#include "pqueue.h" #include #include "string.h" #include typedef char *string; typedef double priority; //priority here is double //There are some errors to be improved. I have already highlighted out. If there is any other errors, please tell points out typedef struct node { string data; priority prior; struct node* next; } NodeT; typedef struct PriorityQueueRepr{ NodeT* top; int element; //number of element in queue }PriorityQueueRepr; pqueue pqueue_create() { pqueue pq = (pqueue)malloc(sizeof(PriorityQueueRepr)); if (pq == NULL) { return NULL; } pq->element = 0; pq->top = NULL; return pq; } void pqueue_destroy(pqueue pq) { while (!pqueue_empty(pq)) { string s = pqueue_leave(pq); free(s); } free(pq); } void pqueue_join(pqueue pq, string dat, priority prio) { NodeT* newNode = (NodeT*)malloc(sizeof(NodeT)); newNode->data = (string)malloc(strlen(dat) + 1); //error is here how to improve it newNode->prior = (priority)malloc(sizeof(prio) + 1); strcpy(newNode->data, dat); newNode->next = NULL; // If the queue is empty, add the new node as the first element. if (pq->top == NULL) { pq->top = newNode; } else { NodeT* current = pq->top; NodeT* previous = NULL; // Find the position for the new node based on its priority. //error is here while (current != NULL && strcmp(current->prior, prio) < 0) { previous = current; current = current->next; } // If the new node should be at the beginning of the queue. if (previous == NULL) { newNode->next = pq->top; pq->top = newNode; } else { newNode->next = current; previous->next = newNode; } } pq->element++; } string pqueue_leave(pqueue pq) { if (pqueue_empty(pq)) { return NULL; } NodeT *temp = pq->top; string data = temp->data; pq->top = temp->next; //error is here free(temp->prior); free(temp); pq->element--; return data; } string pqueue_peek(pqueue pq) { if (pqueue_empty(pq)) { return NULL; } return pq->top->data; } bool pqueue_empty(pqueue pq) { return pq->top == NULL; }
#include "pqueue.h"
#include <assert.h>
#include "string.h"
#include <stdlib.h>
typedef char *string;
typedef double priority; //priority here is double
//There are some errors to be improved. I have already highlighted out. If there is any other errors, please tell points out
typedef struct node {
string data;
priority prior;
struct node* next;
} NodeT;
typedef struct PriorityQueueRepr{
NodeT* top;
int element; //number of element in queue
}PriorityQueueRepr;
pqueue pqueue_create() {
pqueue pq = (pqueue)malloc(sizeof(PriorityQueueRepr));
if (pq == NULL) {
return NULL;
}
pq->element = 0;
pq->top = NULL;
return pq;
}
void pqueue_destroy(pqueue pq) {
while (!pqueue_empty(pq)) {
string s = pqueue_leave(pq);
free(s);
}
free(pq);
}
void pqueue_join(pqueue pq, string dat, priority prio) {
NodeT* newNode = (NodeT*)malloc(sizeof(NodeT));
newNode->data = (string)malloc(strlen(dat) + 1);
//error is here how to improve it
newNode->prior = (priority)malloc(sizeof(prio) + 1);
strcpy(newNode->data, dat);
newNode->next = NULL;
// If the queue is empty, add the new node as the first element.
if (pq->top == NULL) {
pq->top = newNode;
}
else {
NodeT* current = pq->top;
NodeT* previous = NULL;
// Find the position for the new node based on its priority.
//error is here
while (current != NULL && strcmp(current->prior, prio) < 0) {
previous = current;
current = current->next;
}
// If the new node should be at the beginning of the queue.
if (previous == NULL) {
newNode->next = pq->top;
pq->top = newNode;
}
else {
newNode->next = current;
previous->next = newNode;
}
}
pq->element++;
}
string pqueue_leave(pqueue pq) {
if (pqueue_empty(pq)) {
return NULL;
}
NodeT *temp = pq->top;
string data = temp->data;
pq->top = temp->next;
//error is here
free(temp->prior);
free(temp);
pq->element--;
return data;
}
string pqueue_peek(pqueue pq) {
if (pqueue_empty(pq)) {
return NULL;
}
return pq->top->data;
}
bool pqueue_empty(pqueue pq) {
return pq->top == NULL;
}

Step by step
Solved in 3 steps

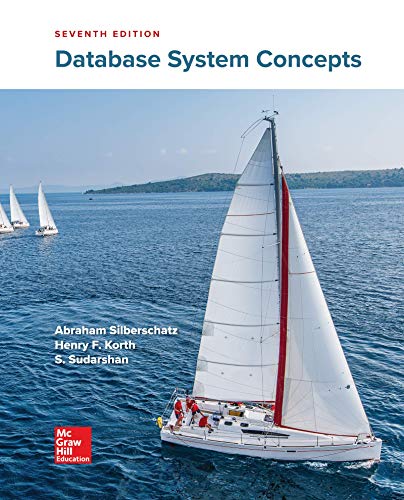
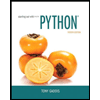
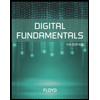
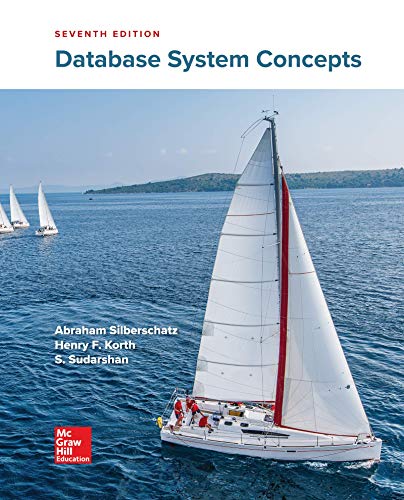
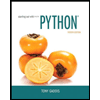
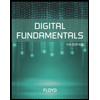
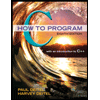
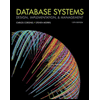
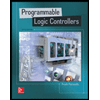