Exercise: I A company pays its employees on a weekly basis. The employees are of four types: Salaried employees are paid a fixed weekly salary regardless of the number of hours worked, hourly employees are paid by the hour and receive overtime pay (1.5* wage) for all hours worked in excess of 40 hours, commission employees are paid a percentage of their sales and base-commission employees receive a base salary plus a commission based on their gross sales. The company wants to implement a Java application that performs its payroll calculations polymorphically. (50 points) Hint: This programming exercise is similar to the AbstractClassExample posted in Blackboard under chapter 10. SalariedEmployee Employee Class Commission Employee BasePlusCommission Employee HourlyEmployee
Use NetBeans
Note for all the above User-Defined Classes:
• Provide appropriate validation code so the right values get populated in the instance variables. For example, the payrate should not be negative.
Write a Java application (Client) program with a static method called generateEmployees( ) that returns a random list of 10 different types of Employee objects. You would use an ArrayList to store the employee objects that will be returned. Use a for loop to populate randomly different types of employee objects with some random data. You could possibly think of a range of values like 1 – 4. If random value is 1, create a HourlyEmployee object with some randomly generated data, if 2, a SalariedEmployee object with some random data and so on. I would leave it to your ingenuity to generate and populate these different Employee objects with other data like name etc. As these objects are generated, add them to your data structure (array or ArrayList that you are using). Finally, the method returns this ArrayList In the same application class, implement the main( ) method. Call the generateEmployees( ) static method and using a for loop to print the details of each of the employee along with their earnings on the output window.



Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 2 images

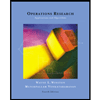
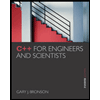
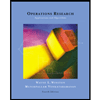
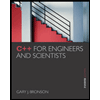