Decision Structures Do this for jGrasp This program will calculate the cost of having a framed picture for customers. After the customer enters the appropriate prompted values, the program will calculate the cost of the framing based on the specifications outlined below. The program should allow the user to order a second frame if desired. Define a java instance class as described below. Save the class definition in a file with an appropriate filename. Class Name: Frame Instance Variables: length – float (representing inches) (Fields) width – float (representing inches) type – char (valid values P, W, or M for Plastic, Wood, or Metal) matte – integer (representing the number of matting layers) Static Variable: count – tracks the number of frames created Instance Methods: A constructor that accepts no arguments and sets default values as follows: the length to 8, width to 10, type to W, and matte to 1 A constructor that accepts 4 arguments, and sets the fields appropriately. getArea( ) A method to calculate and return the area of the frame. getType( ) A method to return frame type. getMatte( ) A method to return the number of matting layers. toString() Displays the object’s attributes Create a program class, TestFrame, that contains a main() to test and use the above class. This process should contain methods as follows: main(): This method should: Call makeFrame() to get the inputs and create a Frame object. Call calcCost() to determine the cost of the picture. Display the cost of the picture along with the attribute values. Ask the user if he/she wants another picture. If the answer is No, display the total cost of the picture If the answer is Yes, implement steps 1 and 2 again (without a loop), then display: the number of frames created (through a variable) total cost of the picture(s), and average picture cost. The program should then end. Class methods: makeFrame( ) A function to prompt the user for inputs and accept the four (4) input values needed to create an instance of a Frame. It instantiates a Frame object and returns the object to the calling method. calcCost( ) This function has a Frame object as its formal argument and returns the cost of the picture to be determined as follows: The basic cost of a frame is $10 for P(lastic), $22 for W(ood), and $31 for M(etal). Any frame with an area greater than 100 square inches is considered large. Add $12.50 to the cost for a large frame. The basic frame comes with one (1) matte. There is an extra charge of $7.25 for each additional matte over one. A framed picture is charged for a maximum of five (5) matte layers total. Any picture with more than 5 mattes is charged for 5 matte layers. Additional Notes: Be sure to efficiently implement all 3 decision structures discussed in class Implement sound program design so as to avoid duplicated code An invalid frame type entry should default to Plastic Your program should accommodate upper and lower case user inputs Dollar values should be displayed to 2 decimal places Check your output!!
Decision Structures
Do this for jGrasp
This
- Define a java instance class as described below. Save the class definition in a file with an appropriate filename.
Class Name: Frame
Instance Variables: length – float (representing inches)
(Fields) width – float (representing inches)
type – char (valid values P, W, or M for Plastic, Wood, or Metal)
matte – integer (representing the number of matting layers)
Static Variable: count – tracks the number of frames created
Instance Methods: A constructor that accepts no arguments and sets default values as follows: the length to 8, width to 10, type to W, and matte to 1
A constructor that accepts 4 arguments, and sets the fields appropriately.
getArea( ) A method to calculate and return the area of the frame.
getType( ) A method to return frame type.
getMatte( ) A method to return the number of matting layers.
toString() Displays the object’s attributes
- Create a program class, TestFrame, that contains a main() to test and use the above class. This process should contain methods as follows:
main(): This method should:
- Call makeFrame() to get the inputs and create a Frame object.
- Call calcCost() to determine the cost of the picture.
- Display the cost of the picture along with the attribute values.
- Ask the user if he/she wants another picture.
- If the answer is No, display the total cost of the picture
- If the answer is Yes, implement steps 1 and 2 again (without a loop), then display:
- the number of frames created (through a variable)
- total cost of the picture(s), and
- average picture cost.
- The program should then end.
Class methods: makeFrame( ) A function to prompt the user for inputs and accept the four (4) input values needed to create an instance of a Frame. It instantiates a Frame object and returns the object to the calling method.
calcCost( ) This function has a Frame object as its formal argument and returns the cost of the picture to be determined as follows:
- The basic cost of a frame is $10 for P(lastic), $22 for W(ood), and $31 for M(etal).
- Any frame with an area greater than 100 square inches is considered large. Add $12.50 to the cost for a large frame.
- The basic frame comes with one (1) matte. There is an extra charge of $7.25 for each additional matte over one. A framed picture is charged for a maximum of five (5) matte layers total. Any picture with more than 5 mattes is charged for 5 matte layers.
Additional Notes:
- Be sure to efficiently implement all 3 decision structures discussed in class
- Implement sound program design so as to avoid duplicated code
- An invalid frame type entry should default to Plastic
- Your program should accommodate upper and lower case user inputs
- Dollar values should be displayed to 2 decimal places
- Check your output!!

Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 3 images

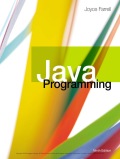
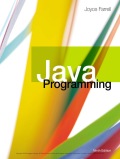