Instructions To implement the calculateBalanceWithout Monthly Deposit function, follow these steps: 1. Assume that you have a working printDetails(int yearIndex, double balance, double interestEarned This Year) function. 2. Compound the interest monthly. 3. Divide the interest rate by 100 and by 12 to calculate the monthly interest rate. 4. Make sure to keep values to at least 2 decimal places. 5. Remember that the year begins with 1. not 0. 6. After calculating the balance and interest earned for each year, call the printDetails function to print the year. year-end balance, and interest earned for that year. 7. Finally, return the final calculated end of year balance. Here is an example of the expected output for an initial investment of $100, a 10% interest rate. and 4 years: Year 1 2 3 4 Year End Balance $110.47 $122.04 $134.82 $148.94 Interest Earned $10.47 $11.57 $12.78 $14.12 Solution 1/** 2. Calculates and returns an end of year balance for given number of years 3. 4. @param initialInvestment dollar amount of initial investment 5* @param interestRate percentage of investment earned each year (annually), so a passed value of 3.5 is a rate of .035 6. @param numberOfYears number of years to calculate balance for 7. 8 @return the final calculated end of year balance 9 / 10 double calculateBalancewithouthonthlyDeposit(double initialInvestment, double interestRate, int numberOfYears) ( 11 // you may wish to change this function when you bring it into your program 12 // for now please get it to work here as specified 13 14 15 16 17 IMPORTANT TIPS: // You should assume a working printDetails(int yearIndex, double balance, double interestEarned This Year) exists // printDetails should be called after each year // Compounding should be done monthly // Make sure to keep values to at least 2 decimal places // Make sure to divide the interest rate by 100 and by 12 // Year begins with 1, not e 18 19 20 21 22 23 Example: for 100 initial, 10% interest, and 4 years /
Instructions To implement the calculateBalanceWithout Monthly Deposit function, follow these steps: 1. Assume that you have a working printDetails(int yearIndex, double balance, double interestEarned This Year) function. 2. Compound the interest monthly. 3. Divide the interest rate by 100 and by 12 to calculate the monthly interest rate. 4. Make sure to keep values to at least 2 decimal places. 5. Remember that the year begins with 1. not 0. 6. After calculating the balance and interest earned for each year, call the printDetails function to print the year. year-end balance, and interest earned for that year. 7. Finally, return the final calculated end of year balance. Here is an example of the expected output for an initial investment of $100, a 10% interest rate. and 4 years: Year 1 2 3 4 Year End Balance $110.47 $122.04 $134.82 $148.94 Interest Earned $10.47 $11.57 $12.78 $14.12 Solution 1/** 2. Calculates and returns an end of year balance for given number of years 3. 4. @param initialInvestment dollar amount of initial investment 5* @param interestRate percentage of investment earned each year (annually), so a passed value of 3.5 is a rate of .035 6. @param numberOfYears number of years to calculate balance for 7. 8 @return the final calculated end of year balance 9 / 10 double calculateBalancewithouthonthlyDeposit(double initialInvestment, double interestRate, int numberOfYears) ( 11 // you may wish to change this function when you bring it into your program 12 // for now please get it to work here as specified 13 14 15 16 17 IMPORTANT TIPS: // You should assume a working printDetails(int yearIndex, double balance, double interestEarned This Year) exists // printDetails should be called after each year // Compounding should be done monthly // Make sure to keep values to at least 2 decimal places // Make sure to divide the interest rate by 100 and by 12 // Year begins with 1, not e 18 19 20 21 22 23 Example: for 100 initial, 10% interest, and 4 years /
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter5: Control Structures Ii (repetition)
Section: Chapter Questions
Problem 20PE: When you borrow money to buy a house, a car, or for some other purpose, you repay the loan by making...
Related questions
Question
Help please

Transcribed Image Text:Instructions
To implement the calculateBalanceWithout Monthly Deposit function, follow these steps:
1. Assume that you have a working printDetails(int yearIndex, double balance,
double interest Earned This Year) function.
2. Compound the interest monthly.
3. Divide the interest rate by 100 and by 12 to calculate the monthly interest rate.
Here is an example of the expected output for an initial investment of $100, a 10% interest rate,
and 4 years:
Year
1
2
4. Make sure to keep values to at least 2 decimal places.
5. Remember that the year begins with 1, not 0.
6. After calculating the balance and interest earned for each year, call the printDetails
function to print the year, year-end balance, and interest earned for that year.
7. Finally, return the final calculated end of year balance.
3
4
Year End Balance.
$110.47
$122.04
$134.82
$148.94
Interest Earned
$10.47
$11.57
$12.78
$14.12
Solution
1 /**
2
* Calculates and returns an end of year balance for given number of years
*
3
4
* @param initialInvestment dollar amount of initial investment
5
* @param interestRate percentage of investment earned each year (annually), so a passed value of 3.5 is a rate of .035
6 * @param numberOf Years number of years to calculate balance for
7
*
8
* @return the final calculated end of year balance
9
*/
10 double calculateBalanceWithoutMonthlyDeposit (double initialInvestment, double interestRate, int numberOfYears) {
11
// you may wish to change this function when you bring it into your program
12
// for now please get it to work here as specified
13
14
15
16
17
18
19
20
21
22
23
IMPORTANT TIPS:
// You should assume a working printDetails(int yearIndex, double balance, double interestEarned This Year) exists
// printDetails should be called after each year
// Compounding should be done monthly
// Make sure to keep values to at least 2 decimal places
// Make sure to divide the interest rate by 100 and by 12
// Year begins with 1, not 0
/*
Example: for 100 initial, 10% interest, and 4 years
Language: cpp
F
L
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
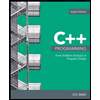
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
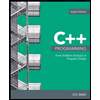
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning