in Java Part 1 : Start by creating a new class called MyPoint that can be initialized with either of these constructors: MyPoint p1 = new MyPoint(); MyPoint p2 = new MyPoint(2.0, 4.0); The two arguments given to the constructor are x (2.0) and y (4.0) co-ordinates. Don’t forget to include getters and setters as these should be private instance variables! Part 2 : Create a toString() instance method that prints out the co-ordinates of the point. Add two instance methods that return the Euclidean distance to another point, or to another (x, y) coordinate. Example: double distance = p2.distance(p1); The Euclidean distance between two points is: d=√x^2 + y^2 You will need to make use of methods from the Math package: https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/Math.html Test your constructors and the new methods. Part 3 : Create a class method, contains(MyPoint[] points, MyPoint p), that checks if the point p is in the array points and returns a boolean. Finally, add a class method horizontal(MyPoint[] points) that determines if an array of points is in a horizontal line and returns a boolean.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
in Java
Part 1 : Start by creating a new class called MyPoint that can be initialized with either of these constructors:
MyPoint p1 = new MyPoint();
MyPoint p2 = new MyPoint(2.0, 4.0);
The two arguments given to the constructor are x (2.0) and y (4.0) co-ordinates. Don’t forget to include getters and setters as these should be private instance variables!
Part 2 :
Create a toString() instance method that prints out the co-ordinates of the point. Add two instance methods that return the Euclidean distance to another point, or to another (x, y) coordinate. Example:
double distance = p2.distance(p1);
The Euclidean distance between two points is: d=√x^2 + y^2
You will need to make use of methods from the Math package: https://docs.oracle.com/en/java/javase/17/docs/api/java.base/java/lang/Math.html
Test your constructors and the new methods.
Part 3 : Create a class method,
contains(MyPoint[] points, MyPoint p), that checks if the point p is in the array points and returns a boolean.
Finally, add a class method horizontal(MyPoint[] points) that determines if an array of points is in a horizontal line and returns a boolean.

Step by step
Solved in 3 steps with 2 images

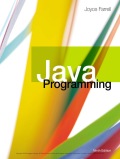
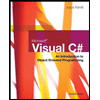
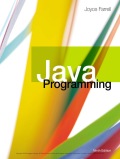
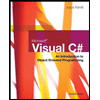