In C++ Plz LAB: Grocery shopping list (linked list: inserting at the end of a list) Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts ItemNode.h Default Code: #include #include using namespace std; class ItemNode { private: string item; ItemNode* nextNodeRef; public: // Constructor ItemNode() { item = ""; nextNodeRef = NULL; } // Constructor ItemNode(string itemInit) { this->item = itemInit; this->nextNodeRef = NULL; } // Constructor ItemNode(string itemInit, ItemNode *nextLoc) { this->item = itemInit; this->nextNodeRef = nextLoc; } // Insert node after this node. void InsertAfter(ItemNode &nodeLoc) { ItemNode* tmpNext; tmpNext = this->nextNodeRef; this->nextNodeRef = &nodeLoc; nodeLoc.nextNodeRef = tmpNext; } // TODO: Define InsertAtEnd() function that inserts a node // to the end of the linked list // Get location pointed by nextNodeRef ItemNode* GetNext() { return this->nextNodeRef; } void PrintNodeData() { cout << this->item << endl; } };
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
In C++ Plz
LAB: Grocery shopping list (linked list: inserting at the end of a list)
Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Ex. if the input is:
4 Kale Lettuce Carrots Peanuts
where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list.
The output is:
Kale Lettuce Carrots Peanuts
ItemNode.h Default Code:
#include <iostream>
#include <string>
using namespace std;
class ItemNode {
private:
string item;
ItemNode* nextNodeRef;
public:
// Constructor
ItemNode() {
item = "";
nextNodeRef = NULL;
}
// Constructor
ItemNode(string itemInit) {
this->item = itemInit;
this->nextNodeRef = NULL;
}
// Constructor
ItemNode(string itemInit, ItemNode *nextLoc) {
this->item = itemInit;
this->nextNodeRef = nextLoc;
}
// Insert node after this node.
void InsertAfter(ItemNode &nodeLoc) {
ItemNode* tmpNext;
tmpNext = this->nextNodeRef;
this->nextNodeRef = &nodeLoc;
nodeLoc.nextNodeRef = tmpNext;
}
// TODO: Define InsertAtEnd() function that inserts a node
// to the end of the linked list
// Get location pointed by nextNodeRef
ItemNode* GetNext() {
return this->nextNodeRef;
}
void PrintNodeData() {
cout << this->item << endl;
}
};

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

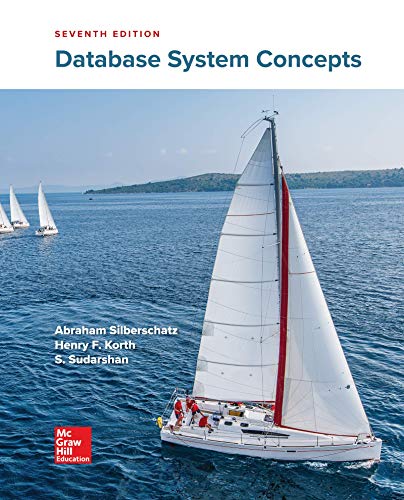
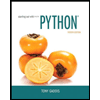
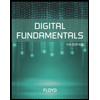
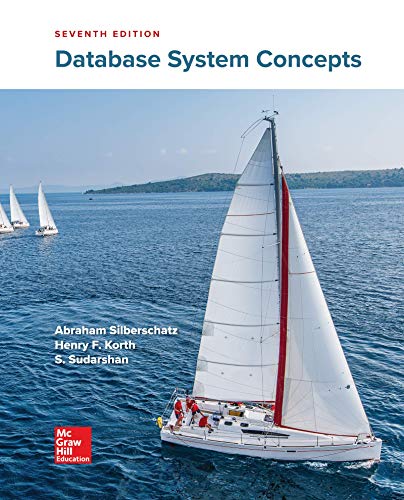
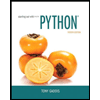
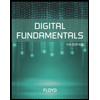
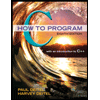
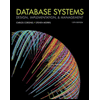
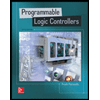