#include // Part 1 //Declare a node of character // each node has a value and a pointer that points to the next node struct charNode { __1__ val; __2__ __3__ next; }; /*This function takes the first pointer (head) and iterates through the list to print one character at a time.*/ __4__ printCharLL(__5__ __6__ curPtr) { printf("\nPrinting back the list: \n"); while(__7__ __8__ __9__) //condition to keep running the loop { printf("%c", __10__); //print the value using pointer, no spaces curPtr = __11__; //move the pointer to point to the next node in list, no spaces } printf("\n"); } // Part 2 //Declare a node of double // each node has a value and a pointer that points to the next node struct doubleNode { __12__ val; __13__ __14__ next; }; /*This function takes the first pointer (h) and iterates through the list to print one double at a time. Note the the format for double is %lf. We'll round to 3 decimal places, and there'll be tab in between each double for readability.*/ __15__ printDoubleLL(__16__ __17__ h) { printf("\nPrinting back the list: \n"); while(__18__ __19__ __20__) { printf("%.3lf\t", __21__); //print the value using pointer, no spaces h = __22__; //move the pointer to point to the next node in list, no spaces } printf("\n"); } /*This function takes the first pointer (head) and iterates through the list to get the one double at a time from the user, and save it to our list Note the the format for double is %lf. */ __23__ scanDoubleLL(__24__ __25__ head) { printf("Please fill in 4 values for our linked list.\n"); while(__26__ __27__ __28__) { printf("Enter a double: "); scanf("%lf", & __29__);//get the value from user using pointer, no space head = __30__;//move the pointer to point to the next node in list, no spaces } } int main() { //PART 1 //Create 5 character nodes, and link a head pointer to first node. __31__ __32__ cn1, cn2, cn3, cn4, cn5; //nodes __33__ __34__ head = __35__; //head pointer points to first node cn1, no spaces //Assign values to all nodes to make "Hello", no spaces __36__ = __37__; //value for cn1. __38__ = __39__; //value for cn2. __40__ = __41__; //value for cn3. __42__ = __43__; //value for cn4. __44__ = __45__; //value for cn5. //Link all nodes, no spaces __46__ = __47__; //make a link from cn1 to cn2 __48__ = __49__; //make a link from cn2 to cn3 __50__ = __51__; //make a link from cn3 to cn4 __52__ = __53__; //make a link from cn4 to cn5 __54__ = __55__; //make a link to show cn5 is the end //Call function printCharLL() to print Hello printCharLL(__56__); //PART 2 //Create 4 double nodes, and link the a head pointer to first node. __57__ __58__ dn1, dn2, dn3, dn4; //nodes __59__ __60__ head2 = &dn1; //head pointer points to first node dn1, no spaces //Link all nodes first so that we don't have to ask the user individually // if we don't link first, we'll have to ask value for each node's value manually __61__ = __62__; //make a link from dn1 to dn2 __63__ = __64__; //make a link from dn2 to dn3 __65__ = __66__; //make a link from dn3 to dn4 __67__ = __68__; //make a link to show dn4 is the end //Get values from the user scanDoubleLL(__69__); //Call function printCharLL() to print Hello printDoubleLL(__70__); return 0
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
Please fill in the blanks for C.
// Singly Linked List
#include<stdio.h>
// Part 1
//Declare a node of character
// each node has a value and a pointer that points to the next node
struct charNode
{
__1__ val;
__2__ __3__ next;
};
/*This function takes the first pointer (head) and iterates through the list
to print one character at a time.*/
__4__ printCharLL(__5__ __6__ curPtr)
{
printf("\nPrinting back the list: \n");
while(__7__ __8__ __9__) //condition to keep running the loop
{
printf("%c", __10__); //print the value using pointer, no spaces
curPtr = __11__; //move the pointer to point to the next node in list, no spaces
}
printf("\n");
}
// Part 2
//Declare a node of double
// each node has a value and a pointer that points to the next node
struct doubleNode
{
__12__ val;
__13__ __14__ next;
};
/*This function takes the first pointer (h) and iterates through the list
to print one double at a time.
Note the the format for double is %lf. We'll round to 3 decimal places,
and there'll be tab in between each double for readability.*/
__15__ printDoubleLL(__16__ __17__ h)
{
printf("\nPrinting back the list: \n");
while(__18__ __19__ __20__)
{
printf("%.3lf\t", __21__); //print the value using pointer, no spaces
h = __22__; //move the pointer to point to the next node in list, no spaces
}
printf("\n");
}
/*This function takes the first pointer (head) and iterates through the list
to get the one double at a time from the user, and save it to our list
Note the the format for double is %lf. */
__23__ scanDoubleLL(__24__ __25__ head)
{
printf("Please fill in 4 values for our linked list.\n");
while(__26__ __27__ __28__)
{
printf("Enter a double: ");
scanf("%lf", & __29__);//get the value from user using pointer, no space
head = __30__;//move the pointer to point to the next node in list, no spaces
}
}
int main()
{
//PART 1
//Create 5 character nodes, and link a head pointer to first node.
__31__ __32__ cn1, cn2, cn3, cn4, cn5; //nodes
__33__ __34__ head = __35__; //head pointer points to first node cn1, no spaces
//Assign values to all nodes to make "Hello", no spaces
__36__ = __37__; //value for cn1.
__38__ = __39__; //value for cn2.
__40__ = __41__; //value for cn3.
__42__ = __43__; //value for cn4.
__44__ = __45__; //value for cn5.
//Link all nodes, no spaces
__46__ = __47__; //make a link from cn1 to cn2
__48__ = __49__; //make a link from cn2 to cn3
__50__ = __51__; //make a link from cn3 to cn4
__52__ = __53__; //make a link from cn4 to cn5
__54__ = __55__; //make a link to show cn5 is the end
//Call function printCharLL() to print Hello
printCharLL(__56__);
//PART 2
//Create 4 double nodes, and link the a head pointer to first node.
__57__ __58__ dn1, dn2, dn3, dn4; //nodes
__59__ __60__ head2 = &dn1; //head pointer points to first node dn1, no spaces
//Link all nodes first so that we don't have to ask the user individually
// if we don't link first, we'll have to ask value for each node's value manually
__61__ = __62__; //make a link from dn1 to dn2
__63__ = __64__; //make a link from dn2 to dn3
__65__ = __66__; //make a link from dn3 to dn4
__67__ = __68__; //make a link to show dn4 is the end
//Get values from the user
scanDoubleLL(__69__);
//Call function printCharLL() to print Hello
printDoubleLL(__70__);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

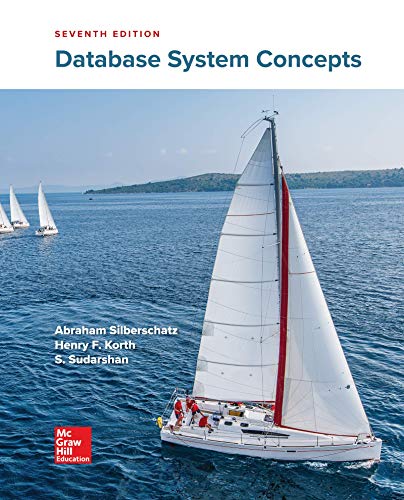
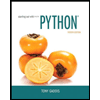
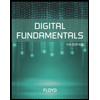
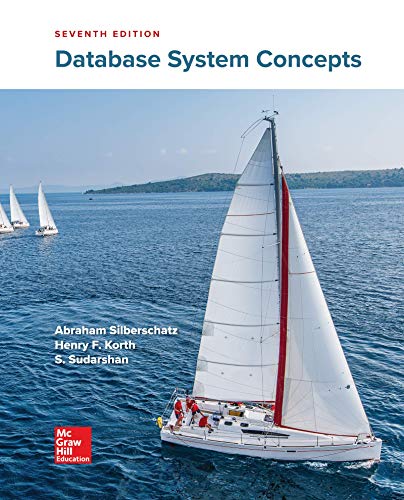
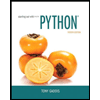
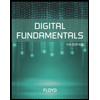
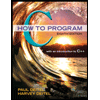
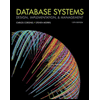
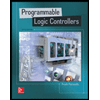