Internally your program should have two Java classes: ValidatorNumeric (superclass) ValidatorString (subclass) The subclass ValidatorString extends ValidatorNumeric class, forming a hierarchy of two classes. Add a separate main class with the main() method to test all public methods in both ValidatorNumeric and ValidatorString classes. The main() method should create the necessary objects and run the user interaction as shown above. The ValidatorNumeric class is responsible for capturing user prompt and a range of valid numeric inputs. Its methods display the prompt and accept input from the user. It should provide the following public interface: // Default constructor ValidatorNumeric() // Specific constructor taking params as follows: ValidatorNumeric( String prompt, int min, int max ) // Another specific constructor taking params as follows: ValidatorNumeric( String prompt, double min, double max ) // Method that shows user prompt and gets an int from the user: public int getInt() // Method that shows user prompt and gets an int within a range. // Consider throwing InvalidArgumentException if min > max. public int getIntWithinRange() // Method that shows user prompt and gets a double from the user: public double getDouble() // Method that shows user prompt and gets a double within a range: // Consider throwing InvalidArgumentException if min > max. public double getDoubleWithinRange() Add private data attributes to store the values String prompt int min int max double min double max Optionally you can also add some getter and setter methods for the data attributes of the ValidatorNumeric class. NOTE: Make sure that all methods of the ValidatorNumeric class are non-static. Create another class named ValidatorString that extends the ValidatorNumeric. It is responsible for displaying user prompt and accepting string inputs from the user. The ValidatorString class should provide the following public interface: // Default constructor: ValidatorString() // Specific constructor taking a user prompt param: ValidatorString( String prompt ) // Specific constructor taking a user prompt and two choice params: ValidatorString( String prompt, String choiceOne, String choiceTwo ) // Method that shows user prompt and returns a non-empty string from the user: public String getRequiredString() // Method that shows user prompt and returns one of the two pre-defined choices. // Consider throwing InvalidArgumentException if any of choiceOne or choiceTwo is empty. public String getChoiceString() Add private data attributes to store the values String prompt String choiceOne String choiceTwo Optionally you can also add some getter and setter methods for the data attributes of the ValidatorString class. NOTE: Make sure that all methods of the ValidatorString class are non-static.
-
Internally your program should have two Java classes:
-
ValidatorNumeric (superclass)
-
ValidatorString (subclass)
The subclass ValidatorString extends ValidatorNumeric class, forming a hierarchy of two classes.
-
-
Add a separate main class with the main() method to test all public methods in both ValidatorNumeric and ValidatorString classes. The main() method should create the necessary objects and run the user interaction as shown above.
-
The ValidatorNumeric class is responsible for capturing user prompt and a range of valid numeric inputs. Its methods display the prompt and accept input from the user. It should provide the following public interface:
// Default constructor ValidatorNumeric() // Specific constructor taking params as follows: ValidatorNumeric( String prompt, int min, int max ) // Another specific constructor taking params as follows: ValidatorNumeric( String prompt, double min, double max ) // Method that shows user prompt and gets an int from the user: public int getInt() // Method that shows user prompt and gets an int within a range. // Consider throwing InvalidArgumentException if min > max. public int getIntWithinRange() // Method that shows user prompt and gets a double from the user: public double getDouble() // Method that shows user prompt and gets a double within a range: // Consider throwing InvalidArgumentException if min > max. public double getDoubleWithinRange() -
Add private data attributes to store the values
String prompt int min int max double min double max -
Optionally you can also add some getter and setter methods for the data attributes of the ValidatorNumeric class.
-
NOTE: Make sure that all methods of the ValidatorNumeric class are non-static.
-
Create another class named ValidatorString that extends the ValidatorNumeric. It is responsible for displaying user prompt and accepting string inputs from the user. The ValidatorString class should provide the following public interface:
// Default constructor: ValidatorString() // Specific constructor taking a user prompt param: ValidatorString( String prompt ) // Specific constructor taking a user prompt and two choice params: ValidatorString( String prompt, String choiceOne, String choiceTwo ) // Method that shows user prompt and returns a non-empty string from the user: public String getRequiredString() // Method that shows user prompt and returns one of the two pre-defined choices. // Consider throwing InvalidArgumentException if any of choiceOne or choiceTwo is empty. public String getChoiceString() -
Add private data attributes to store the values
String prompt String choiceOne String choiceTwo -
Optionally you can also add some getter and setter methods for the data attributes of the ValidatorString class.
-
NOTE: Make sure that all methods of the ValidatorString class are non-static.
-


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

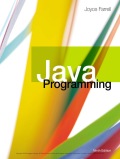
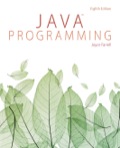
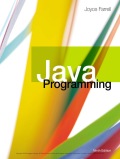
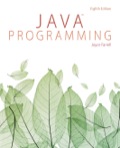