Hello the question is the following: Write the definitions of the functions of the class primeFactorization (Example 11-4) declared in the primeFactorization header file. Write a program that uses this class to output the prime factorization of an integer. Your program should prompt the user for an integer, determine if the input is a prime number, and display the factorization of the integer. An example of the program is shown below: Enter an integer between 2 and 270,000,000,000,000: 1005427 1005427 is a prime number. Its factorization is: 1005427 = 1005427 I need help with write three things: integerManipulationImp.cpp, primeFactorizationImp.cpp, and a main.cpp. The attached images are the .h files respective to the cpp files that are needed to answer this question. Thank you.
Hello the question is the following:
Write the definitions of the functions of the class primeFactorization (Example 11-4) declared in the primeFactorization header file. Write a
An example of the program is shown below:
Enter an integer between 2 and 270,000,000,000,000: 1005427 1005427 is a prime number. Its factorization is: 1005427 = 1005427
I need help with write three things: integerManipulationImp.cpp, primeFactorizationImp.cpp, and a main.cpp.
The attached images are the .h files respective to the cpp files that are needed to answer this question.
Thank you.

![1 #ifndef primeFactorization_H
2 #define primeFactorization_H
3
4 #include "integerManipulation.h"
5
6 class primeFactorization: public integerManipulation
7 {
8 public:
9
10
11
56
12
13
14 primeFactorization (long long n = 0);
15
16
29
30
31
factorization();
//Function to output the prime factorization of num
//Postcondition: Prime factorization of num is printed;
32
33
34
35
36
void
17
18
19
20
21
22
23 private:
24
25
26
27
28
37 };
38
//Constructor with a default parameter.
//The instance variables of the base class are set according
//to the parameters and the array first125000Primes is
//created.
//Postcondition: num = n; revNum = 0; evenscount = 0;
// oddsCount = 0; zerosCount = 0;
//
first125000Primes
= first 125000 prime numbers.
long long first125000Primes [125000];
void primeFact(long long num, long long list [], int length,
int firstPrimeFact Index);
void first125000PrimeNum (long long list[], int length);
//Function to determine and store the first 125000 prime
//integers.
//Postcondition: The first 125000 prime numbers are
// determined and stored in the array first125000Primes;
void primeTest (long long num, long long list[], int length,
bool& primeNum, int& firstPrimeFact Index);
bool isPrime(long long number);
39 #endif
40](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F129b2cf9-3e0e-4398-9c26-940741b3a35e%2F864e0834-399f-4b15-be3d-9bd40e67fbf3%2Fre9y0zg_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

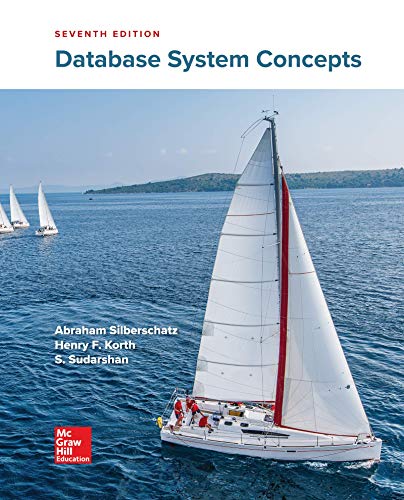
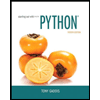
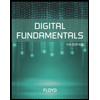
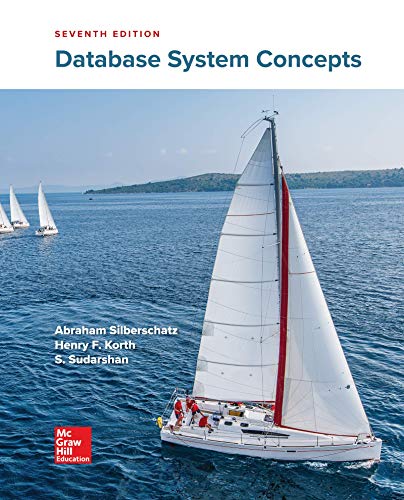
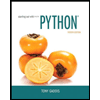
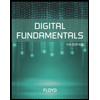
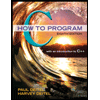
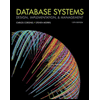
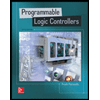