from collections import namedtuple #----------------------------------------------------------------------- class Person(): # Stores info about a single person # Created when an Individual (INDI) GEDCOM record is processed. #------------------------------------------------------------------- def __init__(self,ref): # Initializes a new Person object, storing the string (ref) by # which it can be referenced. self._id = ref self._asSpouse = [] # use a list to handle multiple families self._asChild = None def addName(self, names): # Extracts name parts from a list of names and stores them self._given = names[0] self._surname = names[1] self._suffix = names[2] def addIsSpouse(self, famRef): # Adds the string (famRef) indicating family in which this person # is a spouse, to list of any other such families self._asSpouse.append(famRef) def addIsChild(self, famRef): # Stores the string (famRef) indicating family in which this person # is a child self._asChild = famRef def printDescendants(self, prefix=''): # print info for this person and then call method in Family print(prefix + self.name()) # recursion stops when self is not a spouse for fam in self._asSpouse: families[fam].printFamily(self._id,prefix) def name (self): # returns a simple name string return self._given + ' ' + self._surname.upper()\ + ' ' + self._suffix def treeInfo (self): # returns a string representing the structure references included in self if self._asChild: # make sure value is not None childString = ' | asChild: ' + self._asChild else: childString = '' if self._asSpouse != []: # make sure _asSpouse list is not empty # Use join() to put commas between identifiers for multiple families # No comma appears if there is only one family on the list spouseString = ' | asSpouse: ' + ','.join(self._asSpouse) else: spouseString = '' return childString + spouseString def eventInfo(self): ## add code here to show information from events once they are recognized return '' def __str__(self): # Returns a string representing all info in a Person instance # When treeInfo is no longer needed for debugging it can return self.name() \ + self.eventInfo() \ + self.treeInfo() ## Comment out when not needed for debugging # Declare a named tuple type used by Family to create a list of spouses Spouse = namedtuple('Spouse',['personRef','tag']) class Family(): # Stores info about a family # An instance is created when an Family (FAM) GEDCOM record is processed. #------------------------------------------------------------------- def __init__(self, ref): # Initializes a new Family object, storing the string (ref) by # which it can be referenced. self._id = ref self._spouse1 = None self._spouse2 = None self._children = [] self._asSpouse = [] def addSpouse(self, personRef, tag): # Stores the string (personRef) indicating a spouse in this family newSpouse = Spouse(personRef, tag) if self._spouse1 == None: self._spouse1 = newSpouse else: self._spouse2 = newSpouse def addChild(self, personRef): # Adds the string (personRef) indicating a new child to the list self._children.append(personRef) def printFamily(self, firstSpouse, prefix): # Used by printDecendants in Person to print other spouse # and recursively invoke printDescendants on children # Manipulate prefix to prepare for adding a spouse and children if prefix != '': prefix = prefix[:-2]+' ' # Print out a '+' and the name of the second spouse, if present if self._spouse2: # check for the presence of a second spouse # If a second spouse is included, figure out which is the # "other" spouse relative to the descendant firstSpouse if self._spouse1.personRef == firstSpouse: secondSpouse = self._spouse2.personRef else: secondSpouse = self._spouse1.personRef print(prefix+ '+' + persons[secondSpouse].name()) # Make a recursive call for each child in this family for child in self._children: persons[child].printDescendants(prefix+'|--')
from collections import namedtuple
#-----------------------------------------------------------------------
class Person():
# Stores info about a single person
# Created when an Individual (INDI) GEDCOM record is processed.
#-------------------------------------------------------------------
def __init__(self,ref):
