I have this program that runs perfetly but receives a stylecheck error and I have no idea how to fix the error. I will attach the style check error below and the sample outputs. main.cc file #include #include "rectangle.h" int main() { unsigned int length = 0; unsigned int width = 0; Rectangle r1; Rectangle r2; std::cout << "====== Rectangle 1 ======" << std::endl; // ===================== YOUR CODE HERE ======================== // Accept user input for the length and width of rectangle 1, // and instantiate a new Rectangle object with these inputs. // ============================================================= std::cout << "Please enter the length: "; // input length std::cin>>length; r1.SetLength(length); std::cout << "Please enter the width: "; // input width std::cin>>width; r1.SetWidth(width); std::cout << "Rectangle 1 created with length " << r1.GetLength() << " and width " << r1.GetWidth() << std::endl; std::cout << "The area of rectangle 1 is: " << r1.Area() << std::endl; std::cout << "The perimeter of Rectangle 1 is: " << r1.Perimeter() << std::endl << std::endl; std::cout << "====== Rectangle 2 ======" << std::endl; // ===================== YOUR CODE HERE ======================== // Accept user input for the length and width of rectangle 2, // and instantiate a new Rectangle object with these inputs. // ============================================================= std::cout << "Please enter the length: "; std::cin>>length; r2.SetLength(length); std::cout << "Please enter the width: "; std::cin>>width; r2.SetWidth(width); std::cout << "Rectangle 2 created with length " << r2.GetLength() << " and width " << r2.GetWidth() << std::endl; std::cout << "The area of rectangle 2 is: " << r2.Area() << std::endl; std::cout << "The perimeter of Rectangle 2 is: " << r2.Perimeter() << std::endl << std::endl; // ===================== YOUR CODE HERE ======================== // Call LargestRectangleByArea to determine which rectangle // is larger, and print out its length, width, and area. // Follow the README for formatting. // ============================================================= Rectangle largestRectangle = LargestRectangleByArea(r1, r2); std::cout << "The largest rectangle has a length of " << largestRectangle.GetLength() << ", a width of " << largestRectangle.GetWidth() << ", and an area of " << largestRectangle.Area() << "." << std::endl; return 0; } rectangle.cc file #include "rectangle.h" unsigned int Rectangle::Area() const { // ===================== YOUR CODE HERE ======================== // Compute the area of this rectangle object. // Remember that member functions can access the member variables of the // class. Hint: look at `rectangle.h` to see the member variables you can // access. // ============================================================= unsigned int area = length_ * width_; return area; } unsigned int Rectangle::Perimeter() const { // ===================== YOUR CODE HERE ======================== // Compute the perimeter of this rectangle object. // ============================================================= unsigned int perimeter = 2 * (length_ + width_); return perimeter; } Rectangle LargestRectangleByArea(Rectangle &r1, Rectangle &r2) { // ===================== YOUR CODE HERE ======================== // Compare the areas of the two given rectangles, and return // the Rectangle whose area is larger. // ============================================================= Rectangle result = r1.Area() > r2.Area() ? r1 : r2; return result; } rectangle.h file class Rectangle { public: // Setter functions of the Rectangle class. void SetLength(unsigned int length) { length_ = length; } void SetWidth(unsigned int width) { width_ = width; } // Getter functions of the Rectangle class. unsigned int GetLength() const { return length_; } unsigned int GetWidth() const { return width_; } // Other member functions of the Rectangle class. unsigned int Area() const; unsigned int Perimeter() const; private: // Member variables of the Rectangle class. unsigned int length_; unsigned int width_; }; Rectangle LargestRectangleByArea(Rectangle &r1, Rectangle &r2);
I have this program that runs perfetly but receives a stylecheck error and I have no idea how to fix the error. I will attach the style check error below and the sample outputs.
main.cc file
#include <iostream>
#include "rectangle.h"
int main() {
unsigned int length = 0;
unsigned int width = 0;
Rectangle r1;
Rectangle r2;
std::cout << "====== Rectangle 1 ======" << std::endl;
// ===================== YOUR CODE HERE ========================
// Accept user input for the length and width of rectangle 1,
// and instantiate a new Rectangle object with these inputs.
// =============================================================
std::cout << "Please enter the length: "; // input length
std::cin>>length;
r1.SetLength(length);
std::cout << "Please enter the width: "; // input width
std::cin>>width;
r1.SetWidth(width);
std::cout << "Rectangle 1 created with length " << r1.GetLength() << " and width " << r1.GetWidth() << std::endl;
std::cout << "The area of rectangle 1 is: " << r1.Area() << std::endl;
std::cout << "The perimeter of Rectangle 1 is: " << r1.Perimeter() << std::endl << std::endl;
std::cout << "====== Rectangle 2 ======" << std::endl;
// ===================== YOUR CODE HERE ========================
// Accept user input for the length and width of rectangle 2,
// and instantiate a new Rectangle object with these inputs.
// =============================================================
std::cout << "Please enter the length: ";
std::cin>>length;
r2.SetLength(length);
std::cout << "Please enter the width: ";
std::cin>>width;
r2.SetWidth(width);
std::cout << "Rectangle 2 created with length " << r2.GetLength() << " and width " << r2.GetWidth() << std::endl;
std::cout << "The area of rectangle 2 is: " << r2.Area() << std::endl;
std::cout << "The perimeter of Rectangle 2 is: " << r2.Perimeter() << std::endl << std::endl;
// ===================== YOUR CODE HERE ========================
// Call LargestRectangleByArea to determine which rectangle
// is larger, and print out its length, width, and area.
// Follow the README for formatting.
// =============================================================
Rectangle largestRectangle = LargestRectangleByArea(r1, r2);
std::cout << "The largest rectangle has a length of " << largestRectangle.GetLength() << ", a width of "
<< largestRectangle.GetWidth() << ", and an area of " << largestRectangle.Area() << "." << std::endl;
return 0;
}
rectangle.cc file
#include "rectangle.h"
unsigned int Rectangle::Area() const {
// ===================== YOUR CODE HERE ========================
// Compute the area of this rectangle object.
// Remember that member functions can access the member variables of the
// class. Hint: look at `rectangle.h` to see the member variables you can
// access.
// =============================================================
unsigned int area = length_ * width_;
return area;
}
unsigned int Rectangle::Perimeter() const {
// ===================== YOUR CODE HERE ========================
// Compute the perimeter of this rectangle object.
// =============================================================
unsigned int perimeter = 2 * (length_ + width_);
return perimeter;
}
Rectangle LargestRectangleByArea(Rectangle &r1, Rectangle &r2) {
// ===================== YOUR CODE HERE ========================
// Compare the areas of the two given rectangles, and return
// the Rectangle whose area is larger.
// =============================================================
Rectangle result = r1.Area() > r2.Area() ? r1 : r2;
return result;
}
rectangle.h file
class Rectangle {
public:
// Setter functions of the Rectangle class.
void SetLength(unsigned int length) {
length_ = length;
}
void SetWidth(unsigned int width) {
width_ = width;
}
// Getter functions of the Rectangle class.
unsigned int GetLength() const {
return length_;
}
unsigned int GetWidth() const {
return width_;
}
// Other member functions of the Rectangle class.
unsigned int Area() const;
unsigned int Perimeter() const;
private:
// Member variables of the Rectangle class.
unsigned int length_;
unsigned int width_;
};
Rectangle LargestRectangleByArea(Rectangle &r1, Rectangle &r2);
![35890 warnings generated.
/home/runner/lab04-danielhdz2000/prob02/main.cc:16:3: warning: uninitialized record typ
e: 'r1' [cppcoreguidelines-pro-type-member-init, hicpp-member-init]
Rectangle r1;
{}
/home/runner/lab04-danielhdz2000/prob02/main.cc:17:3: warning: uninitialized record typ
e: 'r2¹ [cppcoreguidelines-pro-type-member-init, hicpp-member-init]
Rectangle r2;
{}
/home/runner/Lab04-danielhdz2000/prob02/main.cc:52:13:
variable largestRectangle' [readability-identifier-naming]
Rectangle largestRectangle = LargestRectangleByArea(r1, r2);
NN
largest rectangle
NN
Style checker failed
warning: invalid case style for](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7b779863-aa72-4537-9750-69366ddc9e6e%2F612d3371-98f3-4e26-b9d7-4de695714824%2Fyr1dl66_processed.png&w=3840&q=75)


The solution is given below for the above given question:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

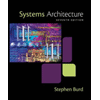
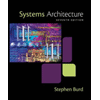