How to get my second switch operations to work. import java.util.Stack; import java.util.Scanner; public class PostfixEvaluator { private final static char ADD = '+'; private final static char SUBTRACT = '-'; private final static char MULTIPLY = '*'; private final static char DIVIDE = '/'; private final static char MODULUS = '%'; private final static char NEGATIVE = 'm'; private final static char EXCHANGE = 'r'; private final static char DUPLICATE = 'd'; private final static char PRINT = 'p'; private final static char PRINT_AND_REMOVE = 'n'; private final static char PRINT_ALL = 'f'; private final static char CLEARall = 'c'; private final static char QUIT = 'q'; private final static char HElP = '?'; private Stack stack; public PostfixEvaluator() { stack = new Stack(); } public int evaluate(String expr) { int op1, op2, result = 0; String token; Scanner parser = new Scanner(expr); while (parser.hasNext()) { token = parser.next(); if (isOperator(token)) { op2 = (stack.pop()).intValue(); op1 = (stack.pop()).intValue(); result = evaluateSingleOperator(token.charAt(0), op1, op2); stack.push(new Integer(result)); } if (isCommand(token)); evaluateother(token.charAt(0), op1, op2); } else stack.push(new Integer(Integer.parseInt(token))); } return result; } /////////////////////////////////////////////////////////////////////////////////// private boolean isOperator(String token) { return (token.equals("+") || token.equals("-") ||token.equals("*") || token.equals("/") || token.equals("%") || token.equals("m")); } private boolean isCommand(String token) { return (token.equals("r") || token.equals("d") || token.equals("p") || token.equals("n") || token.equals("f") || token.equals("c") || token.equals("q") || token.equals("?")); } /////////////////////////////////////////////////////////////////////////////////// private int evaluateSingleOperator(char operation, int op1, int op2) { int result = 0; switch (operation) { case ADD: // this is + result = op1 + op2; break; case SUBTRACT: // this is - result = op1 - op2; break; case MULTIPLY: // this is * result = op1 * op2; break; case DIVIDE: // this is / result = op1 / op2; break; case MODULUS: // this is % result = op1 % op2; break; case NEGATIVE: // this is m int result = op1 * -op2; break; } return result; } private void evaluateOtherOperator(char commmand, int op1, int op2) { int reslut = 0; switch (operation) // This is second switch (operation) { case EXCHANGE: // this is r result = break case DUPLICATE: // this is d result break case PRINT: // this is p result break case PRINT_AND_REMOVE: // this is n break case PRINT_ALL: // this is f System.out.println(stack) break case CLEARall: // this is c stack.clear(); break case QUIT: // this is q result break case HElP: // this is ?, this show info for the Operations. System.out.println("\n --Program Info for Operations--"); System.out.println("The + add the top two items, the - subtract the top item from the next item."); System.out.println("The * multiply the top two items, the / integer divide the second item by the top item."); System.out.println("The % find the integer remainder when dividing the second item by the top item."); System.out.println("The m unary minus -- negate the top item, the r exchange the top two items"); System.out.println("The d duplicate top item on stack, the p print (to the screen) the top item"); System.out.println("The n will print and remove the top item, the f will print all the contents of the stack (leaving it intact) "); System.out.println("The c will clear the stack, the ? or h will print a help message"); System.out.println("And the q will make you quit the program."); break } }
How to get my second switch operations to work.
import java.util.Stack;
import java.util.Scanner;
public class PostfixEvaluator {
private final static char ADD = '+';
private final static char SUBTRACT = '-';
private final static char MULTIPLY = '*';
private final static char DIVIDE = '/';
private final static char MODULUS = '%';
private final static char NEGATIVE = 'm';
private final static char EXCHANGE = 'r';
private final static char DUPLICATE = 'd';
private final static char PRINT = 'p';
private final static char PRINT_AND_REMOVE = 'n';
private final static char PRINT_ALL = 'f';
private final static char CLEARall = 'c';
private final static char QUIT = 'q';
private final static char HElP = '?';
private Stack stack;
public PostfixEvaluator()
{
stack = new Stack();
}
public int evaluate(String expr)
{
int op1, op2, result = 0;
String token;
Scanner parser = new Scanner(expr);
while (parser.hasNext())
{
token = parser.next();
if (isOperator(token))
{
op2 = (stack.pop()).intValue();
op1 = (stack.pop()).intValue();
result = evaluateSingleOperator(token.charAt(0), op1, op2);
stack.push(new Integer(result));
}
if (isCommand(token));
evaluateother(token.charAt(0), op1, op2);
}
else
stack.push(new Integer(Integer.parseInt(token)));
}
return result;
}
///////////////////////////////////////////////////////////////////////////////////
private boolean isOperator(String token)
{
return (token.equals("+") || token.equals("-") ||token.equals("*") ||
token.equals("/") || token.equals("%") || token.equals("m"));
}
private boolean isCommand(String token)
{
return (token.equals("r") || token.equals("d") || token.equals("p") ||
token.equals("n") || token.equals("f") || token.equals("c") ||
token.equals("q") || token.equals("?"));
}
///////////////////////////////////////////////////////////////////////////////////
private int evaluateSingleOperator(char operation, int op1, int op2)
{
int result = 0;
switch (operation)
{
case ADD: // this is +
result = op1 + op2;
break;
case SUBTRACT: // this is -
result = op1 - op2;
break;
case MULTIPLY: // this is *
result = op1 * op2;
break;
case DIVIDE: // this is /
result = op1 / op2;
break;
case MODULUS: // this is %
result = op1 % op2;
break;
case NEGATIVE: // this is m
int result = op1 * -op2;
break;
}
return result;
}
private void evaluateOtherOperator(char commmand, int op1, int op2)
{
int reslut = 0;
switch (operation) // This is second switch (operation)
{
case EXCHANGE: // this is r
result =
break
case DUPLICATE: // this is d
result
break
case PRINT: // this is p
result
break
case PRINT_AND_REMOVE: // this is n
break
case PRINT_ALL: // this is f
System.out.println(stack)
break
case CLEARall: // this is c
stack.clear();
break
case QUIT: // this is q
result
break
case HElP: // this is ?, this show info for the Operations.
System.out.println("\n --
System.out.println("The + add the top two items, the - subtract the top item from the next item.");
System.out.println("The * multiply the top two items, the / integer divide the second item by the top item.");
System.out.println("The % find the integer remainder when dividing the second item by the top item.");
System.out.println("The m unary minus -- negate the top item, the r exchange the top two items");
System.out.println("The d duplicate top item on stack, the p print (to the screen) the top item");
System.out.println("The n will print and remove the top item, the f will print all the contents of the stack (leaving it intact) ");
System.out.println("The c will clear the stack, the ? or h will print a help message");
System.out.println("And the q will make you quit the program.");
break
}
}
}

Step by step
Solved in 2 steps

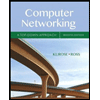
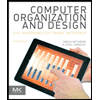
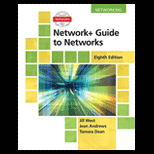
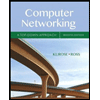
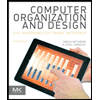
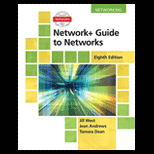
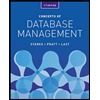
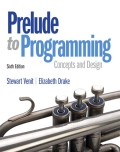
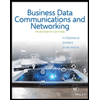