I need help. I have the following code: class Money { private int dollar; private int cent; final static int MIN_CENT_VALUE=0; final static int MAX_CENT_VALUE=99; public Money(){ this.dollar=0; this.cent=0; } public Money(int dollar,int cent){ this.dollar=dollar; if(cent>=MIN_CENT_VALUE&¢<=MAX_CENT_VALUE){ this.cent=cent; }else{ cent=0; } } public int getDollar(){ return this.dollar; } public void setDollar(int dol){ this.dollar=dol; } public int getCent(){ return this.cent; } public void setCent(int c){ if(c>=MIN_CENT_VALUE&&c<=MAX_CENT_VALUE){ this.cent=c; } } public Money add(Money otherMoney){ this.dollar+=otherMoney.dollar; this.cent+=otherMoney.cent; return this; } public Money add(Money otherMoney){ this.dollar-=otherMoney.dollar; this.cent-=otherMoney.cent; if(this.dollar<0||this.cent<0){ return null; } return this; } public boolean equals(Money otherMoney){ return this==otherMoney; } public int compareTo(Money otherMoney){ if(this.dollar m){ Money res=m.get(0); for(int i=0;i m){ for(int i=0;i
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I need help. I have the following code:
class Money {
private int dollar;
private int cent;
final static int MIN_CENT_VALUE=0;
final static int MAX_CENT_VALUE=99;
public Money(){
this.dollar=0;
this.cent=0;
}
public Money(int dollar,int cent){
this.dollar=dollar;
if(cent>=MIN_CENT_VALUE&¢<=MAX_CENT_VALUE){
this.cent=cent;
}else{
cent=0;
}
}
public int getDollar(){
return this.dollar;
}
public void setDollar(int dol){
this.dollar=dol;
}
public int getCent(){
return this.cent;
}
public void setCent(int c){
if(c>=MIN_CENT_VALUE&&c<=MAX_CENT_VALUE){
this.cent=c;
}
}
public Money add(Money otherMoney){
this.dollar+=otherMoney.dollar;
this.cent+=otherMoney.cent;
return this;
}
public Money add(Money otherMoney){
this.dollar-=otherMoney.dollar;
this.cent-=otherMoney.cent;
if(this.dollar<0||this.cent<0){
return null;
}
return this;
}
public boolean equals(Money otherMoney){
return this==otherMoney;
}
public int compareTo(Money otherMoney){
if(this.dollar<otherMoney.dollar){
return 1;
}else if(this.dollar==otherMoney.dollar){
return 0;
}else{
return -1;
}
}
public String toString(){
return "Dollar is"+this.dollar+"and cents is"+this.cent;
}
public Money max(ArrayList<Money> m){
Money res=m.get(0);
for(int i=0;i<m.size();i++){
if(res.dollar<m.get(i).dollar){
res=m.get(i);
}
}
return res;
}
public void publicList(ArrayList<Money> m){
for(int i=0;i<m.size();i++){
System.out.print(m.get(i)+" ");
}
}
}
_________________________________________________
for use with this driver program, testMoney.java
import java.util.*;
public class TestMoney
{
public static void main(String[] args)
{
Random rnd = new Random(10);
Money m1 = new Money(5,45);
Money m2 = new Money(10,95);
m1.setCent(101);
Money sum = m1.add(m2);
System.out.println(m1+" + "+m2+" is "+sum);
Money diff = m1.subtract(m2);
System.out.println(m1+" - "+m2+" is "+diff);
diff = m2.subtract(m1);
System.out.println(m2+" - "+m1+" is "+diff);
System.out.println("equals method result: "+m1.equals(m2));
System.out.println("compareTo method result: "+m1.compareTo(m2));
Money[] list = new Money[15];
// Generate 15 random Money objects
for(int i=0;i<list.length;++i)
{
list[i] = new Money((int)(1+rnd.nextInt(30)),rnd.nextInt(100));
}
// print the list of Money objects, 10 per line
System.out.println("Unsorted list");
Money.printList(list,10);
// Find the largest Money object
System.out.println("The Max is "+Money.max(list));
// Sort and print
System.out.println("Sorted list");
Money.selectionSort(list);
Money.printList(list,10);
}
}
____________________________________________
but I keep getting these error messages:
![Failed to compile
Money.java:48:
error: method add (Money) is already defined in class Money
public Money add (Money otherMoney) {
Money.java:75: error: cannot find symbol
public Money max (ArrayList<Money> m) {
symbol: class ArrayList
location: class Money
Money.java:85: error: cannot find symbol
public void publicList (ArrayList<Money> m) {
symbol: class ArrayList
location: class Money
TestMoney.java:12: error: cannot find symbol
Money diff = ml.subtract (m2);
symbol: method subtract (Money)
location: variable m1 of type Money
TestMoney.java:14: error: cannot find symbol
diff = m2.subtract (m1);
symbol: method subtract (Money)
location: variable m2 of type Money
TestMoney.java:24: warning: [cast] redundant cast to int
list[i] = new Money ( (int) (1+rnd.nextInt (30)), rnd.nextInt (100));
TestMoney.java:28: error: cannot find symbol
Money.printList (list, 10);
symbol: method printList (Money [], int)
location: class Money
TestMoney.java:30: error: non-static method max (<any>) cannot be referenced from a static co
System.out.println("The Max is "+Money.max (list));
TestMoney.java:33: error: cannot find symbol
Money.selectionSort (list);
symbol: method selectionSort (Money [])
location: class Money
TestMoney.java:34: error: cannot find symbol
Money.printList (list, 10);
symbol: method printList (Money [], int)
location: class Money
9 errors
1 warning](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe4e826cb-c133-47e8-aeaa-858e7b3a09da%2Fa58866c4-6060-440e-90f5-24cd77611912%2Fvlga0p8_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

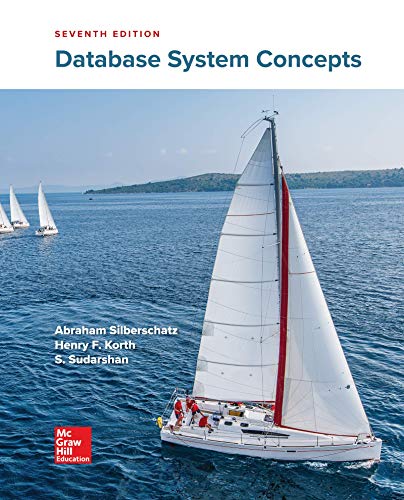
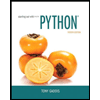
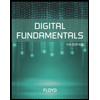
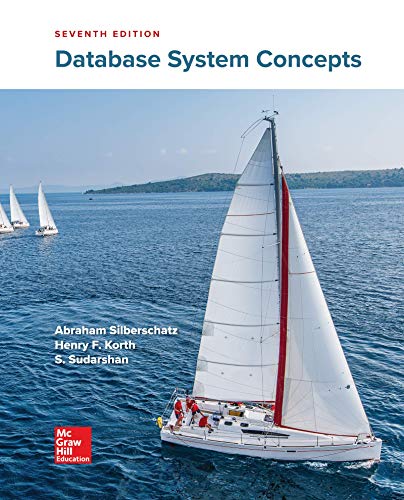
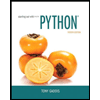
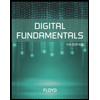
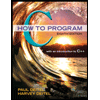
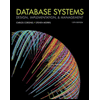
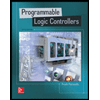