#include using namespace std; struct Triple { int row, col, value; }; class Matrix; class MatrixNode { friend class Matrix; friend istream& operator>>(istream&, Matrix&); private: MatrixNode *down, *right; bool head; union { MatrixNode *next; Triple triple; }; MatrixNode(bool, Triple*); }; MatrixNode::MatrixNode(bool b, Triple *t) { head = b; if (b) { right = down = this; } else triple = *t; }; class Matrix { friend istream& operator>>(istream&, Matrix&); public: ~Matrix(); MatrixNode* private: MatrixNode *headnode; }; Matrix::~Matrix() {// Return all nodes to the av list, which is a chain linked // via the right field. // av is a static variable pointing to the first of the av list. if (!headnode ) return; // no nodes to delete MatrixNode *x = headnode->right; headnode->right = av; av = headnode; // return headnode while (x != headnode) { // return nodes by rows MatrixNode *y = x->right; x->right = av; av = y; x = x->next; // next row } headnode = 0; } istream& operator>>(istream& is, Matrix& matrix) { // Read in a maxtix and set up its linked representation Triple s; is >> s.row >> s.col >> s.value; // matrix dimensions int p = max(s.row, s.col); // set up header node for list of header nodes matrix.headnode = new MatrixNode(false, &s); if (p == 0) { matrix.headnode->right = matrix.headnode; return is; // for supporting "cin >> mi >> mj;" } // at least one row or column MatrixNode **head = new MatrixNode* [p]; for (int i = 0; i < p; i++) head[i] = new MatrixNode(true, 0); // please continue on the next page int currentRow = 0; MatrixNode* last = head[0]; // last node in current row for (int i = 0; i < s.value; i++) // input triples { Triple t; is >> t.row >> t.col >> t.value; if (t.row > currentRow) // end of current row { last->right = head[currentRow]; // close current row currentRow = t.row; last = head[currentRow]; } // end of if last = last->right = new MatrixNode(false, &t); // link new node into row list head[t.col]->next = head[t.col]->next->down = last; // link into column list } // end of for // please continue on the next page last->right = head[currentRow]; // close last row for (int i = 0; i < s.col; i++) head[i]->next->down = head[i]; // close all column lists // link the header nodes together for (int i = 0; i < p; i++) head[i]->next = head[i + 1]; head[p-1]->next = matrix.headnode; matrix.headnode->right = head[0]; delete [] head; return is; } int main() { Matrix m, n, k; cin >> m >> n >>k; cout << "Hello world!" << endl; return 0; }
#include <iostream>
using namespace std;
struct Triple
{
int row, col, value;
};
class Matrix;
class MatrixNode
{
friend class Matrix;
friend istream& operator>>(istream&, Matrix&);
private:
MatrixNode *down, *right;
bool head;
union
{
MatrixNode *next;
Triple triple;
};
MatrixNode(bool, Triple*);
};
MatrixNode::MatrixNode(bool b, Triple *t)
{
head = b;
if (b)
{
right = down = this;
}
else triple = *t;
};
class Matrix
{
friend istream& operator>>(istream&, Matrix&);
public:
~Matrix();
MatrixNode*
private:
MatrixNode *headnode;
};
Matrix::~Matrix()
{// Return all nodes to the av list, which is a chain linked
// via the right field.
// av is a static variable pointing to the first of the av list.
if (!headnode )
return; // no nodes to delete
MatrixNode *x = headnode->right;
headnode->right = av;
av = headnode; // return headnode
while (x != headnode) { // return nodes by rows
MatrixNode *y = x->right;
x->right = av;
av = y;
x = x->next; // next row
}
headnode = 0;
}
istream& operator>>(istream& is, Matrix& matrix)
{
// Read in a maxtix and set up its linked representation
Triple s;
is >> s.row >> s.col >> s.value; // matrix dimensions
int p = max(s.row, s.col);
// set up header node for list of header nodes
matrix.headnode = new MatrixNode(false, &s);
if (p == 0)
{
matrix.headnode->right = matrix.headnode;
return is; // for supporting "cin >> mi >> mj;"
}
// at least one row or column
MatrixNode **head = new MatrixNode* [p];
for (int i = 0; i < p; i++)
head[i] = new MatrixNode(true, 0);
// please continue on the next page
int currentRow = 0;
MatrixNode* last = head[0]; // last node in current row
for (int i = 0; i < s.value; i++) // input triples
{
Triple t;
is >> t.row >> t.col >> t.value;
if (t.row > currentRow) // end of current row
{
last->right = head[currentRow]; // close current row
currentRow = t.row;
last = head[currentRow];
} // end of if
last = last->right = new MatrixNode(false, &t);
// link new node into row list
head[t.col]->next = head[t.col]->next->down = last;
// link into column list
} // end of for
// please continue on the next page
last->right = head[currentRow]; // close last row
for (int i = 0; i < s.col; i++)
head[i]->next->down = head[i]; // close all column lists
// link the header nodes together
for (int i = 0; i < p; i++)
head[i]->next = head[i + 1];
head[p-1]->next = matrix.headnode;
matrix.headnode->right = head[0];
delete [] head;
return is;
}
int main()
{
Matrix m, n, k;
cin >> m >> n >>k;
cout << "Hello world!" << endl;
return 0;
}
Based on this class, do the following tasks.
(a) Write the C++ function, operator+(const Matrix& b) const, which returns the matrix *this + b.
(b) Write the C++ function, operator*(const Matrix& b) const, which returns the matrix *this * b.
(c) Write the C++ function, operator<<(), which outputs a sparse matrix as triples (i, j, aij).
(d) Write the C++ function, Transpose(), which transpose a sparse matrix.
(e) Write and test a copy constructor for sparse matrices. What is the computing time of your copy constructor?
Note:
I have tried to code it, but the overloaded operator>> has a bug, I think it's this line "head[t.col]->next = head[t.col]->next->down = last;". for illustration I attach two pictures.



Step by step
Solved in 2 steps

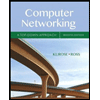
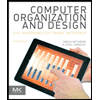
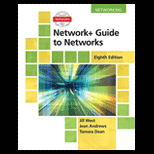
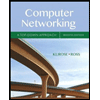
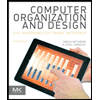
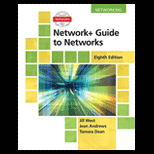
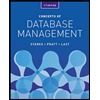
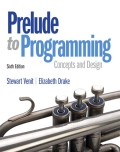
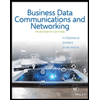