Implement a class IntArr using dynamic memory. a. data members: capacity: maximum number of elements in the array size: current number of elements in the array array: a pointer to a dynamic array of integers b. constructors: default constructor: 1. Set capacity to 5, size to 0 2. Create a dynamic array of capacity 5 user constructor: 1. Parameter c to set the capacity 2. Set capacity to c, size to 0 3. Create a dynamic array of capacity c c. overloaded operators: subscript operator: 1. Parameter index 2. Return the element at position index constant subscript operator: 1. Identical to subscript operator but for constants 2. This is required for the big three to work properly d. the big three - copy constructor: 1. Construct a new IntArr object from an existing IntArr object e. the big three - assignment overload operator: 1. Copy from an IntArr object to another IntArr object f. the big three - destructor: 1. Destroy the dynamic memory of and IntArr object g. grow function: 1. This should be a private function (located in private). 2. Grow the array so that the new capacity is original capacity*2+1 h. push_back function: 1. If size is equivalent to capacity call the grow function 2. Append a new integer to the end of the array i. pop_back function: 1. If the array is not empty, decrement size by one j. getSize function: 1. return the current size of the array k. getCapacity function: 1. return the current capacity of the array Output Test Constructors and Push_Back Array A: 5 10 15 20 25 size: 5 capacity: 5 Test Grow Calling grow for element 30 Array A: 5 10 15 20 25 30 35 size: 7 capacity: 11 Test Copy Constructor (IntArr b=a) Array A: 5 10 15 20 25 30 35 size: 7 capacity: 11 Array B: 5 10 15 20 25 30 35 size: 7 capacity: 11 Test Pop_Back (pop last two elements) Array B: 5 10 15 20 25 size: 5 capacity: 11 Test Assignment Operator (a=b) Array A: 5 10 15 20 25 size: 5 capacity: 11 Array B: 5 10 15 20 25 size: 5 capacity: 11
Implement a class IntArr using dynamic memory. a. data members: capacity: maximum number of elements in the array size: current number of elements in the array array: a pointer to a dynamic array of integers b. constructors: default constructor: 1. Set capacity to 5, size to 0 2. Create a dynamic array of capacity 5 user constructor: 1. Parameter c to set the capacity 2. Set capacity to c, size to 0 3. Create a dynamic array of capacity c c. overloaded operators: subscript operator: 1. Parameter index 2. Return the element at position index constant subscript operator: 1. Identical to subscript operator but for constants 2. This is required for the big three to work properly d. the big three - copy constructor: 1. Construct a new IntArr object from an existing IntArr object e. the big three - assignment overload operator: 1. Copy from an IntArr object to another IntArr object f. the big three - destructor: 1. Destroy the dynamic memory of and IntArr object g. grow function: 1. This should be a private function (located in private). 2. Grow the array so that the new capacity is original capacity*2+1 h. push_back function: 1. If size is equivalent to capacity call the grow function 2. Append a new integer to the end of the array i. pop_back function: 1. If the array is not empty, decrement size by one j. getSize function: 1. return the current size of the array k. getCapacity function: 1. return the current capacity of the array Output Test Constructors and Push_Back Array A: 5 10 15 20 25 size: 5 capacity: 5 Test Grow Calling grow for element 30 Array A: 5 10 15 20 25 30 35 size: 7 capacity: 11 Test Copy Constructor (IntArr b=a) Array A: 5 10 15 20 25 30 35 size: 7 capacity: 11 Array B: 5 10 15 20 25 30 35 size: 7 capacity: 11 Test Pop_Back (pop last two elements) Array B: 5 10 15 20 25 size: 5 capacity: 11 Test Assignment Operator (a=b) Array A: 5 10 15 20 25 size: 5 capacity: 11 Array B: 5 10 15 20 25 size: 5 capacity: 11
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I'm confused on what I need to do. What do I do?