# Initializes a new Person object, storing the string (ref) by
# which it can be referenced.
self._id = ref
self._asSpouse = [] # use a list to handle multiple families
self._asChild = None
def addName(self, names):
# Extracts name parts from a list of names and stores them
self._given = names[0]
self._surname = names[1]
self._suffix = names[2]
def addIsSpouse(self, famRef):
# Adds the string (famRef) indicating family in which this person
# is a spouse, to list of any other such families
self._asSpouse.append(famRef)
def addIsChild(self, famRef):
# Stores the string (famRef) indicating family in which this person
# is a child
self._asChild = famRef
def printDescendants(self, prefix=''):
# print info for this person and then call method in Family
print(prefix + self.name())
# recursion stops when self is not a spouse
for fam in self._asSpouse:
families[fam].printFamily(self._id,prefix)
def name (self):
# returns a simple name string
return self._given + ' ' + self._surname.upper()\
+ ' ' + self._suffix
def treeInfo (self):
# returns a string representing the structure references included in self
if self._asChild: # make sure value is not None
childString = ' | asChild: ' + self._asChild
else: childString = ''
if self._asSpouse != []: # make sure _asSpouse list is not empty
# Use join() to put commas between identifiers for multiple families
# No comma appears if there is only one family on the list
spouseString = ' | asSpouse: ' + ','.join(self._asSpouse)
else: spouseString = ''
return childString + spouseString
def eventInfo(self):
## add code here to show information from events once they are recognized
return ''
def __str__(self):
# Returns a string representing all info in a Person instance
# When treeInfo is no longer needed for debugging it can
return self.name() \
+ self.eventInfo() \
+ self.treeInfo() ## Comment out when not needed for debugging
# Declare a named tuple type used by Family to create a list of spouses
Spouse = namedtuple('Spouse',['personRef','tag'])
class Family():
# Stores info about a family
# An instance is created when an Family (FAM) GEDCOM record is processed.
#-------------------------------------------------------------------
def __init__(self, ref):
# Initializes a new Family object, storing the string (ref) by
# which it can be referenced.
self._id = ref
self._spouse1 = None
self._spouse2 = None
self._children = []
self._asSpouse = []
def addSpouse(self, personRef, tag):
# Stores the string (personRef) indicating a spouse in this family
newSpouse = Spouse(personRef, tag)
if self._spouse1 == None:
self._spouse1 = newSpouse
else: self._spouse2 = newSpouse
def addChild(self, personRef):
# Adds the string (personRef) indicating a new child to the list
self._children.append(personRef)
def printFamily(self, firstSpouse, prefix):
# Used by printDecendants in Person to print other spouse
# and recursively invoke printDescendants on children
# Manipulate prefix to prepare for adding a spouse and children
if prefix != '': prefix = prefix[:-2]+' '
# Print out a '+' and the name of the second spouse, if present
if self._spouse2: # check for the presence of a second spouse
# If a second spouse is included, figure out which is the
# "other" spouse relative to the descendant firstSpouse
if self._spouse1.personRef == firstSpouse:
secondSpouse = self._spouse2.personRef
else: secondSpouse = self._spouse1.personRef
print(prefix+ '+' + persons[secondSpouse].name())
# Make a recursive call for each child in this family
for child in self._children:
persons[child].printDescendants(prefix+'|--')


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

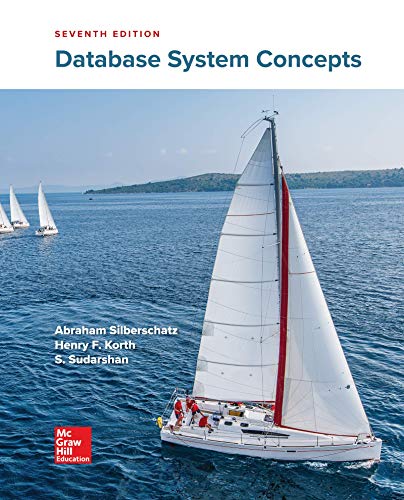
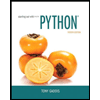
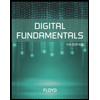
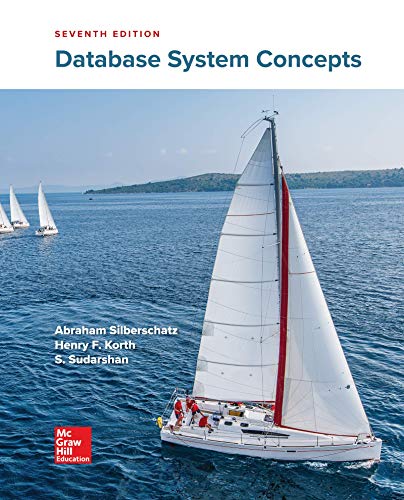
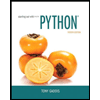
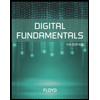
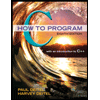
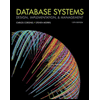
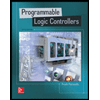