I am working on a project to create a program containing a main() that uses a recursive function to create a Koch curve. We output cout statements and do output redirection at time of execution to a .ps file and view it there to see the results, how do I calculate the points?
I am working on a project to create a program containing a main() that uses a recursive function to create a Koch curve. We output cout statements and do output redirection at time of execution to a .ps file and view it there to see the results, how do I calculate the points?
Chapter16: Graphics
Section: Chapter Questions
Problem 4RQ
Related questions
Question
I am working on a project to create a program containing a main() that uses a recursive function to create a Koch curve. We output cout statements and do output redirection at time of execution to a .ps file and view it there to see the results, how do I calculate the points?

Transcribed Image Text:and Nineto Commands indicated
showpage Causes the graphics to be rendered to the page (required if you want to see or
print the page).
By default, the units for coordinates are points (a point is 1/72 of an inch); therefore the
commands 11 moveto 72 72 lineto stroke Would produce a 1.4 inch diagonal line from the lower
left comer. A simple postscript program that draws a level 1 snowflake is (generated by koch 72
360 504 360 1):
X!PS-Adobe -2.0
72
360 moveto
144
O rlineto
72 125 rlineto
72 -125 rlineto
144
O rlineto
stroke
showpage
Statement of Work
Write a program (yes, that means an entire program, including acpp file with main()) to draw a
Koch curve of arbitrary level and with arbitrary starting line segment endpoints using a recursive
algorithm. Your program should take the initial line segment endpoints and the desired final level
as command line arguments. So, if your executable is named "koch" (on Linux/MacOS) or
"koch.exe" (on Windows), you would run it as:
koch x1 y1 x2 y2 level
The first four arguments are the starting line segment endpoints (1,n) and (,n) in page
coordinates; the last is the curve level (2 0).
Your program should send to cout a Postscript program (which is plain text) to draw the
requested curve. If you save this to a file with an extension of pE (using output redirection, for
on the command line), you can view the result on MacOS and Linux by double-
example with
clicking on the .pz file (for Windows, you may need to download Ghostscript
(https://www.ghostscript.com/)_to preview Postscript files). You can also preview Postscript files
using Google Drive, by saving the file to Drive and then just double-clicking there.
2/13/2022, 1:17 PM
Ilround
Expert Solution

Step 1 Code to implement Koch curve
Python Code to implement Koch curve
from turtle import *
def snowflake(lengthSide, levels):
if levels == 0:
forward(lengthSide)
return
lengthSide /= 3.0
snowflake(lengthSide, levels-1)
left(60)
snowflake(lengthSide, levels-1)
right(120)
snowflake(lengthSide, levels-1)
left(60)
snowflake(lengthSide, levels-1)
if __name__ == "__main__":
speed(0)
length = 300.0
penup()
backward(length/2.0)
pendown()
for i in range(3):
snowflake(length, 4)
right(120)
mainloop()
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
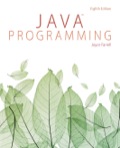
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
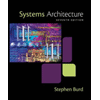
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
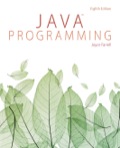
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
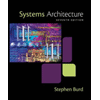
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning