passed here, set the name to "Unknown" This will have its own introduceWithStyle(int n) method which prints the message "I am your son" in decreasing number of spaces. For example, if n is 4 then the printed message would be: I am your son I am your son I am your son I am your son For this program, you are also tasked to create the main(). In the main(), all you have to do are the following: Ask the user to input the details of the Father - its name and its age, and then instantiate an object of the Father class Ask the user to input the details of Son #1 - its name and its age as well, and then instantiate an object of the Son class Ask the user to input the details of Son #2 - only its age, and then instantiate another object of the Son class
JAVA CODE
For this program, you are tasked to create a class called Person which will have the following private properties:
- name - a string which holds the name of the person.
- age - an integer which holds the age of the person.
- gender - a character which holds the gender of the person. This could either be 'M' or 'F'
Then, for this class, you need to create its getters and setters for all of its properties and its toString() method which simply returns its name, age, and gender in the format, name - age - gender. An example returned value from its toString() method is "Luce - 25 - M". Also implement its constructor with the following signature: public Person(String name, int age, char gender)
Additionally, you need to implement its isMinor() method which basically returns true if its age is lesser than 18 and false otherwise.
After creating the class Person, create the class Father which inherits from the Person class. This class will have a constructor with the following signature: public Father(String name, int age). You don't need to pass a gender value when creating a Father object because its gender will and should always be 'M'. This Father class will have 1 method, introduceWithStyle(int n) which accepts a positive integer variable n and will print the message "I am your father" n times with each line having an increasing number of spaces. For example, if n is 4 then the printed message would be:
I am your father
I am your father
I am your father
I am your father
Finally, create another class Son which inherits from the Father class. This class should be set as final and should have two constructors:
- public Son(String name, int age)
- public Son(int age) - since no name is being passed here, set the name to "Unknown"
This will have its own introduceWithStyle(int n) method which prints the message "I am your son" in decreasing number of spaces. For example, if n is 4 then the printed message would be:
I am your son
I am your son
I am your son
I am your son
For this program, you are also tasked to create the main(). In the main(), all you have to do are the following:
- Ask the user to input the details of the Father - its name and its age, and then instantiate an object of the Father class
- Ask the user to input the details of Son #1 - its name and its age as well, and then instantiate an object of the Son class
- Ask the user to input the details of Son #2 - only its age, and then instantiate another object of the Son class
Here's a sample output of the user prompts so you will be guided with the correct formatting (spelling, spacing, and capitalizations):
FATHER
Enter name: Joselito Antonio
Enter age: 57
SON #1
Enter name: Andres Antonio
Enter age: 23
SON #2
Enter age: 5

Step by step
Solved in 5 steps with 1 images

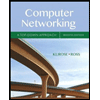
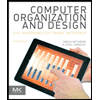
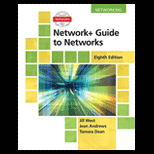
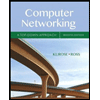
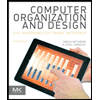
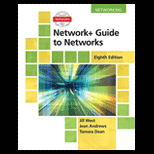
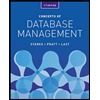
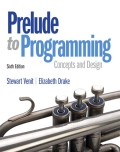
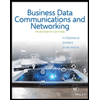