In-Class Programming 12 (Section 1) Create the following program to build a car class (car_class.py) and a program (InClassProgramming 12-1.py) to interact with this class: > Car class: o Three private data attributes: make, model, year (initialized with user value when creating object) One private data attribute speed initialized with zero (0) o • Mutator method for speed named accelerate, parameter accelerateSpeed, increment speed using parameter value o Mutator method for speed named brake, parameter brakeSpeed, decrement speed using parameter value o Accessor method get_speed to return the value of private data attribute speed o_str_method to produce output as shown below > Program: o Three user inputs as shown below, store in variables make, model, and year Create instance of car object and store in variable mycar o o Get user input of acceleration in mph and store in variable acceleration o Loop five times, within loop: . Call accelerate mutator of mycar and pass variable acceleration as argument Display speed by using get_speed accessor method of mycar (see output below) Similar to the previous user input and loop, we need to brake now because we are way too fast Get user input of braking speed in mph and store in variable braking o Loop five times, within loop: . • o At the end of your program, call_str_method and display its content Call brake mutator of mycar and pass variable braking as argument Display speed by using get_speed accessor method of mycar (see output below) Upload the following files to Canvas: > Screenshot of the executed code in command line terminal window (either pasted into a Word document or as an image) > Text files of your code named InClassProgramming12-1.py (put your name and section as comments at the top of file) Notes: > Upload the files (screenshot(s) and Python code as text file(s)) > Your code should contain some meaningful comments Your code should be well organized and formatted > Command Prompt temppypython teclasgrating12-1.p Please enter car's mate (examp * the aucaloration rate in I us drive, accelerate 24 Nissan): Honda le: Altino): civit too fast, need to brak... brake rate in miles per her: Car information: Model: Civic Year: 2001 Current 2000): 2001 par hours 12
In-Class Programming 12 (Section 1) Create the following program to build a car class (car_class.py) and a program (InClassProgramming 12-1.py) to interact with this class: > Car class: o Three private data attributes: make, model, year (initialized with user value when creating object) One private data attribute speed initialized with zero (0) o • Mutator method for speed named accelerate, parameter accelerateSpeed, increment speed using parameter value o Mutator method for speed named brake, parameter brakeSpeed, decrement speed using parameter value o Accessor method get_speed to return the value of private data attribute speed o_str_method to produce output as shown below > Program: o Three user inputs as shown below, store in variables make, model, and year Create instance of car object and store in variable mycar o o Get user input of acceleration in mph and store in variable acceleration o Loop five times, within loop: . Call accelerate mutator of mycar and pass variable acceleration as argument Display speed by using get_speed accessor method of mycar (see output below) Similar to the previous user input and loop, we need to brake now because we are way too fast Get user input of braking speed in mph and store in variable braking o Loop five times, within loop: . • o At the end of your program, call_str_method and display its content Call brake mutator of mycar and pass variable braking as argument Display speed by using get_speed accessor method of mycar (see output below) Upload the following files to Canvas: > Screenshot of the executed code in command line terminal window (either pasted into a Word document or as an image) > Text files of your code named InClassProgramming12-1.py (put your name and section as comments at the top of file) Notes: > Upload the files (screenshot(s) and Python code as text file(s)) > Your code should contain some meaningful comments Your code should be well organized and formatted > Command Prompt temppypython teclasgrating12-1.p Please enter car's mate (examp * the aucaloration rate in I us drive, accelerate 24 Nissan): Honda le: Altino): civit too fast, need to brak... brake rate in miles per her: Car information: Model: Civic Year: 2001 Current 2000): 2001 par hours 12
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter11: Inheritance And Composition
Section: Chapter Questions
Problem 5PE: Using classes, design an online address book to keep track of the names, addresses, phone numbers,...
Related questions
Question
dsadadadada
python please

Transcribed Image Text:In-Class Programming 12 (Section 1)
Create the following program to build a car class (car_class.py) and a program (InClassProgramming 12-1.py) to interact
with this class:
> Car class:
o Three private data attributes: make, model, year (initialized with user value when creating object)
One private data attribute speed initialized with zero (0)
o
Mutator method for speed named accelerate, parameter accelerateSpeed, increment speed using parameter
value
o
Mutator method for speed named brake, parameter brakeSpeed, decrement speed using parameter value
o Accessor method get_speed to return the value of private data attribute speed
o_str_method
to produce output as shown below
> Program:
o Three user inputs as shown below, store in variables make, model, and year
Create instance of car object and store in variable mycar
o
o
Get user input of acceleration in mph and store in variable acceleration
Notes:
o Loop five times, within loop:
o
o
o
•
Call accelerate mutator of my car and pass variable acceleration as argument
• Display speed by using get_speed accessor method of mycar (see output below)
Similar to the previous user input and loop, we need to brake now because we are way too fast
Get user input of braking speed in mph and store in variable braking
Loop five times, within loop:
•
Call brake mutator of my car and pass variable braking as argument
• Display speed by using get_speed accessor method of mycar (see output below)
o At the end of your program, call_str_method and display its content
Upload the following files to Canvas:
> Screenshot of the executed code in command line terminal
window (either pasted into a Word document or as an
image)
Text files of your code named InClassProgramming12-1.py
(put your name and section as comments at the top of file)
Upload the files (screenshot(s) and Python code as text
file(s))
Your code should contain some meaningful comments
➤Your code should be well organized and formatted
Command Prompt
comp\propython InclacsProgramming12-1.py
Please enter your car's make (example: Nissan): Honda
Please enter your car's nodel (example: Altima): civic
Please enter your car's year (example 2008): 2001
Enter the acceleration rate in miles per hour: 12
Let us drive, accelerate
12
Speed
Speed 24
Speed 16
Speed-
49
-ca
--Way too fast, need to prake
Enter the brake rate in miles per hour: 9
Speed 51
Speed 42
Speed-
13
Speed 24
Speed 15
Car information:
Make: Honda
Model: Civic Year: 2001
current speed 15
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
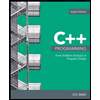
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
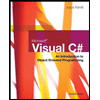
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
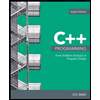
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
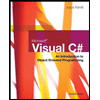
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,