In the below code, if the initial or final character is a space, the answer is wrong. can you please help.
In the below code, if the initial or final character is a space, the answer is wrong. can you please help.
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int count_tokens(const char* str) {
int count = 0;
int invalidToken = 0; // set false
for(int i = 0; i <= strlen(str); i++) {
if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space
if(invalidToken) {
invalidToken = 0; // set false
continue;
}
else {
count++; // increment count only if token was not invalid
continue;
}
}
if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter
invalidToken = 1; // set true
}
}
return count;
}
char** split_tokens(const char* str) {
char **tokenArr;
tokenArr = (char **) malloc(count_tokens(str) * sizeof(char*)); /*use count_tokes() to determine the length*/
int invalidToken = 0; // set false
int startIndex = 0;
int arrayIndex = 0;
for(int i = 0; i <= strlen(str); i++) {
if( i == strlen(str) || str[i] == 32){ // if past end of string, or if space
if(invalidToken) {
invalidToken = 0; // set false
startIndex = i + 1;
continue;
}
else {
int lengthOfTokenFound = i - startIndex;
char *tokenFound;
tokenFound = (char*) malloc((lengthOfTokenFound+1)*sizeof(char)); /*+1 for '\0' character */
for(int i = 0; i < lengthOfTokenFound; i++) {
tokenFound[i] = str[startIndex+i];
}
tokenArr[arrayIndex] = tokenFound;
arrayIndex++;
startIndex = i + 1;
continue;
}
}
if( !(str[i] >= 97 && str[i] <= 122) ) { // if not lowercase letter
invalidToken = 1; // set true
}
}
return tokenArr;
}
void printArrayOfStrings(char ** arr, int length) {
printf("[");
for(int i = 0; i < length; i++) {
printf("'%s'", arr[i]);
if(i < length-1) {
printf(", ");
}
}
printf("]\n\n");
}
void main() {
char myStr1[] = "abc EFaG hi";
int count1 = count_tokens(myStr1);
printf("String '%s' has %d tokens.\n",myStr1, count1);
char** tokArr1 = split_tokens(myStr1);
printArrayOfStrings(tokArr1, count1);
char myStr2[] = "ab 12 ef hi";
int count2 = count_tokens(myStr2);
printf("String '%s' has %d tokens.\n",myStr2, count2);
char** tokArr2 = split_tokens(myStr2);
printArrayOfStrings(tokArr2, count2);
char myStr3[] = "ab12ef+";
int count3 = count_tokens(myStr3);
printf("String '%s' has %d tokens.\n",myStr3, count3);
char** tokArr3 = split_tokens(myStr3);
printArrayOfStrings(tokArr3, count3);
}

Step by step
Solved in 2 steps with 1 images

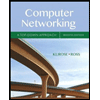
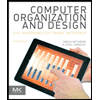
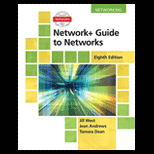
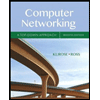
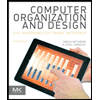
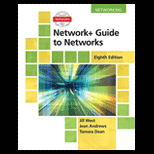
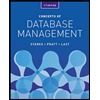
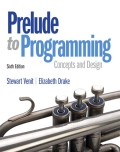
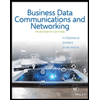