I // Fig. 12.9: Employee.h 2 // Employee abstract base class. 3 #ifndef EMPLOYEE_H 4 #define EMPLOYEE_H 5 6 #include // C++ standard string class 7 8 class Employee { 9 public: Employee(const std::string&, const std::string&, const std::string &); virtual -Employee() - default; // compiler generates virtual destructor 10 II 12 13 void setFirstName(const std::string&); // set first name std::string getFirstName() const; // return first name 14 15 16 void setlastName (const std::string&); // set last name std::string getLastName () const; // return last name 17 18 19 void setSocialSecurityNumber(const std::string&); // set SSN std::string getSocialSecurityNumber () const; !/ return SSN 20 21 // pure virtual function makes Employee an abstract base class virtual double earnings () const 0; // pure virtual virtual std::string toString() const; // virtual private: std::string firstName; std::string lastName; std::string socialSecurityNumber; 22 23 24 25 26 27 28 29 }; 30 31 #endif // EMPLOYEE_H Fig. 12.9 Employee abstract base class. // Fig. 12.10: Employee.cpp 2 // Abstract-base-class Employee menber-function definitions. 3 // Note: No definitions are given for pure virtual functions. 4 #include 5 #include "Employee.h" // Employee class definition 6 using namespace std; 7 8 // constructor 9 Employee::Employee (const string& first, const string& last, const string& ssn) : firstName(first), lastName(last), socialsSecurityNumber(ssn) {} 10 II 12 // set first name 14 void Employee::setFirstName (const string& first) {firstName = first;} 13 15 16 // return first name 17 string Employee::getFirstName () const {return firstName;} 18 19 // set last name 20 void Employee::setLastName (const string& last) {lastName = last;} 21 22 // return last name string Employee::getlastName () const {return lastName;} 24 // set social security number void Employee::setSocialSecurityNumber (const string& ssn) { socialSecurityNumber = ssn; // should validate } 23 25 26 27 28 29 // return social security number string Employee::getSocialSecurityNumber() const { return socialSecurityNumber; 30 31 32 33 34 35 // tostring Employee's information (virtual, but not pure virtual) 36 string Employee::toString () const { return getFirstName() + " "s + getLastName ) + "\nsocial security number: "s + getSocialSecurityNumber(); 37 38 39 Fig. 12.10 Employee Class implementation file.
I // Fig. 12.9: Employee.h 2 // Employee abstract base class. 3 #ifndef EMPLOYEE_H 4 #define EMPLOYEE_H 5 6 #include // C++ standard string class 7 8 class Employee { 9 public: Employee(const std::string&, const std::string&, const std::string &); virtual -Employee() - default; // compiler generates virtual destructor 10 II 12 13 void setFirstName(const std::string&); // set first name std::string getFirstName() const; // return first name 14 15 16 void setlastName (const std::string&); // set last name std::string getLastName () const; // return last name 17 18 19 void setSocialSecurityNumber(const std::string&); // set SSN std::string getSocialSecurityNumber () const; !/ return SSN 20 21 // pure virtual function makes Employee an abstract base class virtual double earnings () const 0; // pure virtual virtual std::string toString() const; // virtual private: std::string firstName; std::string lastName; std::string socialSecurityNumber; 22 23 24 25 26 27 28 29 }; 30 31 #endif // EMPLOYEE_H Fig. 12.9 Employee abstract base class. // Fig. 12.10: Employee.cpp 2 // Abstract-base-class Employee menber-function definitions. 3 // Note: No definitions are given for pure virtual functions. 4 #include 5 #include "Employee.h" // Employee class definition 6 using namespace std; 7 8 // constructor 9 Employee::Employee (const string& first, const string& last, const string& ssn) : firstName(first), lastName(last), socialsSecurityNumber(ssn) {} 10 II 12 // set first name 14 void Employee::setFirstName (const string& first) {firstName = first;} 13 15 16 // return first name 17 string Employee::getFirstName () const {return firstName;} 18 19 // set last name 20 void Employee::setLastName (const string& last) {lastName = last;} 21 22 // return last name string Employee::getlastName () const {return lastName;} 24 // set social security number void Employee::setSocialSecurityNumber (const string& ssn) { socialSecurityNumber = ssn; // should validate } 23 25 26 27 28 29 // return social security number string Employee::getSocialSecurityNumber() const { return socialSecurityNumber; 30 31 32 33 34 35 // tostring Employee's information (virtual, but not pure virtual) 36 string Employee::toString () const { return getFirstName() + " "s + getLastName ) + "\nsocial security number: "s + getSocialSecurityNumber(); 37 38 39 Fig. 12.10 Employee Class implementation file.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
(Enhanced Employee Class) Modify class Employee in Figs. 12.9–12.10 by adding a private utility function called isValidSocialSecurityNumber . This member function should validate the format of a social security number (e.g., ###-##- #### , where # is a digit). If the format is valid, return true ; otherwise return false . |

Transcribed Image Text:I // Fig. 12.9: Employee.h
2 // Employee abstract base class.
3 #ifndef EMPLOYEE_H
4 #define EMPLOYEE_H
5
6 #include <string> // C++ standard string class
7
8 class Employee {
9 public:
Employee(const std::string&, const std::string&, const std::string &);
virtual -Employee() - default; // compiler generates virtual destructor
10
II
12
13
void setFirstName(const std::string&); // set first name
std::string getFirstName() const; // return first name
14
15
16
void setlastName (const std::string&); // set last name
std::string getLastName () const; // return last name
17
18
19
void setSocialSecurityNumber(const std::string&); // set SSN
std::string getSocialSecurityNumber () const; !/ return SSN
20
21
// pure virtual function makes Employee an abstract base class
virtual double earnings () const 0; // pure virtual
virtual std::string toString() const; // virtual
private:
std::string firstName;
std::string lastName;
std::string socialSecurityNumber;
22
23
24
25
26
27
28
29 };
30
31
#endif // EMPLOYEE_H
Fig. 12.9 Employee abstract base class.

Transcribed Image Text:// Fig. 12.10: Employee.cpp
2 // Abstract-base-class Employee menber-function definitions.
3 // Note: No definitions are given for pure virtual functions.
4 #include <sstream>
5 #include "Employee.h" // Employee class definition
6 using namespace std;
7
8 // constructor
9 Employee::Employee (const string& first, const string& last,
const string& ssn)
: firstName(first), lastName(last), socialsSecurityNumber(ssn) {}
10
II
12
// set first name
14 void Employee::setFirstName (const string& first) {firstName = first;}
13
15
16 // return first name
17 string Employee::getFirstName () const {return firstName;}
18
19 // set last name
20 void Employee::setLastName (const string& last) {lastName = last;}
21
22 // return last name
string Employee::getlastName () const {return lastName;}
24
// set social security number
void Employee::setSocialSecurityNumber (const string& ssn) {
socialSecurityNumber = ssn; // should validate
}
23
25
26
27
28
29
// return social security number
string Employee::getSocialSecurityNumber() const {
return socialSecurityNumber;
30
31
32
33
34
35 // tostring Employee's information (virtual, but not pure virtual)
36 string Employee::toString () const {
return getFirstName() + " "s + getLastName ) +
"\nsocial security number: "s + getSocialSecurityNumber();
37
38
39
Fig. 12.10 Employee Class implementation file.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
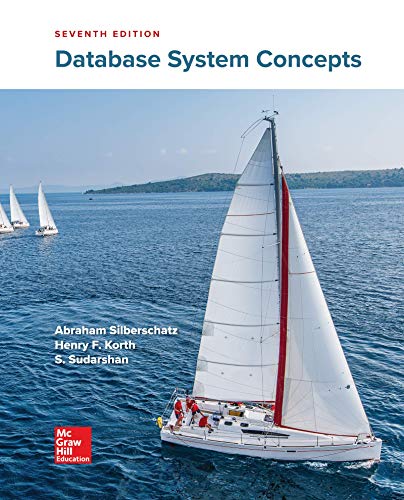
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
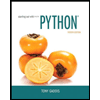
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
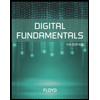
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
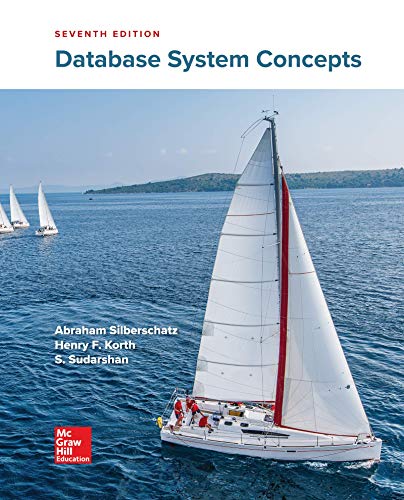
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
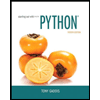
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
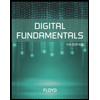
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
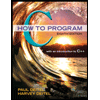
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
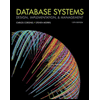
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
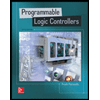
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education