My code:
#include <iostream>
#include <cassert>
using namespace std;
class IntArr {
public:
IntArr();
IntArr(int c);
int& operator[](int index);
constint& operator[](int index) const;
IntArr(const IntArr &a);
IntArr& operator=(const IntArr &a);
~IntArr();
int getSize() const;
int getCapacity() const;
void push_back(int n);
void pop_back();
private:
void grow();
int capacity;
int size;
int *array;
};
IntArr::IntArr(){
capacity = 5;
}
IntArr::IntArr(int c): capacity(c){
size = 0;
assert(c>0);
IntArr a{c};
}
int& IntArr::operator[](int index){
if(index > 0 && index <= size-1)
return a[index];
}
const int& IntArr::operator[](int index)const{
}
IntArr::IntArr(const IntArr& a){
}
IntArr& IntArr::operator=(const IntArr& a){
}
IntArr::~IntArr(){
}
int IntArr::getSize() const{
}
int IntArr::getCapacity() const{
}
void IntArr::grow(){
}
void IntArr::push_back(int n){
}
void IntArr::pop_back(){
}
int main(){
IntArr a{5};
cout << "Test Constructors and Push_Back" << endl;
for(int i = 0; i<5; i++) {a.push_back((i+1)*5); }
cout<<"Array A; ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] << " "; }
cout<< "size: " << a.getSize() << " capacity: " << a.getCapacity() << endl;
cout << "\nTest Grow" << endl;
a.push_back(30);
a.push_back(35);
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] <<" "; }
cout << "size: "<< a.getSize() << " capacity: "<< a.getCapacity() <<endl;
cout<<"\nTest Copy Constructor (IntArr b=a)"<<endl;
IntArr b = a;
cout<<"Array A: ";
for(int i=0; i<a.getSize(); i++) { cout<<a[i]<<" "; }
cout<<"size: "<<a.getSize()<<" capacity: "<<a.getCapacity()<<endl;
cout<<"Array B: ";
for(int i=0; i<b.getSize(); i++) { cout<<b[i]<<" "; }
cout<<"size: "<<b.getSize()<<" capacity: "<<b.getCapacity()<<endl;
cout<<"\nTest Pop_back (pop last two elements)"<<endl;
b.pop_back();
b.pop_back();
cout<<"Array B: ";
for(int i = 0; i<b.getSize(); i++){cout<<b[i]<<" "; }
cout<<"size: "<<b.getSize()<<" capacity: "<<b.getCapacity()<<endl;
cout<<"\nTest Assignment Operator (a=b)"<<endl;
a=b;
cout<<"Array A: ";
for(int i=0; i<a.getSize(); i++){cout<<a[i]<<" ";}
cout<<"size: "<<a.getSize()<<" capacity: "<<a.getCapacity()<<endl;
cout<<"Array B: ";
for(int i=0; i<b.getSize(); i++) { cout<<b[i]<<" ";
cout<<"size: "<<b.getSize()<<" capacity: "<<b.getCapacity()<<endl;
}
}

Transcribed Image Text:Implement a class IntArr using dynamic memory.
a. data members:
capacity: maximum number of elements in the array
size: current number of elements in the array
array: a pointer to a dynamic array of integers
b. constructors:
default constructor:
1. Set capacity to 5, size to 0
2. Create a dynamic array of capacity 5
user constructor:
1. Parameter c to set the capacity
2. Set capacity to c, size to 0
3. Create a dynamic array of capacity c
c. overloaded operators:
subscript operator:
1. Parameter index
2. Return the element at position index
constant subscript operator:
1. Identical to subscript operator but for constants
2. This is required for the big three to work properly
d. the big three - copy constructor:
1. Construct a new IntArr object from an existing IntArr object
e.
the big three - assignment overload operator:
1. Copy from an IntArr object to another IntArr object
f. the big three - destructor:
1. Destroy the dynamic memory of and IntArr object
g. grow function:
1. This should be a private function (located in private).
2. Grow the array so that the new capacity is original capacity*2+1
h. push_back function:
1. If size is equivalent to capacity call the grow function
2. Append a new integer to the end of the array
i. pop_back function:
1. If the array is not empty, decrement size by one
j. getSize function:
1. return the current size of the array
k. getCapacity function:
1. return the current capacity of the array

Transcribed Image Text:Output
Test Constructors and Push_Back
Array A: 5 10 15 20 25 size: 5 capacity: 5
Test Grow
Calling grow for element 30
Array A: 5 10 15 20 25 30 35 size: 7 capacity: 11
Test Copy Constructor (IntArr b=a)
Array A: 5 10 15 20 25 30 35 size: 7 capacity: 11
Array B: 5 10 15 20 25 30 35 size: 7 capacity: 11
Test Pop_Back (pop last two elements)
Array B: 5 10 15 20 25 size: 5 capacity: 11
Test Assignment Operator (a=b)
Array A: 5 10 15 20 25 size: 5 capacity: 11
Array B: 5 10 15 20 25 size: 5 capacity: 11
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
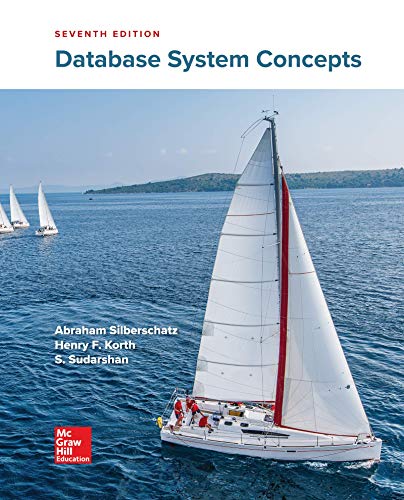
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
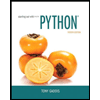
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
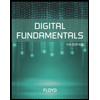
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
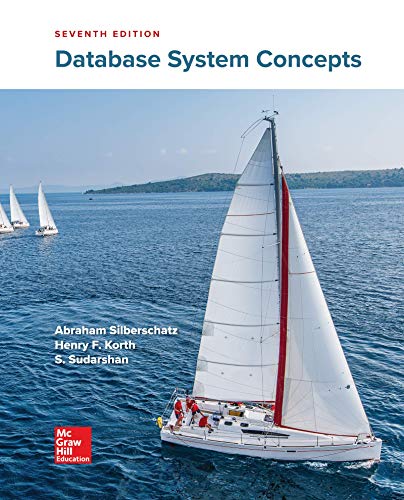
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
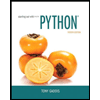
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
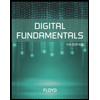
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
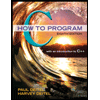
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
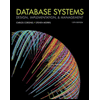
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
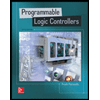
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education