Given the following Boxer class: (a) provide the code for a Comparator called BoxerComparator that will allow us to sort a list of boxers in ascending order, by weight. (b) Also provide a predicate as a lambda expression that will take the sorted list produced by your comparator, and will return a sublist of the heavyweight boxers (as indicated by their enum value HEAVY). Your solution should work with the code below. Fill in the code for your lambda expression in the commented section of the filter() method. A stub the BoxerComparator is included here; you may use that as your starting point for your Comparator. import java.util.ArrayList; import java.util.Comparator; import java.util.List; import java.util.stream.Collectors; public class Boxer { private String name; private double weight; private WEIGHT_CLASS weightClass; public enum WEIGHT_CLASS { FEATHER, MIDDLE, HEAVY }; public Boxer(String name, double weight) { this.name = name; this.weight = weight; if (weight < 135.0) { this.weightClass = WEIGHT_CLASS.FEATHER; } else if (weight < 180.0 ) { this.weightClass = WEIGHT_CLASS.MIDDLE; } else { this.weightClass = WEIGHT_CLASS.HEAVY; } } public String getName() { return this.name; } public double getWeight() { return this.weight; } public WEIGHT_CLASS getWeightClass() { return this.weightClass;} @Override public String toString() { return getName(); } // Smoke test to verify our boxers public static void main(String [] args) { List boxers = new ArrayList<>(); boxers.add(new Boxer("George Foreman", 283.3)); boxers.add(new Boxer("Sugar Ray Leonard", 155.2)); boxers.add(new Boxer("Muhammad Ali", 245.5)); boxers.add(new Boxer("Rocky Marciano", 210.0)); boxers.add(new Boxer("Manny Pacquiao", 134.2)); boxers.add(new Boxer("Floyd Mayweather", 134.6)); BoxerComparator byWeight = new BoxerComparator(); // *** YOU Write the BoxerComparator instantiated in the line above *** List sortedByWeight = boxers .stream() .sorted(byWeight) // YOUR Comparator is used here .collect(Collectors.toList()); System.out.println(sortedByWeight); List heavyweights = sortedByWeight .stream() .filter(/* YOU write the predicate as a lambda */) .collect(Collectors.toList()); System.out.println(heavyweights); } } /* Comparator stub is here. Normally placed in a separate file, but it's okay to include everything in the single essay answer for the exam */ import java.util.Comparator; public class BoxerComparator implements Comparator{ // YOU write the code for the BoxerComparator
Given the following Boxer class:
(a) provide the code for a Comparator called BoxerComparator that will allow us to sort a list of boxers in ascending order, by weight.
(b) Also provide a predicate as a lambda expression that will take the sorted list produced by your comparator, and will return a sublist of the heavyweight boxers (as indicated by their enum value HEAVY).
Your solution should work with the code below. Fill in the code for your lambda expression in the commented section of the filter() method. A stub the BoxerComparator is included here; you may use that as your starting point for your Comparator.
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.stream.Collectors;
public class Boxer {
private String name;
private double weight;
private WEIGHT_CLASS weightClass;
public enum WEIGHT_CLASS { FEATHER, MIDDLE, HEAVY };
public Boxer(String name, double weight) {
this.name = name;
this.weight = weight;
if (weight < 135.0) {
this.weightClass = WEIGHT_CLASS.FEATHER;
}
else if (weight < 180.0 ) {
this.weightClass = WEIGHT_CLASS.MIDDLE;
}
else {
this.weightClass = WEIGHT_CLASS.HEAVY;
}
}
public String getName() { return this.name; }
public double getWeight() { return this.weight; }
public WEIGHT_CLASS getWeightClass() { return this.weightClass;}
@Override
public String toString() { return getName(); }
// Smoke test to verify our boxers
public static void main(String [] args) {
List<Boxer> boxers = new ArrayList<>();
boxers.add(new Boxer("George Foreman", 283.3));
boxers.add(new Boxer("Sugar Ray Leonard", 155.2));
boxers.add(new Boxer("Muhammad Ali", 245.5));
boxers.add(new Boxer("Rocky Marciano", 210.0));
boxers.add(new Boxer("Manny Pacquiao", 134.2));
boxers.add(new Boxer("Floyd Mayweather", 134.6));
BoxerComparator byWeight = new BoxerComparator();
// *** YOU Write the BoxerComparator instantiated in the line above ***
List<Boxer> sortedByWeight = boxers
.stream()
.sorted(byWeight) // YOUR Comparator is used here
.collect(Collectors.toList());
System.out.println(sortedByWeight);
List<Boxer> heavyweights = sortedByWeight
.stream()
.filter(/* YOU write the predicate as a lambda */)
.collect(Collectors.toList());
System.out.println(heavyweights);
}
}
/* Comparator stub is here. Normally placed in a separate file, but
it's okay to include everything in the single essay answer for the exam */
import java.util.Comparator;
public class BoxerComparator implements Comparator<Boxer>{
// YOU write the code for the BoxerComparator
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

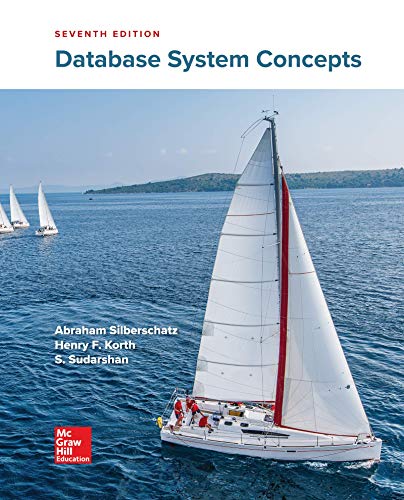
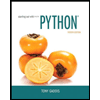
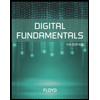
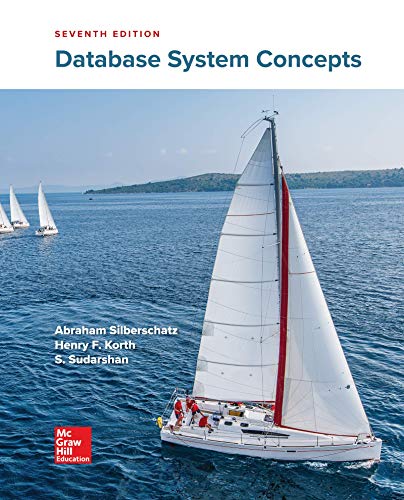
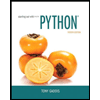
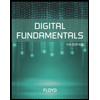
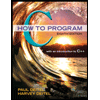
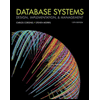
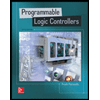