in c# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book. The Corner’s Turn() method should display You turn corners to go around the block. The Pancake's Turn() method should display You turn a pancake when it's done on one side. The Leaf's Turn() method should display A leaf turns colors in the fall. i keep getting the errors and my code is in the pics Unit Test Incomplete Page class defined Build Status Build Failed Build Outpu tCompilation failed: 3 error(s), 0 warnings NtTest5f1df6cd.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest5f1df6cd.cs(23,7): error CS0246: The type or namespace name `Page' could not be found. Are you missing `TurningDemo' using directive? NtTest5f1df6cd.cs(24,7): error CS0841: A local variable `page' cannot be used before it is declared Test Contents [TestFixture] public class PageClassTest { [Test] public void PageTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Page)), "`Page` class should implement `IRecoverable` class"); } [Test] public void PageTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Page page = new Page(); page.Turn(); string expected = "You turn a page in a book"; Assert.AreEqual(expected, sw.ToString().Trim()); } } } Unit Test Incomplete Leaf class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTeste0f8fa97.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTeste0f8fa97.cs(23,7): error CS0246: The type or namespace name `Leaf' could not be found. Are you missing `TurningDemo' using directive? NtTeste0f8fa97.cs(24,7): error CS0841: A local variable `leaf' cannot be used before it is declared Test Contents [TestFixture] public class LeafClassTest { [Test] public void LeafTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Leaf)), "`Leaf` class should implement `IRecoverable` class"); } [Test] public void LeafTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Leaf leaf = new Leaf(); leaf.Turn(); string expected = "A leaf turns colors in the fall"; Assert.AreEqual(expected, sw.ToString().Trim()); } } } Unit Test Incomplete Pancake class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTest68bcf81d.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest68bcf81d.cs(23,7): error CS0246: The type or namespace name `Pancake' could not be found. Are you missing `TurningDemo' using directive? NtTest68bcf81d.cs(24,7): error CS0841: A local variable `pancake' cannot be used before it is declared Test Contents [TestFixture] public class PancakeClassTest { [Test] public void PancakeTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Pancake)), "`Pancake` class should implement `IRecoverable` class"); } [Test] public void PancakeTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Pancake pancake = new Pancake(); pancake.Turn(); string expected = "You turn a pancake when it's done on one side"; Assert.AreEqual(expected, sw.ToString().Trim()); } } } Unit Test Incomplete Corner class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTest014d6109.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest014d6109.cs(23,7): error CS0246: The type or namespace name `Corner' could not be found. Are you missing `TurningDemo' using directive? NtTest014d6109.cs(24,7): error CS0841: A local variable `corner' cannot be used before it is declared Test Contents [TestFixture] public class CornerClassTest { [Test] public void CornerTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Corner)), "`Corner` class should implement `IRecoverable` class"); } [Test] public void CornerTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Corner corner = new Corner(); corner.Turn(); string expected = "You turn corners to go around the block"; Assert.AreEqual(expected, sw.ToString().Trim()); } } }
in c# i need to Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf. Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable. Create each class’s Turn() method to display an appropriate message. For example: The Page’s Turn() method should display You turn a page in a book. The Corner’s Turn() method should display You turn corners to go around the block. The Pancake's Turn() method should display You turn a pancake when it's done on one side. The Leaf's Turn() method should display A leaf turns colors in the fall. i keep getting the errors and my code is in the pics Unit Test Incomplete Page class defined Build Status Build Failed Build Outpu tCompilation failed: 3 error(s), 0 warnings NtTest5f1df6cd.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest5f1df6cd.cs(23,7): error CS0246: The type or namespace name `Page' could not be found. Are you missing `TurningDemo' using directive? NtTest5f1df6cd.cs(24,7): error CS0841: A local variable `page' cannot be used before it is declared Test Contents [TestFixture] public class PageClassTest { [Test] public void PageTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Page)), "`Page` class should implement `IRecoverable` class"); } [Test] public void PageTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Page page = new Page(); page.Turn(); string expected = "You turn a page in a book"; Assert.AreEqual(expected, sw.ToString().Trim()); } } } Unit Test Incomplete Leaf class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTeste0f8fa97.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTeste0f8fa97.cs(23,7): error CS0246: The type or namespace name `Leaf' could not be found. Are you missing `TurningDemo' using directive? NtTeste0f8fa97.cs(24,7): error CS0841: A local variable `leaf' cannot be used before it is declared Test Contents [TestFixture] public class LeafClassTest { [Test] public void LeafTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Leaf)), "`Leaf` class should implement `IRecoverable` class"); } [Test] public void LeafTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Leaf leaf = new Leaf(); leaf.Turn(); string expected = "A leaf turns colors in the fall"; Assert.AreEqual(expected, sw.ToString().Trim()); } } } Unit Test Incomplete Pancake class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTest68bcf81d.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest68bcf81d.cs(23,7): error CS0246: The type or namespace name `Pancake' could not be found. Are you missing `TurningDemo' using directive? NtTest68bcf81d.cs(24,7): error CS0841: A local variable `pancake' cannot be used before it is declared Test Contents [TestFixture] public class PancakeClassTest { [Test] public void PancakeTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Pancake)), "`Pancake` class should implement `IRecoverable` class"); } [Test] public void PancakeTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Pancake pancake = new Pancake(); pancake.Turn(); string expected = "You turn a pancake when it's done on one side"; Assert.AreEqual(expected, sw.ToString().Trim()); } } } Unit Test Incomplete Corner class defined Build Status Build Failed Build Output Compilation failed: 3 error(s), 0 warnings NtTest014d6109.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest014d6109.cs(23,7): error CS0246: The type or namespace name `Corner' could not be found. Are you missing `TurningDemo' using directive? NtTest014d6109.cs(24,7): error CS0841: A local variable `corner' cannot be used before it is declared Test Contents [TestFixture] public class CornerClassTest { [Test] public void CornerTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Corner)), "`Corner` class should implement `IRecoverable` class"); } [Test] public void CornerTest2() { using (StringWriter sw = new StringWriter()) { Console.SetOut(sw); Corner corner = new Corner(); corner.Turn(); string expected = "You turn corners to go around the block"; Assert.AreEqual(expected, sw.ToString().Trim()); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
in c# i need to
Create an application named TurningDemo that creates instances of four classes: Page, Corner, Pancake, and Leaf.
Create an interface named ITurnable that contains a single method named Turn(). The classes named Page, Corner, Pancake, and Leaf implement ITurnable.
Create each class’s Turn() method to display an appropriate message. For example:
- The Page’s Turn() method should display You turn a page in a book.
- The Corner’s Turn() method should display You turn corners to go around the block.
- The Pancake's Turn() method should display You turn a pancake when it's done on one side.
- The Leaf's Turn() method should display A leaf turns colors in the fall.
i keep getting the errors and my code is in the pics
Unit Test
Incomplete
Page class defined
Build Status
Build Failed
Build Outpu
tCompilation failed: 3 error(s), 0 warnings
NtTest5f1df6cd.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTest5f1df6cd.cs(23,7): error CS0246: The type or namespace name `Page' could not be found. Are you missing `TurningDemo' using directive?
NtTest5f1df6cd.cs(24,7): error CS0841: A local variable `page' cannot be used before it is declared
[TestFixture]
public class PageClassTest
{
[Test]
public void PageTest()
{
Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Page)),
"`Page` class should implement `IRecoverable` class");
}
[Test]
public void PageTest2()
{
using (StringWriter sw = new StringWriter())
{
Console.SetOut(sw);
Page page = new Page();
page.Turn(); string expected = "You turn a page in a book";
Assert.AreEqual(expected, sw.ToString().Trim());
}
}
}
Unit Test
Incomplete
Leaf class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTeste0f8fa97.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTeste0f8fa97.cs(23,7): error CS0246: The type or namespace name `Leaf' could not be found. Are you missing `TurningDemo' using directive?
NtTeste0f8fa97.cs(24,7): error CS0841: A local variable `leaf' cannot be used before it is declared
[TestFixture]
public class LeafClassTest
{
[Test]
public void LeafTest()
{
Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Leaf)),
"`Leaf` class should implement `IRecoverable` class");
}
[Test]
public void LeafTest2()
{
using (StringWriter sw = new StringWriter())
{
Console.SetOut(sw);
Leaf leaf = new Leaf();
leaf.Turn(); string expected = "A leaf turns colors in the fall";
Assert.AreEqual(expected, sw.ToString().Trim());
}
}
}
Unit Test
Incomplete
Pancake class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTest68bcf81d.cs(12,26): error CS0246: The type or namespace name `ITurnable' could not be found. Are you missing `TurningDemo' using directive?
NtTest68bcf81d.cs(23,7): error CS0246: The type or namespace name `Pancake' could not be found. Are you missing `TurningDemo' using directive?
NtTest68bcf81d.cs(24,7): error CS0841: A local variable `pancake' cannot be used before it is declared
Test Contents[TestFixture]
public class PancakeClassTest
{
[Test]
public void PancakeTest() { Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Pancake)),
"`Pancake` class should implement `IRecoverable` class");
}
[Test]
public void PancakeTest2()
{
using (StringWriter sw = new StringWriter())
{
Console.SetOut(sw);
Pancake pancake = new Pancake(); pancake.Turn();
string expected = "You turn a pancake when it's done on one side"; Assert.AreEqual(expected, sw.ToString().Trim());
}
}
}
Unit Test
Incomplete
Corner class defined
Build Status
Build Failed
Build Output
Compilation failed: 3 error(s), 0 warnings
NtTest014d6109.cs(12,26): error CS0246: The type or namespace name
`ITurnable' could not be found. Are you missing `TurningDemo' using directive? NtTest014d6109.cs(23,7): error CS0246: The type or namespace name `Corner' could not be found. Are you missing `TurningDemo' using directive?
