Given vector.h and vector.c from the Vector ADT section of the book, modify the code so that the vector holds characters instead of integers. Define also the following new functions in vector.c: 1. void vector_from_string(vector* v, char* string) - Create vector v then copy the characters from string to v. 2. void vector_print (vector* v) - Print vector v as a string; do not end with a newline The result will implement a string that is more flexible than the standard C char* strings. In particular insertion and deletion of characters are allowed anywhere in the strings. The main program performs various actions to test the new vector. Complete main() by replacing each TODO comment with code that implements the described actions. Note: Some operations in main() occur in pairs. After the program performs the first operation, the second operation is applied to the result of the first operation, rather than the state of the vector before performing the first operation. Ex: vector = friend
Given vector.h and vector.c from the Vector ADT section of the book, modify the code so that the vector holds characters instead of integers. Define also the following new functions in vector.c: 1. void vector_from_string(vector* v, char* string) - Create vector v then copy the characters from string to v. 2. void vector_print (vector* v) - Print vector v as a string; do not end with a newline The result will implement a string that is more flexible than the standard C char* strings. In particular insertion and deletion of characters are allowed anywhere in the strings. The main program performs various actions to test the new vector. Complete main() by replacing each TODO comment with code that implements the described actions. Note: Some operations in main() occur in pairs. After the program performs the first operation, the second operation is applied to the result of the first operation, rather than the state of the vector before performing the first operation. Ex: vector = friend
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section7.6: The Standard Template Library (stl)
Problem 8E
Related questions
Question
Given
![1 // TODO: Modify the code so that the vector holds characters instead of integers -- a flexible string
3 #ifndef VECTOR_H
4 #define VECTOR_H
6 #include "string.h"
unsigned int size;
8 // struct and typedef declaration for Vector ADT
9 typedef struct vector_struct {
10 int* elements;
11
12 vector;
13
Current file: vector.h
14 // interface for accessing Vector ADT
15
19 // Destroy vector
20 void vector_destroy (vector* v);
21
16 // Initialize vector
17 void vector_create(vector* v, unsigned int vectorSize);
18
22 // Resize the size of the vector
23 void vector_resize(vector* v, unsigned int vectorSize);
24
// for conversion
25 // Return pointer to element at specified index
26 int* vector_at (vector* v, unsigned int index);
27
28 // Insert new value at specified index
29 void vector_insert(vector* v, unsigned int index, int value);
30
31 // Insert new value at end of vector
32 void vector_push_back(vector* v, int value);
33
34 // Erase (remove) value at specified index
35 void vector_erase (vector* v, unsigned int index);
36
37 // Return number of elements within vector
38 int vector_size(vector* v);
39
40 // Convert an ordinary string to a vector
41 void vector_from_string(vector*, char* string);
42
43 // Print the vector as if it were a string
44 void vector_print (vector* v);
45
46 #endif
47
Current file: Vector.c
1 // TODO: Modify the code so that the vector holds characters instead of integers -- a flexible string
2
3 #include <stdio.h>
4 #include <stdlib.h>
5 #include "vector.h"
6
7 // Initialize vector with specified size
8 void vector_create(vector* v, unsigned int vectorSize) {
9
int i;
10
11
12
13
14
15
16
17
18 }
19
27 }
28
20 // Destroy vector
21 void vector_destroy(vector* v) {
22
if (v == NULL) return;
23
24
25
26
32
33
34
35
36
29 // Resize the size of the vector
30 void vector_resize(vector* v, unsigned int vectorSize) {
31
37
38
39
40
41
42 }
43
if (v== NULL) return;
v->elements = (int*) malloc(vectorSize * sizeof(int));;
v->size = vectorSize;
for (i = 0; i < v->size; ++i) {
v->elements [i] = 0;
}
47
48
49 }
50
free (v->elements);
v->elements = NULL;
v->size = 0;
int oldSize;
int i;
if (v== NULL) return;
oldSize = v->size;
v->elements = (int*)realloc(v->elements, vectorSize *sizeof(int));
v->size vectorSize;
for (i = oldSize; i < v->size; ++i) {
v->elements[i] = 0;
44 // Return pointer to element at specified index
45 int* vector_at (vector* v, unsigned int index) {
46
if (v== NULL || index >= v->size) return NULL;
return &(v->elements [index]);
}
50
51 // Insert new value at specified index
52 void vector_insert(vector* v, unsigned int index, int value) {
53 int i;
54
55
if (v== NULL || index > v->size) return;
56
57
vector_resize(v, v->size + 1);
58
for (i = v->size - 1; i > index; --i) {
59
v->elements[i] = v->elements [i-1];
60
61
v->elements [index] = value;
62}
63
64 // Insert new value at end of vector
65 void vector_push_back(vector* v, int value) {
vector_insert(v, v->size, value);
