#include<iostream>#include<string>class Bicycle{friend std::ostream& operator<<(std::ostream& out_ref, const Bicycle& bike_ref);public:Bicycle(const std::string& name_ref, unsigned int gear, bool motorized, double range):name_(name_ref), gear_(gear), motorized_(motorized), range_(range){current_gear_=1;};Bicycle operator++() ;Bicycle operator--(int) ;double operator<(const Bicycle& rhs_ref) const;double operator>(const Bicycle& rhs_ref) const;double operator!() const;std::string name() const { return name_;};unsigned int current_gear() const { return current_gear_;};unsigned int gear_max() const { return gear_max_;};double range() const { return range_;}bool motorized() const { return motorized_;};private:std::string name_;unsigned int gear_max_;unsigned int current_gear_;double range_;bool motorized_;}; For the operator-- a member function should be used. The function shall decrement the current gear (current_gear_) if the current gear is higher then 1. Otherwise nothing shall happen. For example the result of std::cout << (bicycle1--).current_gear() << std::endl // consol output: 2std::cout << bicycle1.current_gear() << std::endl;
#include<iostream>
#include<string>
class Bicycle
{
friend std::ostream& operator<<(std::ostream& out_ref, const Bicycle& bike_ref);
public:
Bicycle(const std::string& name_ref, unsigned int gear, bool motorized, double range):
name_(name_ref), gear_(gear), motorized_(motorized), range_(range){current_gear_=1;};
Bicycle operator++() ;
Bicycle operator--(int) ;
double operator<(const Bicycle& rhs_ref) const;
double operator>(const Bicycle& rhs_ref) const;
double operator!() const;
std::string name() const { return name_;};
unsigned int current_gear() const { return current_gear_;};
unsigned int gear_max() const { return gear_max_;};
double range() const { return range_;}
bool motorized() const { return motorized_;};
private:
std::string name_;
unsigned int gear_max_;
unsigned int current_gear_;
double range_;
bool motorized_;
};
For the operator-- a member function should be used. The function shall decrement the current gear (current_gear_) if the current gear is higher then 1. Otherwise nothing shall happen.
For example the result of
std::cout << (bicycle1--).current_gear() << std::endl // consol output: 2
std::cout << bicycle1.current_gear() << std::endl;
Unlock instant AI solutions
Tap the button
to generate a solution
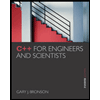
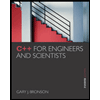