Implement solutions for the following methods: • getCourseSize() – returns the number of students registered in the course (not in the waitlist). It should maintain the public size variable that keeps track of the number of students registered. • getRegisteredIDs() – returns an array of int[], namely registered student id’s. The length of the array is the size (number of students) in the course. • getRegisteredStudents() – returns an array of type Student[], namely the registered Students. The length of the array is the current size (number of students) of the course. • getWaitlistedIDs() – returns an array of type int[], namely the ids of students in the waitlist. • getWaitlistedStudents() – returns an array of Students in the waitlist.
Implement solutions for the following methods:
• getCourseSize() – returns the number of students registered in the course (not in the waitlist). It should maintain the public size variable that keeps track of the number of students registered.
• getRegisteredIDs() – returns an array of int[], namely registered student id’s. The length of the array is the size (number of students) in the course.
• getRegisteredStudents() – returns an array of type Student[], namely the registered Students. The length of the array is the current size (number of students) of the course.
• getWaitlistedIDs() – returns an array of type int[], namely the ids of students in the waitlist.
• getWaitlistedStudents() – returns an array of Students in the waitlist.
public class Course {
public String code;
public int capacity;
public SLinkedList<Student>[] studentTable;
public int size;
public SLinkedList<Student> waitlist;
public Course(String code) {
this.code = code;
this.studentTable = new SLinkedList[10];
this.size = 0;
this.waitlist = new SLinkedList<Student>();
this.capacity = 10;
}
public Course(String code, int capacity) {
this.code = code;
this.studentTable = new SLinkedList[capacity];
this.size = 0;
this.waitlist = new SLinkedList<>();
this.capacity = capacity;
}
public int getCourseSize() {
// insert solution here and modify the return statement
return -1;
}
public int[] getRegisteredIDs() {
// insert solution here and modify the return statement
return null;
}
public Student[] getRegisteredStudents() {
// insert solution here and modify the return statement
return null;
}
public int[] getWaitlistedIDs() {
// insert solution here and modify the return statement
return null;
}
public Student[] getWaitlistedStudents() {
// insert solution here and modify the return statement
return null;
}
![public String tostring() {
30
4
String s = "Course: "+ this.code +"\n";
S +=
-\n";
for (int i = 0; i < this.studentTable.length; i++) {
|\n";
s += "|"+i+"
s += "|
SLinkedList<Student> list = this.studentTable[i];
if (list != null) {
for (int j = 0; j < list.size(); j++) {
------> ":
Student student = list.get(j);
S +=
student.id + ": "+ student.name +" --> ";
4
}
}
S += "\n-
--\n\n";
}
return s;
}
}
1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9c002e4e-ece1-4dd1-9934-cbb442392244%2F984a3784-e771-412b-a706-a4ac6868e633%2Fe3r7qvf_processed.png&w=3840&q=75)
![1 public class Course {
public String code;
public int capacity;
public SLinkedList<Student> [] studentTable;
public int size;
public SLinkedList<Student> waitlist;
4
5
6.
7
8
public Course(String code) {
this.code = code;
this.studentTable = new SLinkedList[10];
this.size = 0;
this.waitlist = new SLinkedList<Student>();
this.capacity
}
10
11
12
13
14
= 10;
15
16
public Course(String code, int capacity) {
this.code = code;
this.studentTable = new SLinkedList[capacity];
this.size = 0;
this.waitlist = new SLinkedList<>();
this.capacity = capacity;
}
17e
18
19
20
21
22
23
24
O 250
虽26
® 27
O 28
29
public void changeArrayLength(int m) {
public SLinkedList<Student>[] newStudentTable;
for (int i = 0; i < m; i++) {
newStudentTable[i] = new SLinkedList<Student>;
}
30
O 31
for (int i = 0; i < studentTable. length; i++) {
for (int j = 0; j < studentTable[i].size(); j++) {
int tempIndex = studentTable[i].get(j). getId() % m;
newStudentTable[tempIndex].add (studentTable[i].get(j));
}
}
32
A 33
x 34
35
36
37
38
studentTable = newStudentTable;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9c002e4e-ece1-4dd1-9934-cbb442392244%2F984a3784-e771-412b-a706-a4ac6868e633%2Fc8xazn_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

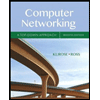
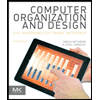
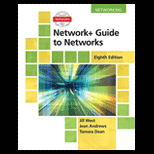
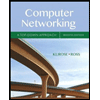
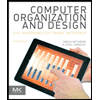
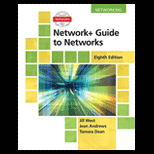
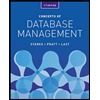
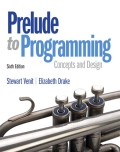
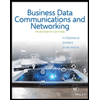