66
}
74
75
76
77
78
67 }
68
69 // Erase (remove) value at specified index
70 void vector_erase (vector* v, unsigned int index) {
71
int i;
72
73
if (v == NULL || index >= v->size) return;
for (i = index; i < v->size - 1; ++i) {
v->elements[i] = v->elements[i+1];
vector_resize(v, v->size - 1);
Current file: vector.c
}
79}
80
81 // Return number of elements within vector
82 int vector_size(vector* v) {
83
if (v== NULL) return -1;
return v->size;
84
85
86}
87
88 // Convert an ordinary string to a vector
89 void vector_from_string(vector* v, char* string) {
90
91
92
93
vector_create(v, strlen(string));
/* Type your code here. </
94}
95
96 // Print the elements of the vector (no final newline)
97 void vector_print (vector* v) {
98
99
/* Type your code here. */
100
101
102 }
103
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5779ee7a-d811-43a6-ba75-7d41285b789d%2Fcd734b81-caeb-487f-8373-167f0df8b72b%2F2xncxrb_processed.png&w=3840&q=75)
Transcribed Image Text:1 // TODO: Modify the code so that the vector holds characters instead of integers -- a flexible string
3 #ifndef VECTOR_H
4 #define VECTOR_H
6 #include "string.h"
unsigned int size;
8 // struct and typedef declaration for Vector ADT
9 typedef struct vector_struct {
10 int* elements;
11
12 vector;
13
Current file: vector.h
14 // interface for accessing Vector ADT
15
19 // Destroy vector
20 void vector_destroy (vector* v);
21
16 // Initialize vector
17 void vector_create(vector* v, unsigned int vectorSize);
18
22 // Resize the size of the vector
23 void vector_resize(vector* v, unsigned int vectorSize);
24
// for conversion
25 // Return pointer to element at specified index
26 int* vector_at (vector* v, unsigned int index);
27
28 // Insert new value at specified index
29 void vector_insert(vector* v, unsigned int index, int value);
30
31 // Insert new value at end of vector
32 void vector_push_back(vector* v, int value);
33
34 // Erase (remove) value at specified index
35 void vector_erase (vector* v, unsigned int index);
36
37 // Return number of elements within vector
38 int vector_size(vector* v);
39
40 // Convert an ordinary string to a vector
41 void vector_from_string(vector*, char* string);
42
43 // Print the vector as if it were a string
44 void vector_print (vector* v);
45
46 #endif
47
Current file: Vector.c
1 // TODO: Modify the code so that the vector holds characters instead of integers -- a flexible string
2
3 #include <stdio.h>
4 #include <stdlib.h>
5 #include "vector.h"
6
7 // Initialize vector with specified size
8 void vector_create(vector* v, unsigned int vectorSize) {
9
int i;
10
11
12
13
14
15
16
17
18 }
19
27 }
28
20 // Destroy vector
21 void vector_destroy(vector* v) {
22
if (v == NULL) return;
23
24
25
26
32
33
34
35
36
29 // Resize the size of the vector
30 void vector_resize(vector* v, unsigned int vectorSize) {
31
37
38
39
40
41
42 }
43
if (v== NULL) return;
v->elements = (int*) malloc(vectorSize * sizeof(int));;
v->size = vectorSize;
for (i = 0; i < v->size; ++i) {
v->elements [i] = 0;
}
47
48
49 }
50
free (v->elements);
v->elements = NULL;
v->size = 0;
int oldSize;
int i;
if (v== NULL) return;
oldSize = v->size;
v->elements = (int*)realloc(v->elements, vectorSize *sizeof(int));
v->size vectorSize;
for (i = oldSize; i < v->size; ++i) {
v->elements[i] = 0;
44 // Return pointer to element at specified index
45 int* vector_at (vector* v, unsigned int index) {
46
if (v== NULL || index >= v->size) return NULL;
return &(v->elements [index]);
}
50
51 // Insert new value at specified index
52 void vector_insert(vector* v, unsigned int index, int value) {
53 int i;
54
55
if (v== NULL || index > v->size) return;
56
57
vector_resize(v, v->size + 1);
58
for (i = v->size - 1; i > index; --i) {
59
v->elements[i] = v->elements [i-1];
60
61
v->elements [index] = value;
62}
63
64 // Insert new value at end of vector
65 void vector_push_back(vector* v, int value) {
vector_insert(v, v->size, value);
66
}
74
75
76
77
78
67 }
68
69 // Erase (remove) value at specified index
70 void vector_erase (vector* v, unsigned int index) {
71
int i;
72
73
if (v == NULL || index >= v->size) return;
for (i = index; i < v->size - 1; ++i) {
v->elements[i] = v->elements[i+1];
vector_resize(v, v->size - 1);
Current file: vector.c
}
79}
80
81 // Return number of elements within vector
82 int vector_size(vector* v) {
83
if (v== NULL) return -1;
return v->size;
84
85
86}
87
88 // Convert an ordinary string to a vector
89 void vector_from_string(vector* v, char* string) {
90
91
92
93
vector_create(v, strlen(string));
/* Type your code here. </
94}
95
96 // Print the elements of the vector (no final newline)
97 void vector_print (vector* v) {
98
99
/* Type your code here. */
100
101
102 }
103
}
![Given vector.h and vector.c from the Vector ADT section of the book, modify the code so that the vector holds characters instead of
integers. Define also the following new functions in vector.c
The result will implement a string that is more flexible than the standard C char* strings. In particular insertion and deletion of characters
are allowed anywhere in the strings.
