CSIS 112: Lab 7—Course RegistrationClasses are getting to be more realistic with each programming assignment. This assignment includes the valuable aspect of inheritance to facilitate reuse of code and operator overloading to allow the usage of familiar operators tailored specifically to the Person, Student, and Faculty classes. Composition is also demonstrated in the use of a Course class that contains objects of the Student and Faculty classes. The objective of this assignment is to demonstrate an ability to implement inheritance, composition, and overload operators in a program. Instructions: You are working in the IT department for a university and have been tasked with developing an application that will manage the registration process, which involves the creation of a course, the enrollment of students in the course, and the assignment of faculty members to teach the course. You will need to create several classes to maintain this information: Course, Person, Student, Faculty, and Date. The characteristics of Students and Faculty are shown below: Students: Faculty: ID (string) ID (string) First Name (string) First Name (string) Last Name (string) Last Name (string) Birthdate (Date) Birthdate (Date) Date enrolled (Date) Date hired (Date) Major (string) Title (string) Level (string) (i.e. Freshman, Sophomore...) Rank (string) GPA (double) Salary (double) Several of the data members above require the use of dates. Strings will not be acceptable substitutes for date fields. C++ does not have a built in data type for Date. Therefore, you may use this Date class in your program: #pragma once #include #include #include class Date{ friend std::ostream& operator<<(std::ostream&, Date); public: Date(int d=0, int m=0, int yyyy=0) { setDate(d, m, yyyy); } ~Date() {} void setDate(int d, int m, int yyyy){ day = d; month = m; year = yyyy; } private: int day; int month; int year; }; std::ostream& operator<<(std::ostream& output, Date d){ output << d.month << "/" << d.day << "/" << d.year; return output; } Person class (base class) The Person class should contain data members that are common to both Students and Faculty. Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor.
CSIS 112: Lab 7—Course RegistrationClasses are getting to be more realistic with each programming assignment. This assignment includes the valuable aspect of inheritance to facilitate reuse of code and operator overloading to allow the usage of familiar operators tailored specifically to the Person, Student, and Faculty classes. Composition is also demonstrated in the use of a Course class that contains objects of the Student and Faculty classes. The objective of this assignment is to demonstrate an ability to implement inheritance, composition, and overload operators in a program. Instructions: You are working in the IT department for a university and have been tasked with developing an application that will manage the registration process, which involves the creation of a course, the enrollment of students in the course, and the assignment of faculty members to teach the course. You will need to create several classes to maintain this information: Course, Person, Student, Faculty, and Date. The characteristics of Students and Faculty are shown below: Students: Faculty: ID (string) ID (string) First Name (string) First Name (string) Last Name (string) Last Name (string) Birthdate (Date) Birthdate (Date) Date enrolled (Date) Date hired (Date) Major (string) Title (string) Level (string) (i.e. Freshman, Sophomore...) Rank (string) GPA (double) Salary (double) Several of the data members above require the use of dates. Strings will not be acceptable substitutes for date fields. C++ does not have a built in data type for Date. Therefore, you may use this Date class in your program: #pragma once #include #include #include class Date{ friend std::ostream& operator<<(std::ostream&, Date); public: Date(int d=0, int m=0, int yyyy=0) { setDate(d, m, yyyy); } ~Date() {} void setDate(int d, int m, int yyyy){ day = d; month = m; year = yyyy; } private: int day; int month; int year; }; std::ostream& operator<<(std::ostream& output, Date d){ output << d.month << "/" << d.day << "/" << d.year; return output; } Person class (base class) The Person class should contain data members that are common to both Students and Faculty. Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
CSIS 112: Lab 7—Course
RegistrationClasses are getting to be more realistic with each programming assignment. This assignment includes
the valuable aspect of inheritance to facilitate reuse of code and operator overloading to allow the
usage of familiar operators tailored specifically to the Person, Student, and Faculty classes. Composition
is also demonstrated in the use of a Course class that contains objects of the Student and Faculty
classes.
The objective of this assignment is to demonstrate an ability to implement inheritance, composition, and overload
operators in a program.
Instructions:
You are working in the IT department for a university and have been tasked with developing an
application that will manage the registration process, which involves the creation of a course, the
enrollment of students in the course, and the assignment of faculty members to teach the course.
You will need to create several classes to maintain this information:
Course, Person, Student, Faculty, and Date.
The characteristics of Students and Faculty are shown below:
Students: Faculty:
ID (string) ID (string)
First Name (string) First Name (string)
Last Name (string) Last Name (string)
Birthdate (Date) Birthdate (Date)
Date enrolled (Date) Date hired (Date)
Major (string) Title (string)
Level (string) (i.e. Freshman, Sophomore...) Rank (string)
GPA (double) Salary (double)
RegistrationClasses are getting to be more realistic with each programming assignment. This assignment includes
the valuable aspect of inheritance to facilitate reuse of code and operator overloading to allow the
usage of familiar operators tailored specifically to the Person, Student, and Faculty classes. Composition
is also demonstrated in the use of a Course class that contains objects of the Student and Faculty
classes.
The objective of this assignment is to demonstrate an ability to implement inheritance, composition, and overload
operators in a program.
Instructions:
You are working in the IT department for a university and have been tasked with developing an
application that will manage the registration process, which involves the creation of a course, the
enrollment of students in the course, and the assignment of faculty members to teach the course.
You will need to create several classes to maintain this information:
Course, Person, Student, Faculty, and Date.
The characteristics of Students and Faculty are shown below:
Students: Faculty:
ID (string) ID (string)
First Name (string) First Name (string)
Last Name (string) Last Name (string)
Birthdate (Date) Birthdate (Date)
Date enrolled (Date) Date hired (Date)
Major (string) Title (string)
Level (string) (i.e. Freshman, Sophomore...) Rank (string)
GPA (double) Salary (double)
Several of the data members above require the use of dates. Strings will not be acceptable
substitutes for date fields.
C++ does not have a built in data type for Date. Therefore, you may use this Date class in your
program:
#pragma once
#include <iostream>
#include <cstdlib>
#include <cctype>
class Date{
friend std::ostream& operator<<(std::ostream&, Date);
public:
Date(int d=0, int m=0, int yyyy=0) {
setDate(d, m, yyyy);
}
~Date() {}
void setDate(int d, int m, int yyyy){
day = d;
month = m;
year = yyyy;
}
private:
int day;
int month;
int year;
};
std::ostream& operator<<(std::ostream& output, Date d){
output << d.month << "/" << d.day << "/" << d.year;
return output;
}
Person class (base class)
The Person class should contain data members that are common to both Students and Faculty.
Write appropriate member functions to store and retrieve information in the member variables above.
Be sure to include a constructor and destructor.
substitutes for date fields.
C++ does not have a built in data type for Date. Therefore, you may use this Date class in your
program:
#pragma once
#include <iostream>
#include <cstdlib>
#include <cctype>
class Date{
friend std::ostream& operator<<(std::ostream&, Date);
public:
Date(int d=0, int m=0, int yyyy=0) {
setDate(d, m, yyyy);
}
~Date() {}
void setDate(int d, int m, int yyyy){
day = d;
month = m;
year = yyyy;
}
private:
int day;
int month;
int year;
};
std::ostream& operator<<(std::ostream& output, Date d){
output << d.month << "/" << d.day << "/" << d.year;
return output;
}
Person class (base class)
The Person class should contain data members that are common to both Students and Faculty.
Write appropriate member functions to store and retrieve information in the member variables above.
Be sure to include a constructor and destructor.
Student class and Faculty class. (derived classes)
Both the Student and Faculty classes should be derived from the Person class. Each should have the
member variables shown above that are unique to each class. The data members that are common to
both classes should be inherited from the Person class.
In each derived class, the << operator should be overloaded to output, neatly formatted, all of the
information associated with a student or faculty member.
The < operator should be overloaded in the Student class to enable sorting of thevector of Student
objects that will exist in the Course class. You will use the sort function to sort this vector. The sort
function is part of the <algorithm> library.
Write member functions to store and retrieve information in the appropriate member variables. Be
sure to include a constructor and destructor in each derived class.
Design a Course class
The Course class has a name data member (i.e. a course’s name might be “CSIS 112”).
The class contains a Faculty object that represents the Faculty member assigned to teach the class.
The class contains a vector of Student objects that represent the students enrolled in the class.
The class has an integer variable, capacity, that represents the maximum number of students that can
be enrolled in the class.
The Course class should support operations to assign a faculty member to the course, enroll students in
the course, and list all of the course information, including its name, capacity, instructor, and students
enrolled in it.
Main()
You should write a main() function that begins by prompting the user for the name and capacity of a
course:
Both the Student and Faculty classes should be derived from the Person class. Each should have the
member variables shown above that are unique to each class. The data members that are common to
both classes should be inherited from the Person class.
In each derived class, the << operator should be overloaded to output, neatly formatted, all of the
information associated with a student or faculty member.
The < operator should be overloaded in the Student class to enable sorting of the
objects that will exist in the Course class. You will use the sort function to sort this vector. The sort
function is part of the <algorithm> library.
Write member functions to store and retrieve information in the appropriate member variables. Be
sure to include a constructor and destructor in each derived class.
Design a Course class
The Course class has a name data member (i.e. a course’s name might be “CSIS 112”).
The class contains a Faculty object that represents the Faculty member assigned to teach the class.
The class contains a vector of Student objects that represent the students enrolled in the class.
The class has an integer variable, capacity, that represents the maximum number of students that can
be enrolled in the class.
The Course class should support operations to assign a faculty member to the course, enroll students in
the course, and list all of the course information, including its name, capacity, instructor, and students
enrolled in it.
Main()
You should write a main() function that begins by prompting the user for the name and capacity of a
course:
“S” should allow the user to create a Student record and enroll the Student in the Course. Likewise, “I”
should allow the user to create an Instructor (aka Faculty) record and assign the Faculty member to the
course as the instructor. “L” should list ALL of the course information, including all of the information
about the Students assigned to the course, (sorted by ID number), as well as all of the information about
the faculty member assigned to teach the course. The user should be able to create and assign only
ONE faculty member to teach the course, but create and enroll as many Students to the course as the
capacity allows. The user should be able to list repeatedly until he or she selects “Q” to quit.
Below are some examples of the information that needs to be entered by the user:
Create and assign a faculty member as the instructor:
should allow the user to create an Instructor (aka Faculty) record and assign the Faculty member to the
course as the instructor. “L” should list ALL of the course information, including all of the information
about the Students assigned to the course, (sorted by ID number), as well as all of the information about
the faculty member assigned to teach the course. The user should be able to create and assign only
ONE faculty member to teach the course, but create and enroll as many Students to the course as the
capacity allows. The user should be able to list repeatedly until he or she selects “Q” to quit.
Below are some examples of the information that needs to be entered by the user:
Create and assign a faculty member as the instructor:

Transcribed Image Text:Result of listing Course Information before assigning any students to the course:
Information for Course CSIS 112
Instructor:
Faculty ID:
Faculty Name:
Birth Date:
Date Hired:
Salary:
12345
Dr. Melesa Poole, Associate Professor
1/20/1900
6/26/2009
1000000000.00
No students are enrolled in the course.
Create and assign a student to the course:
Create Student
ID: 11111
First Name: John
Last Name: Smith
Birthdate (mm/dd/yyyy): 05/05/1995
Major: Accounting
Level (Freshman, Sophomore, Junior, Senior): Junior
GPA: 3.95
Date of Enrollment: 12/12/2020

Transcribed Image Text:It should then present a menu to the user that looks like this:
Main Menu
I
Add Instructor
--
S
Add Student
--
L
List Course Information
--
e -- Quit
Selection:
"g" should allow the user to create a Student record and enroll the Student in the Course. Likewise, "I"
should allow the user to create an Instructor (aka Faculty) record and assign the Faculty member to the
course as the instructor. “L" should list ALL of the course information, including all of the information
about the Students assigned to the course, (sorted by ID number), as well as all of the information about
the faculty member assigned to teach the course. The user should be able to create and assign only
ONE faculty member to teach the course, but create and enroll as many Students to the course as the
capacity allows. The user should be able to list repeatedly until he or she selects "Q" to quit.
Below are some examples of the information that needs to be entered by the user:
Create and assign a faculty member as the instructor:
Create Faculty Member
ID: 12345
First Name: Melesa
Last Name: Poole
Birthdate (mm/dd/yyyy): 01/20/1900
Title:
Dr.
Rank: Associate Professor
Salary: 1000000000
Date of Hire: 06/26/2009
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Recommended textbooks for you
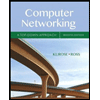
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
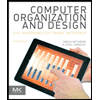
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
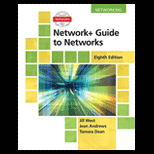
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
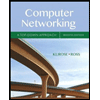
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
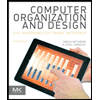
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
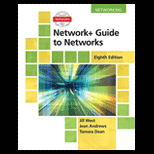
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
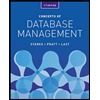
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
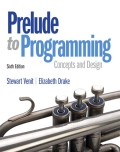
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
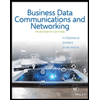
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY