I have an error code that keeps poping up about "Editor does not contain Main type" but everything looks good code wise. My code with the professor instructions. import java.util.*; class Program12_1 { //PSEUDOCODE //Write a program as follows //prompt the user to enter a positive value of type byte. //cast this input first to type int and then to type char. //if the char is a printable ASCII character (from 32 to 122 ), display it. //if the user's input is bad, the program should end gracefully with an error message. public static void main(String[] args) { Scanner key = new Scanner(System.in); System.out.print("Enter a positive byte value "); String num = key.nextLine(); int number; try { number = Integer.parseInt(num); if(number >= 32 && number <= 123) { System.out.println("In ASCII, that is character " + (char) number); } else { System.out.println("Bad input. Run program again"); } } catch(Exception e) { System.out.println("Bad input. Run program again"); } key.close(); } }
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
I have an error code that keeps poping up about "Editor does not contain Main type" but everything looks good code wise.
My code with the professor instructions.
import java.util.*;
class Program12_1 {
//PSEUDOCODE
//Write a
//prompt the user to enter a positive value of type byte.
//cast this input first to type int and then to type char.
//if the char is a printable ASCII character (from 32 to 122 ), display it.
//if the user's input is bad, the program should end gracefully with an error message.
public static void main(String[] args) {
Scanner key = new Scanner(System.in);
System.out.print("Enter a positive byte value ");
String num = key.nextLine();
int number;
try {
number = Integer.parseInt(num);
if(number >= 32 && number <= 123) {
System.out.println("In ASCII, that is character " + (char) number);
}
else {
System.out.println("Bad input. Run program again");
}
}
catch(Exception e) {
System.out.println("Bad input. Run program again");
}
key.close();
}
}

Step by step
Solved in 3 steps with 1 images

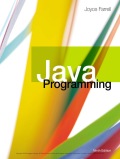
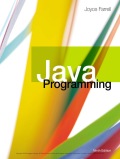