In Chapter 10, the class clockType was designed to implement the time of day in a program. Certain applications, in addition to hours, minutes, and seconds, might require you to store the time zone. Derive the class extClockType from the class clockType by adding a member variable to store the time zone called timeZone. Add the necessary member functions and constructors to make the class functional. Also, write the definitions of the member functions and the constructors. Finally, write a test program to test your class. Codes Given: clockType.h & clockTypelmp.cpp clockType.h //clockType.h, the specification file for the class clockType #ifndef H_ClockType #define H_ClockType class clockType { public: void setTime(int hours, int minutes, int seconds); //Function to set the time. //The time is set according to the parameters. //Postcondition: hr = hours; min = minutes; // sec = seconds // The function checks whether the values of // hours, minutes, and seconds are valid. If a // value is invalid, the default value 0 is // assigned. void getTime(int& hours, int& minutes, int& seconds) const; //Function to return the time. //Postcondition: hours = hr; minutes = min; // seconds = sec void printTime() const; //Function to print the time. //Postcondition: The time is printed in the form // hh:mm:ss. void incrementSeconds(); //Function to increment the time by one second. //Postcondition: The time is incremented by one // second. // If the before-increment time is 23:59:59, the // time is reset to 00:00:00. void incrementMinutes(); //Function to increment the time by one minute. //Postcondition: The time is incremented by one // minute. // If the before-increment time is 23:59:53, // the time is reset to 00:00:53. void incrementHours(); //Function to increment the time by one hour. //Postcondition: The time is incremented by one // hour. // If the before-increment time is 23:45:53, the // time is reset to 00:45:53. bool equalTime(const clockType& otherClock) const; //Function to compare the two times. //Postcondition: Returns true if this time is // equal to otherClock; otherwise, // returns false. clockType(int hours, int minutes, int seconds); //constructor with parameters //The time is set according to the parameters. //Postcondition: hr = hours; min = minutes; // sec = seconds // The constructor checks whether the values of // hours, minutes, and seconds are valid. If a // value is invalid, the default value 0 is // assigned. clockType(); //default constructor with parameters //The time is set to 00:00:00. //Postcondition: hr = 0; min = 0; sec = 0 private: int hr; //variable to store the hours int min; //variable to store the minutes int sec; //variable to store the seconds }; #endif clockTypelmp.cpp //Implementation File for the class clockType #include #include "clockType.h" using namespace std; void clockType::setTime(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) hr = hours; else hr = 0; if (0 <= minutes && minutes < 60) min = minutes; else min = 0; if (0 <= seconds && seconds < 60) sec = seconds; else sec = 0; } void clockType::getTime(int& hours, int& minutes, int& seconds) const { hours = hr; minutes = min; seconds = sec; } void clockType::incrementHours() { hr++; if (hr > 23) hr = 0; } void clockType::incrementMinutes() { min++; if (min > 59) { min = 0; incrementHours(); } } void clockType::incrementSeconds() { sec++; if (sec > 59) { sec = 0; incrementMinutes(); } } void clockType::printTime() const { if (hr < 10) cout << "0"; cout << hr << ":"; if (min < 10) cout << "0"; cout << min << ":"; if (sec < 10) cout << "0"; cout << sec; } bool clockType::equalTime(const clockType& otherClock) const { return (hr == otherClock.hr && min == otherClock.min && sec == otherClock.sec); } clockType::clockType(int hours, int minutes, int seconds) { if (0 <= hours && hours < 24) hr = hours; else hr = 0; if (0 <= minutes && minutes < 60) min = minutes; else min = 0; if (0 <= seconds && seconds < 60) sec = seconds; else sec = 0; } clockType::clockType() //default constructor { hr = 0; min = 0; sec = 0; } Need to come up with extClockType.h , extClockTypelmp.cpp , and main.cpp
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
In Chapter 10, the class clockType was designed to implement the time of day in a program. Certain applications, in addition to hours, minutes, and seconds, might require you to store the time zone. Derive the class extClockType from the class clockType by adding a member variable to store the time zone called timeZone. Add the necessary member functions and constructors to make the class functional. Also, write the definitions of the member functions and the constructors. Finally, write a test program to test your class.
Codes Given: clockType.h & clockTypelmp.cpp
clockType.h

Step by step
Solved in 3 steps

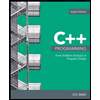
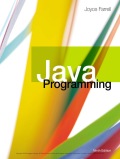
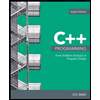
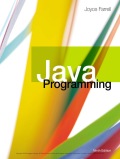