Implement the following two methods in O(n) time. // Reverse the list and return it in O(n) time public static ArrayList reverse(ArrayList list) // Reverse the list and return it in O(n) time public static LinkedList reverse(LinkedList list) Use the following code to test these methods: public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.print("Enter 10 numbers: "); ArrayList list = new ArrayList<>(); for (int i = 0; i < 10; i++) { list.add(input.nextInt()); } reverse(list); for (int i: list) { System.out.print(i + " "); } System.out.println(); LinkedList list1 = new LinkedList<>(list); reverse(list1); for (int i: list1) { System.out.print(i + " "); } System.out.println(); }
Implement the following two methods in O(n) time.
// Reverse the list and return it in O(n) time
public static <E> ArrayList<E> reverse(ArrayList<E> list)
// Reverse the list and return it in O(n) time
public static <E> LinkedList<E> reverse(LinkedList<E> list)
Use the following code to test these methods:
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter 10 numbers: ");
ArrayList<Integer> list = new ArrayList<>();
for (int i = 0; i < 10; i++) {
list.add(input.nextInt());
}
reverse(list);
for (int i: list) {
System.out.print(i + " ");
}
System.out.println();
LinkedList<Integer> list1 = new LinkedList<>(list);
reverse(list1);
for (int i: list1) {
System.out.print(i + " ");
}
System.out.println();
}

Step by step
Solved in 3 steps with 1 images

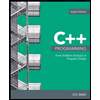
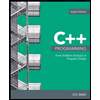