** IT'S A JAVA DEBUGGING PROBLEM! // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp >= 3) fp = fp - 3; System.out.println("Model " + plan + " is priced at only $" + pricesInThousends[fp] + ",000"); } } }
** IT'S A JAVA DEBUGGING PROBLEM! // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp >= 3) fp = fp - 3; System.out.println("Model " + plan + " is priced at only $" + pricesInThousends[fp] + ",000"); } } }
Chapter8: Arrays
Section: Chapter Questions
Problem 7PE
Related questions
Question
** IT'S A JAVA DEBUGGING PROBLEM!
// Application looks up home price
// for different floor plans
// allows upper or lowercase data entry
import java.util.*;
public class DebugEight3
{
public static void main(String[] args)
{
Scanner input = new Scanner(System.in);
String entry;
char[] floorPlans = {'A','B','C','a','b','c'}
int[] pricesInThousands = {145, 190, 235};
char plan;
int x, fp = 99;
String prompt = "Please select a floor plan\n" +
"Our floorPlanss are:\n" + "A - Augusta, a ranch\n" +
"B - Brittany, a split level\n" +
"C - Colonial, a two-story\n" +
"Enter floorPlans letter";
System.out.println(prompt);
entry = input.next();
plan = entry.charAt(1);
for(x = 0; x < floorPlans.length; ++x)
if(plan == floorPlans[x])
x = fp;
if(fp = 99)
System.out.println("Invalid floor plan code entered"));
else
{
if(fp >= 3)
fp = fp - 3;
System.out.println("Model " +
plan + " is priced at only $" +
pricesInThousends[fp] + ",000");
}
}
}
![Tasks
DebugEight3.java
>- Terminal
1 // Application looks up home price
2 // for different floor plans
3 // allows upper or lowercase data entry
sandbox $ rm -f *.class
Program runs as expected
sandbox $
sandbox $
sandbox $ javac DebugEight3.java
0.00
out of
10.00
4 import java.util.*;
5 public class DebugEight3
DebugEight3.java:11: error: ';' expected
char[] floorPlans = {'A','B','C','
6 {
Click the checkbox above to attempt this task.
7
public static void main(String[] args)
{'c' , 'פי , 'a
8
Checks
Scanner input = new Scanner(System.in);
10
String entry;
DebugEight3.java:27: error: ';' expected
System.out.println("Invalid floo
Test Case • Incomplete
>
char[] floorPlans = {'A', 'B','C','a','b','c'}
int[] pricesInThousands = {145, 190, 235};
char plan;
int x, fp = 99;
String prompt = "Please select a floor plan\n" +
"Our floorPlanss are:\n" + "A - Augusta, a ranch\n"
"B - Brittany, a split level\n" +
"C - Colonial, a two-story\n" +
11
Program walkthrough 1
12
I plan code entered"));
13
Test Case • Incomplete
>
14
DebugEight3.java:28: error: 'else' witho
ut 'if"
Program walkthrough 2
15
16
else
Test Case • Incomplete
>
17
18
Program walkthrough 3
3 errors
19
"Enter floorPlans letter";
sandbox $ java DebugEight3
System.out.println(prompt);
entry = input.next();
plan = entry.charAt(1);
for (x = 0; x < floorPlans.length; ++x)
if(plan == floorPlans[x])
x = fp;
if(fp = 99)
System.out.println("Invalid floor plan code entered"));
20
Test Case • Incomplete
>
Error: Could not find or load main class
DebugEight3
sandbox $||
21
Program walkthrough 4
22
23
Test Case • Incomplete
>
24
Program walkthrough 5
25
26
Test Case • Incomplete
>
27
Program walkthrough 6
28
else
{
if(fp >= 3)
fp = fp - 3;
System.out.println("Model " +
plan + " is priced at only $" +
pricesInThousends[fp] + " , 000");
}
29
30
31
32
33
34
35
Run checks
A Submit O%](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2c28351a-a04b-46c1-9e38-160824f161c0%2Ffaabe00a-7d8c-46d6-b5da-600ea14e7df6%2F7zoizfv_processed.png&w=3840&q=75)
Transcribed Image Text:Tasks
DebugEight3.java
>- Terminal
1 // Application looks up home price
2 // for different floor plans
3 // allows upper or lowercase data entry
sandbox $ rm -f *.class
Program runs as expected
sandbox $
sandbox $
sandbox $ javac DebugEight3.java
0.00
out of
10.00
4 import java.util.*;
5 public class DebugEight3
DebugEight3.java:11: error: ';' expected
char[] floorPlans = {'A','B','C','
6 {
Click the checkbox above to attempt this task.
7
public static void main(String[] args)
{'c' , 'פי , 'a
8
Checks
Scanner input = new Scanner(System.in);
10
String entry;
DebugEight3.java:27: error: ';' expected
System.out.println("Invalid floo
Test Case • Incomplete
>
char[] floorPlans = {'A', 'B','C','a','b','c'}
int[] pricesInThousands = {145, 190, 235};
char plan;
int x, fp = 99;
String prompt = "Please select a floor plan\n" +
"Our floorPlanss are:\n" + "A - Augusta, a ranch\n"
"B - Brittany, a split level\n" +
"C - Colonial, a two-story\n" +
11
Program walkthrough 1
12
I plan code entered"));
13
Test Case • Incomplete
>
14
DebugEight3.java:28: error: 'else' witho
ut 'if"
Program walkthrough 2
15
16
else
Test Case • Incomplete
>
17
18
Program walkthrough 3
3 errors
19
"Enter floorPlans letter";
sandbox $ java DebugEight3
System.out.println(prompt);
entry = input.next();
plan = entry.charAt(1);
for (x = 0; x < floorPlans.length; ++x)
if(plan == floorPlans[x])
x = fp;
if(fp = 99)
System.out.println("Invalid floor plan code entered"));
20
Test Case • Incomplete
>
Error: Could not find or load main class
DebugEight3
sandbox $||
21
Program walkthrough 4
22
23
Test Case • Incomplete
>
24
Program walkthrough 5
25
26
Test Case • Incomplete
>
27
Program walkthrough 6
28
else
{
if(fp >= 3)
fp = fp - 3;
System.out.println("Model " +
plan + " is priced at only $" +
pricesInThousends[fp] + " , 000");
}
29
30
31
32
33
34
35
Run checks
A Submit O%
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
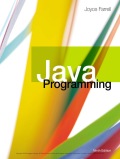
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
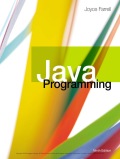
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT