Debug the HTML program for a website that will show an output of text with a template for meeting minutes. The text should include all of the necessary markdown options such as Titles and Bullet Points for the template. After seeing the outputted text, you should be able to click the copy button below the text which will copy all of the text to your clipboard which can be pasted in a word document. Right now, the code will output the correct Meeting Minutes Template, but the copy button does not work. Please make it so the copy button works. Meeting Minutes Date: [Date of meeting] Time: [Time of meeting] Location: [Location of meeting] Attendees: [Name of attendee 1] [Name of attendee 2] Agenda: [Agenda item 1] [Agenda item 2] Discussion: [Discussion point 1] [Discussion point 2] Action Items: [Action item 1] [Action item 2] Copy
Debug the HTML program for a website that will show an output of text with a template for meeting minutes. The text should include all of the necessary markdown options such as Titles and Bullet Points for the template. After seeing the outputted text, you should be able to click the copy button below the text which will copy all of the text to your clipboard which can be pasted in a word document. Right now, the code will output the correct Meeting Minutes Template, but the copy button does not work. Please make it so the copy button works.
<style>
/* Add CSS styles for the template */
.template {
font-family: Arial, sans-serif;
font-size: 14px;
line-height: 1.5;
}
.template h1 {
font-size: 18px;
margin: 0 0 10px 0;
}
.template p {
margin: 0 0 10px 0;
}
.template ul {
list-style-type: disc;
margin: 0 0 10px 20px;
}
.template li {
margin-bottom: 5px;
}
/* Add styles for the "Copy" button */
.copy-button {
background-color: lightblue;
border: none;
color: white;
cursor: pointer;
font-size: 16px;
padding: 10px 20px;
}
.copy-button:hover {
background-color: blue;
}
</style>
<!-- HTML structure for the meeting minutes template -->
<div class="template">
<h1>Meeting Minutes</h1>
<p><strong>Date:</strong> [Date of meeting]</p>
<p><strong>Time:</strong> [Time of meeting]</p>
<p><strong>Location:</strong> [Location of meeting]</p>
<p><strong>Attendees:</strong></p>
<ul>
<li>[Name of attendee 1]</li>
<li>[Name of attendee 2]</li>
<!-- Add additional list items for each attendee -->
</ul>
<p><strong>Agenda:</strong></p>
<ul>
<li>[Agenda item 1]</li>
<li>[Agenda item 2]</li>
<!-- Add additional list items for each agenda item -->
</ul>
<p><strong>Discussion:</strong></p>
<ul>
<li>[Discussion point 1]</li>
<li>[Discussion point 2]</li>
<!-- Add additional list items for each discussion point -->
</ul>
<p><strong>Action Items:</strong></p>
<ul>
<li>[Action item 1]</li>
<li>[Action item 2]</li>
<!-- Add additional list items for each action item -->
</ul>
</div>
<!-- "Copy" button to copy the template to the clipboard -->
<button class="copy-button">Copy</button>
<script>
// Get a reference to the "Copy" button and the template element
const copyButton = document.querySelector('.copy-button');
const template = document.querySelector('.template');
// Add an event listener to the "Copy" button
copyButton.addEventListener('click', () => {
// Select the template element
template.select();
// Copy the selected element to the clipboard
document.execCommand('copy');
// Display a message to the user indicating that the template has been copied
alert('Template copied to clipboard!');
});
</script>

Step by step
Solved in 3 steps with 2 images

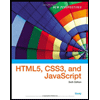
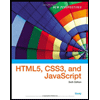