How do I compile these 3 codes together in netbeans. kindly check to make sure that these 3 codes will compile together sucessfully, to run a dice simulator. Main.java import java.util.Scanner; 2 3public class Main { 4 public static void main(String[] args) { 5 Scanner sc = new Scanner(System.in); 6 7 System.out.println("Welcome to the Dice Rolling Game!"); 8 int sides; 9 10 do { 11 System.out.print("Enter the number of sides for each die: "); 12 sides = sc.nextInt(); 13 if (sides <= 1) { 14 System.out.println("Please enter a valid number of sides for the dice."); 15 } 16 } while (sides <= 1); 17 18 PairDice dicePair = new PairDice(sides); 19 boolean playAgain = true; 20 int gamesWon = 0; 21 int gamesLost = 0; 22 23 while (playAgain) { 24 int result = dicePair.rollDice(); 25 26 System.out.println("Result: " + result); 27 28 if (dicePair.isSpecialCombination(result)) { 29 if (result == 2) { 30 System.out.println("1 + 1 = 2 snake eyes!"); 31 } else if (result == 7) { 32 System.out.println("7 craps!"); 33 } else if (result == 12) { 34 System.out.println("6 + 6 = 12 box cars!"); 35 } 36 playAgain = false; 37 gamesLost++; 38 System.out.println("You lost! Games won: " + gamesWon + ", Games lost: " + gamesLost); 39 } else if (result == 7 || result == 11) { 40 playAgain = false; 41 gamesWon++; 42 System.out.println("You won! Games won: " + gamesWon + ", Games lost: " + gamesLost); 43 } else { 44 System.out.println("Roll again!"); 45 } 46 } 47 sc.close(); 48 } 49} SingleDie.java 1class SingleDie { 2 private int sides; 3 4 public SingleDie(int sides) { 5 this.sides = sides; 6 } 7 8 public int roll() { 9 return (int) (Math.random() * sides) + 1; 10 } 11} PairDice.java 1class PairDice { 2 private SingleDie die1; 3 private SingleDie die2; 4 5 public PairDice(int sides) { 6 die1 = new SingleDie(sides); 7 die2 = new SingleDie(sides); 8 } 9 10 public int rollDice() { 11 int result1 = die1.roll(); 12 int result2 = die2.roll(); 13 return result1 + result2; 14 } 15 16 public boolean isSpecialCombination(int sum) { 17 return sum == 2 || sum == 7 || sum == 12; 18 } 19} 20
How do I compile these 3 codes together in netbeans. kindly check to make sure that these 3 codes will compile together sucessfully, to run a dice simulator. Main.java import java.util.Scanner; 2 3public class Main { 4 public static void main(String[] args) { 5 Scanner sc = new Scanner(System.in); 6 7 System.out.println("Welcome to the Dice Rolling Game!"); 8 int sides; 9 10 do { 11 System.out.print("Enter the number of sides for each die: "); 12 sides = sc.nextInt(); 13 if (sides <= 1) { 14 System.out.println("Please enter a valid number of sides for the dice."); 15 } 16 } while (sides <= 1); 17 18 PairDice dicePair = new PairDice(sides); 19 boolean playAgain = true; 20 int gamesWon = 0; 21 int gamesLost = 0; 22 23 while (playAgain) { 24 int result = dicePair.rollDice(); 25 26 System.out.println("Result: " + result); 27 28 if (dicePair.isSpecialCombination(result)) { 29 if (result == 2) { 30 System.out.println("1 + 1 = 2 snake eyes!"); 31 } else if (result == 7) { 32 System.out.println("7 craps!"); 33 } else if (result == 12) { 34 System.out.println("6 + 6 = 12 box cars!"); 35 } 36 playAgain = false; 37 gamesLost++; 38 System.out.println("You lost! Games won: " + gamesWon + ", Games lost: " + gamesLost); 39 } else if (result == 7 || result == 11) { 40 playAgain = false; 41 gamesWon++; 42 System.out.println("You won! Games won: " + gamesWon + ", Games lost: " + gamesLost); 43 } else { 44 System.out.println("Roll again!"); 45 } 46 } 47 sc.close(); 48 } 49} SingleDie.java 1class SingleDie { 2 private int sides; 3 4 public SingleDie(int sides) { 5 this.sides = sides; 6 } 7 8 public int roll() { 9 return (int) (Math.random() * sides) + 1; 10 } 11} PairDice.java 1class PairDice { 2 private SingleDie die1; 3 private SingleDie die2; 4 5 public PairDice(int sides) { 6 die1 = new SingleDie(sides); 7 die2 = new SingleDie(sides); 8 } 9 10 public int rollDice() { 11 int result1 = die1.roll(); 12 int result2 = die2.roll(); 13 return result1 + result2; 14 } 15 16 public boolean isSpecialCombination(int sum) { 17 return sum == 2 || sum == 7 || sum == 12; 18 } 19} 20
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How do I compile these 3 codes together in netbeans.
kindly check to make sure that these 3 codes will compile together sucessfully, to run a dice simulator.
Main.java
import java.util.Scanner; 2 3public class Main { 4 public static void main(String[] args) { 5 Scanner sc = new Scanner(System.in); 6 7 System.out.println("Welcome to the Dice Rolling Game!"); 8 int sides; 9 10 do { 11 System.out.print("Enter the number of sides for each die: "); 12 sides = sc.nextInt(); 13 if (sides <= 1) { 14 System.out.println("Please enter a valid number of sides for the dice."); 15 } 16 } while (sides <= 1); 17 18 PairDice dicePair = new PairDice(sides); 19 boolean playAgain = true; 20 int gamesWon = 0; 21 int gamesLost = 0; 22 23 while (playAgain) { 24 int result = dicePair.rollDice(); 25 26 System.out.println("Result: " + result); 27 28 if (dicePair.isSpecialCombination(result)) { 29 if (result == 2) { 30 System.out.println("1 + 1 = 2 snake eyes!"); 31 } else if (result == 7) { 32 System.out.println("7 craps!"); 33 } else if (result == 12) { 34 System.out.println("6 + 6 = 12 box cars!"); 35 } 36 playAgain = false; 37 gamesLost++; 38 System.out.println("You lost! Games won: " + gamesWon + ", Games lost: " + gamesLost); 39 } else if (result == 7 || result == 11) { 40 playAgain = false; 41 gamesWon++; 42 System.out.println("You won! Games won: " + gamesWon + ", Games lost: " + gamesLost); 43 } else { 44 System.out.println("Roll again!"); 45 } 46 } 47 sc.close(); 48 } 49}SingleDie.java
1class SingleDie { 2 private int sides; 3 4 public SingleDie(int sides) { 5 this.sides = sides; 6 } 7 8 public int roll() { 9 return (int) (Math.random() * sides) + 1; 10 } 11}PairDice.java
1class PairDice { 2 private SingleDie die1; 3 private SingleDie die2; 4 5 public PairDice(int sides) { 6 die1 = new SingleDie(sides); 7 die2 = new SingleDie(sides); 8 } 9 10 public int rollDice() { 11 int result1 = die1.roll(); 12 int result2 = die2.roll(); 13 return result1 + result2; 14 } 15 16 public boolean isSpecialCombination(int sum) { 17 return sum == 2 || sum == 7 || sum == 12; 18 } 19} 20Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 7 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
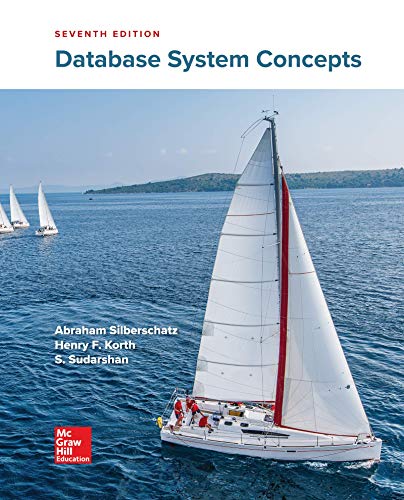
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
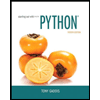
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
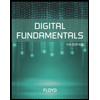
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
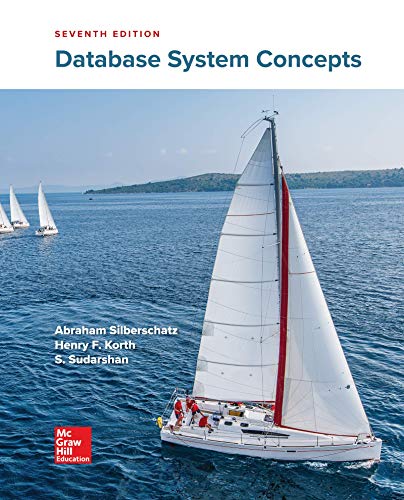
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
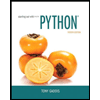
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
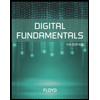
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
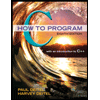
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
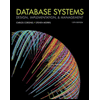
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
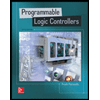
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education