import java.util.Scanner; class BloodData//declraing BloodData class { //declaring required variables static String bloodType;//to store the blood type static String rhFactor;//to store Rhesus factor //default public constructor public BloodData() { //initializing bloodType to o and rhFactor to + bloodType="o"; rhFactor="+"; } //parameterized public constructor public BloodData(String bt,String rh) { //initializing bloodType with bt and rhFactor with rh bloodType=bt; rhFactor=rh; } //function to print bloodType and rhFactor public void display() { //printing the bloodType and rhFactor System.out.println(bloodType+rhFactor+" is added to the bloodbank.\n"); } }//end of class BloodData public class RunBloodData//declaring public class RunBloodData { public static void main(String[] args) { //creating object of Scanner class to take input Scanner sc=new Scanner(System.in); //declaring bt and rh to store user inputs String bt,rh; System.out.print("Enter blood type of patient:"); bt=sc.nextLine();//taking input of blood type System.out.print("Enter the Rhesus factor (+ or -):"); rh=sc.nextLine();//taking input of Rhesus factor BloodData bd;//declaring object of BloodData //if input of blood type and Rhesus factor is empty if(bt.equals("")&&rh.equals("")) { //than call the default constructor bd=new BloodData(); //and call the display function bd.display(); } else//else input is not empty { //than call the parameterized constructor bd=new BloodData(bt,rh); //and call the display function bd.display(); } System.out.print("Enter blood type of patient:"); bt=sc.nextLine();//taking input of blood type System.out.print("Enter the Rhesus factor (+ or -):"); rh=sc.nextLine();//taking input of Rhesus factor BloodData bd2;//creating object of BloodData //if given inputs are empty if(bt.equals("")&&rh.equals("")) { //than call the default constructor bd2=new BloodData(); //and display function bd2.display(); } else//else inputs are not empty { //call the parameterized constructor bd2=new BloodData(bt,rh); //and display function bd2.display(); } } }//end of RunBloodData class
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
import java.util.Scanner; class BloodData//declraing BloodData class { //declaring required variables static String bloodType;//to store the blood type static String rhFactor;//to store Rhesus factor //default public constructor public BloodData() { //initializing bloodType to o and rhFactor to + bloodType="o"; rhFactor="+"; } //parameterized public constructor public BloodData(String bt,String rh) { //initializing bloodType with bt and rhFactor with rh bloodType=bt; rhFactor=rh; } //function to print bloodType and rhFactor public void display() { //printing the bloodType and rhFactor System.out.println(bloodType+rhFactor+" is added to the bloodbank.\n"); } }//end of class BloodData public class RunBloodData//declaring public class RunBloodData { public static void main(String[] args) { //creating object of Scanner class to take input Scanner sc=new Scanner(System.in); //declaring bt and rh to store user inputs String bt,rh; System.out.print("Enter blood type of patient:"); bt=sc.nextLine();//taking input of blood type System.out.print("Enter the Rhesus factor (+ or -):"); rh=sc.nextLine();//taking input of Rhesus factor BloodData bd;//declaring object of BloodData //if input of blood type and Rhesus factor is empty if(bt.equals("")&&rh.equals("")) { //than call the default constructor bd=new BloodData(); //and call the display function bd.display(); } else//else input is not empty { //than call the parameterized constructor bd=new BloodData(bt,rh); //and call the display function bd.display(); } System.out.print("Enter blood type of patient:"); bt=sc.nextLine();//taking input of blood type System.out.print("Enter the Rhesus factor (+ or -):"); rh=sc.nextLine();//taking input of Rhesus factor BloodData bd2;//creating object of BloodData //if given inputs are empty if(bt.equals("")&&rh.equals("")) { //than call the default constructor bd2=new BloodData(); //and display function bd2.display(); } else//else inputs are not empty { //call the parameterized constructor bd2=new BloodData(bt,rh); //and display function bd2.display(); } } }//end of RunBloodData class


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

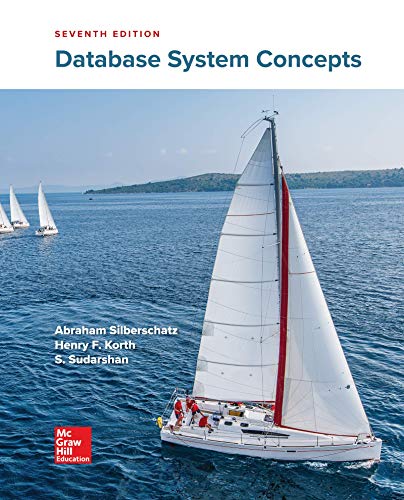
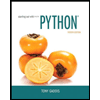
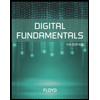
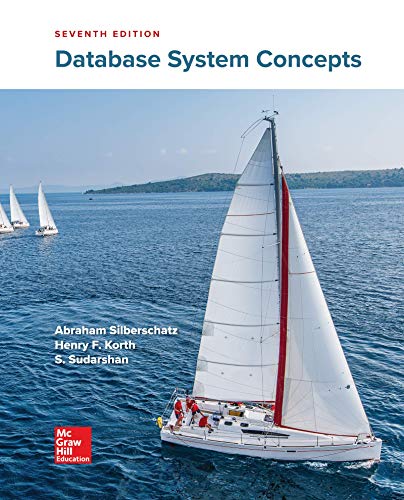
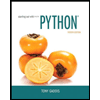
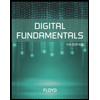
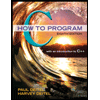
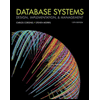
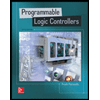