NtTest014d6109.cs(24,7): error CS0841: A local variable `corner' cannot be used before it is declared
[TestFixture]
public class CornerClassTest
{
[Test]
public void CornerTest()
{
Assert.IsTrue(typeof(ITurnable).IsAssignableFrom(typeof(Corner)),
"`Corner` class should implement `IRecoverable` class");
}
[Test]
public void CornerTest2()
{
using (StringWriter sw = new StringWriter())
{ Console.SetOut(sw); Corner corner = new Corner();
corner.Turn();
string expected = "You turn corners to go around the block";
Assert.AreEqual(expected, sw.ToString().Trim());
}
}
}
![41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
}
}
{
}
return "A leaf turns colors in the fall.";
class Program
{
static void Main(string[] args)
{
Page page1 = new Page();
Corner corner1 = new Corner();
Pancake pancake1 = new Pancake ();
Leaf leaf1 = new Leaf();
Console.WriteLine("Page: + page1. Turn());
Console.WriteLine("Corner:
Console.WriteLine("Pancake:
+ corner1. Turn());
+ pancake1. Turn());
Console.WriteLine("Leaf: " + leaf1.Turn());
Console.ReadLine();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda2a136f-d248-470a-adf7-afa3bf81602c%2F240060eb-4874-42e4-8935-2494d1b8df19%2Ffp8t2of_processed.png&w=3840&q=75)
Transcribed Image Text:41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
}
}
{
}
return "A leaf turns colors in the fall.";
class Program
{
static void Main(string[] args)
{
Page page1 = new Page();
Corner corner1 = new Corner();
Pancake pancake1 = new Pancake ();
Leaf leaf1 = new Leaf();
Console.WriteLine("Page: + page1. Turn());
Console.WriteLine("Corner:
Console.WriteLine("Pancake:
+ corner1. Turn());
+ pancake1. Turn());
Console.WriteLine("Leaf: " + leaf1.Turn());
Console.ReadLine();

Transcribed Image Text:1 using System;
using
2
3
using System.Linq;
4 using System.Text;
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
using System.Threading.Tasks;
namespace TurningDemo
{
System.Collections.Generic;
public interface ITurnable
}
}
class Page : ITurnable
{
}
string Turn();
}
public string Turn()
{
}
class Corner : ITurnable
{
return "You turn a page in the book.";
public string Turn()
{
}
return "You turn at the corner.";
class Pancake : ITurnable
{
public string Turn()
{
}
return "You turn a pancake.";
class Leaf : ITurnable
{
public string Turn()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
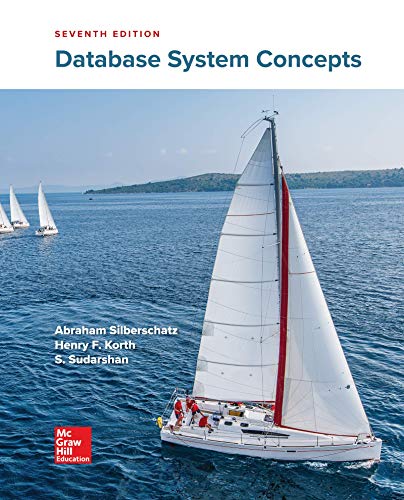
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
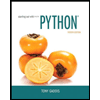
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
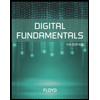
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
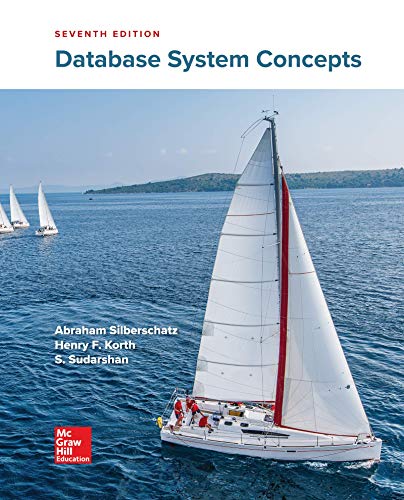
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
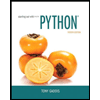
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
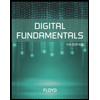
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
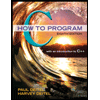
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
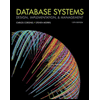
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
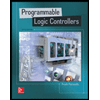
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education