The main program performs various actions to test the new vector. Complete main() by replacing each TODO comment with code that
implements the described actions.
Note: Some operations in main() occur in pairs. After the program performs the first operation, the second operation is applied to the result
of the first operation, rather than the state of the vector before performing the first operation.
Ex:
vector friend
Input indices for removal: a = 1 b = 2
friend removing character at index 1 --> fiend
fiend -- removing character at index 2 --> find
Ex: If the input is:
ridge
y
03
0 e 1 r
1 n 2 e
the output is:
ridge
ridgey
idgy
ergy
energy
1. void vector_from_string(vector* v, char* string) - Create vector v then copy the characters from string to v.
2. void vector_print (vector* v) - Print vector v as a string; do not end with a newline
1 #include <stdio.h>
2 #include "vector.h"
3
9
10
11
4 int main(void) {
5
int a;
6
int b;
7
8
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
43
44
45
46
47
48
49
50
51
--
52
53
54
55
56}
char char1;
char char2;
vector v;
char string[50];
// Read a string
scanf("%s\n", string);
// TODO: Create a vector from the input string
vector_print(&v);
printf("\n");
// Read a character char1
scanf("%c", &charl);
// TODDO: Add charl to the end of the string
vector_print(&v);
printf("\n");
// Read two indices a and b
scanf("%d %d", &a, &b);
40
41
42 vector_print(&v);
printf("\n");
Current file: main.c
// TODO: Remove the character at index a from the vector,
// then remove the character at index b of the modified vector
vector_print(&v);
printf("\n");
// Read two pairs of index and character
scanf("%d %c %d %c", &a, &charl, &b, &char2);
// TODO: Replace the character at index a with charl and
// the character at index b with char2
// Read two pairs of index and character
scanf("%d %c %d %c", &a, &charl, &b, &char2);
return 0;
// TODO: Insert character charl before index a of the vector, then
// insert character char2 before index b of the modified vector
vector_print(&v);
printf("\n");
// TODO: Free the memory allocated to the vectort
Load](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5779ee7a-d811-43a6-ba75-7d41285b789d%2Fcd734b81-caeb-487f-8373-167f0df8b72b%2Forl3jll_processed.png&w=3840&q=75)
Transcribed Image Text:Given vector.h and vector.c from the Vector ADT section of the book, modify the code so that the vector holds characters instead of
integers. Define also the following new functions in vector.c
The result will implement a string that is more flexible than the standard C char* strings. In particular insertion and deletion of characters
are allowed anywhere in the strings.
The main program performs various actions to test the new vector. Complete main() by replacing each TODO comment with code that
implements the described actions.
Note: Some operations in main() occur in pairs. After the program performs the first operation, the second operation is applied to the result
of the first operation, rather than the state of the vector before performing the first operation.
Ex:
vector friend
Input indices for removal: a = 1 b = 2
friend removing character at index 1 --> fiend
fiend -- removing character at index 2 --> find
Ex: If the input is:
ridge
y
03
0 e 1 r
1 n 2 e
the output is:
ridge
ridgey
idgy
ergy
energy
1. void vector_from_string(vector* v, char* string) - Create vector v then copy the characters from string to v.
2. void vector_print (vector* v) - Print vector v as a string; do not end with a newline
1 #include <stdio.h>
2 #include "vector.h"
3
9
10
11
4 int main(void) {
5
int a;
6
int b;
7
8
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
43
44
45
46
47
48
49
50
51
--
52
53
54
55
56}
char char1;
char char2;
vector v;
char string[50];
// Read a string
scanf("%s\n", string);
// TODO: Create a vector from the input string
vector_print(&v);
printf("\n");
// Read a character char1
scanf("%c", &charl);
// TODDO: Add charl to the end of the string
vector_print(&v);
printf("\n");
// Read two indices a and b
scanf("%d %d", &a, &b);
40
41
42 vector_print(&v);
printf("\n");
Current file: main.c
// TODO: Remove the character at index a from the vector,
// then remove the character at index b of the modified vector
vector_print(&v);
printf("\n");
// Read two pairs of index and character
scanf("%d %c %d %c", &a, &charl, &b, &char2);
// TODO: Replace the character at index a with charl and
// the character at index b with char2
// Read two pairs of index and character
scanf("%d %c %d %c", &a, &charl, &b, &char2);
return 0;
// TODO: Insert character charl before index a of the vector, then
// insert character char2 before index b of the modified vector
vector_print(&v);
printf("\n");
// TODO: Free the memory allocated to the vectort
Load
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
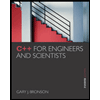
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
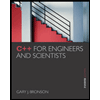
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr