IN SCALA COULD YOU COMPLETE THE FOLLOWING FUNCTIONS: // Regular Expressions abstract class Rexp case object ZERO extends Rexp case object ONE extends Rexp case class CHAR(c: Char) extends Rexp case class ALTs(rs: List[Rexp]) extends Rexp // alternatives case class SEQ(r1: Rexp, r2: Rexp) extends Rexp // sequence case class STAR(r: Rexp) extends Rexp // star // some convenience for typing regular expressions //the usual binary choice can be defined in terms of ALTs def ALT(r1: Rexp, r2: Rexp) = ALTs(List(r1, r2)) import scala.language.implicitConversions import scala.language.reflectiveCalls def charlist2rexp(s: List[Char]): Rexp = s match { case Nil => ONE case c::Nil => CHAR(c) case c::s => SEQ(CHAR(c), charlist2rexp(s)) } implicit def string2rexp(s: String): Rexp = charlist2rexp(s.toList) implicit def RexpOps (r: Rexp) = new { def | (s: Rexp) = ALT(r, s) def % = STAR(r) def ~ (s: Rexp) = SEQ(r, s) } implicit def stringOps (s: String) = new { def | (r: Rexp) = ALT(s, r) def | (r: String) = ALT(s, r) def % = STAR(s) def ~ (r: Rexp) = SEQ(s, r) def ~ (r: String) = SEQ(s, r) } // (1) Complete the function nullable according to // the definition given in the coursework; this // function checks whether a regular expression // can match the empty string and Returns a boolean // accordingly. def nullable (r: Rexp) : Boolean = ??? // (2) Complete the function der according to // the definition given in the coursework; this // function calculates the derivative of a // regular expression w.r.t. a character. def der (c: Char, r: Rexp) : Rexp = ??? // (3) Implement the flatten function flts. It // deletes 0s from a list of regular expressions // and also 'spills out', or flattens, nested // ALTernativeS. def flts(rs: List[Rexp]) : List[Rexp] = ??? // (4) Complete the simp function according to // the specification given in the coursework description; // this function simplifies a regular expression from // the inside out, like you would simplify arithmetic // expressions; however it does not simplify inside // STAR-regular expressions. Use the _.distinct and // flts functions. def simp(r: Rexp) : Rexp = ??? // (5) Complete the two functions below; the first // calculates the derivative w.r.t. a string; the second // is the regular expression matcher taking a regular // expression and a string and checks whether the // string matches the regular expression def ders (s: List[Char], r: Rexp) : Rexp = ??? def matcher(r: Rexp, s: String): Boolean = ??? // (6) Complete the size function for regular // expressions according to the specification // given in the coursework. def size(r: Rexp): Int = ???
IN SCALA COULD YOU COMPLETE THE FOLLOWING FUNCTIONS:
// Regular Expressions
abstract class Rexp
case object ZERO extends Rexp
case object ONE extends Rexp
case class CHAR(c: Char) extends Rexp
case class ALTs(rs: List[Rexp]) extends Rexp // alternatives
case class SEQ(r1: Rexp, r2: Rexp) extends Rexp // sequence
case class STAR(r: Rexp) extends Rexp // star
// some convenience for typing regular expressions
//the usual binary choice can be defined in terms of ALTs
def ALT(r1: Rexp, r2: Rexp) = ALTs(List(r1, r2))
import scala.language.implicitConversions
import scala.language.reflectiveCalls
def charlist2rexp(s: List[Char]): Rexp = s match {
case Nil => ONE
case c::Nil => CHAR(c)
case c::s => SEQ(CHAR(c), charlist2rexp(s))
}
implicit def string2rexp(s: String): Rexp = charlist2rexp(s.toList)
implicit def RexpOps (r: Rexp) = new {
def | (s: Rexp) = ALT(r, s)
def % = STAR(r)
def ~ (s: Rexp) = SEQ(r, s)
}
implicit def stringOps (s: String) = new {
def | (r: Rexp) = ALT(s, r)
def | (r: String) = ALT(s, r)
def % = STAR(s)
def ~ (r: Rexp) = SEQ(s, r)
def ~ (r: String) = SEQ(s, r)
}
// (1) Complete the function nullable according to
// the definition given in the coursework; this
// function checks whether a regular expression
// can match the empty string and Returns a boolean
// accordingly.
def nullable (r: Rexp) : Boolean = ???
// (2) Complete the function der according to
// the definition given in the coursework; this
// function calculates the derivative of a
// regular expression w.r.t. a character.
def der (c: Char, r: Rexp) : Rexp = ???
// (3) Implement the flatten function flts. It
// deletes 0s from a list of regular expressions
// and also 'spills out', or flattens, nested
// ALTernativeS.
def flts(rs: List[Rexp]) : List[Rexp] = ???
// (4) Complete the simp function according to
// the specification given in the coursework description;
// this function simplifies a regular expression from
// the inside out, like you would simplify arithmetic
// expressions; however it does not simplify inside
// STAR-regular expressions. Use the _.distinct and
// flts functions.
def simp(r: Rexp) : Rexp = ???
// (5) Complete the two functions below; the first
// calculates the derivative w.r.t. a string; the second
// is the regular expression matcher taking a regular
// expression and a string and checks whether the
// string matches the regular expression
def ders (s: List[Char], r: Rexp) : Rexp = ???
def matcher(r: Rexp, s: String): Boolean = ???
// (6) Complete the size function for regular
// expressions according to the specification
// given in the coursework.
def size(r: Rexp): Int = ???

Trending now
This is a popular solution!
Step by step
Solved in 6 steps

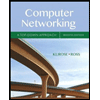
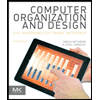
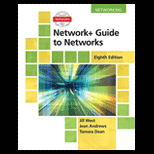
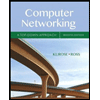
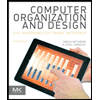
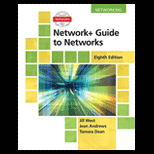
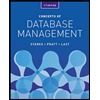
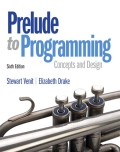
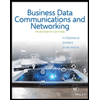